Question
For this assignment you are to write a C++ program that reads a data file consisting of a players name followed by their batting average.
For this assignment you are to write a C++ program that reads a data file consisting of a players name followed by their batting average. The program will then print the highest batting average and the names of the people who have the highest batting average. It will also print the lowest batting average and the names of the people who have the lowest average. We will also assume that the file will not contain more than 100 players.
Input:
The program reads an input file consisting of each players name followed by their average. Here is the sample:
John .425 Terry .696 Glenn .704 Doug .636 Gary .400 Rusty .775 Mike .400 Orlando .775 Charles .606 J.D. .775 Gina .400 Sam .702 Rich .686 Nick .719 Mike .400 Rod .475 Tom .579 Hank .602 Steve .775 Ruben .702 Natalie .585 Jane .400 Larry .775 Joe .606 Don .422
Output:
The program outputs the highest and lowest batting averages and the names associated with these averages. For example, in the above sample data Glenn and Joe have the highest batting average and Gary has the lowest.
In order to keep track of all the people with the highest and lowest you will need to use a stack. Actually you will need to use two instances of the stack. One to hold the names of the highest and one to hold the names of the lowest averages. You should have created a stack in an earlier assignment.
Here is how the program might flow
When you read the first name and average at that time it is both the highest and lowest. You will store the average in variables that keep track of the highest and lowest average. You will then push the name on to both of the stacks. After reading the second name and average you are faced with some choices.
1. The new average is greater than the highest stored
a. Update the value of the highest average so far
b. Initialize the stack that holds the highest that is remove the names of the players from the stack
c. Save the name of the player having the highest average so far on the Stack
2. The new average is equal to the highest average so far. In this case simply add the name of the player to the top of the stack
3. The new average is smaller than the highest average so far. In this case you check to see if it is less than the lowest average found so far. If this is the case you need to do the following
a. Update the value of the lowest average so far
b. Initialize the stack that holds the lowest that is remove the names of the players from the stack
c. Save the names of the players having the lowest average so far on the stack
4. The average is equal to the lowest average so far. In this case add the name of the player to the top of the stack.
5. The average is not smaller than the smallest average so far and it is not larger than the largest so far. In this case simply discard the name and the average.
When the file has been completely read you should have a stack that contains the names of the players with the highest average and a stack with the names of the lowest average. You should also have a variable that contains the highest average and a variable that contains the lowest average. Print to the screen the highest average and the players with this average. Do the same with the lowest.
Please Note:
I do NOT want to see all of your code written in main. This is the end of an advanced programming course and you should write functions when appropriate. I want to see at least one class. For example, I might have a class named Payers with a function AddPlayer(string name, double batAvg);, a function like printLow(), printHigh() etc. You don't have to follow this example, just write good code that FULLY meets the requirements as stated. Of course, your stack class from Assignment 14 would be very useful as well.
Code for my class Stack: [code]
#ifndef STACK
#define STACK
#include
#define default_value 10
using std::vector;
template
class Stack : public vector
{
private:
int size;
T *values;
int index;
public:
Stack(int = default_value);
~Stack() { delete[] values; }
bool push(T);
T pop();
T peek();
bool isEmpty();
bool isFull();
};
template
Stack
size(x),
values(new T[size]),
index(-1)
{
}
template
bool Stack
{
if ((index + 1) == size)
return 1;
else
return 0;
}
template
bool Stack
{
bool b = 0;
if (!Stack
{
index += 1;
values[index] = x;
b = 1;
}
return b;
}
template
bool Stack
{
if (index == -1)
return 1;
else
return 0;
}
template
T Stack
{
T val = -1;
if (!Stack
{
val = values[index];
index -= 1;
}
else
{
cout << "Stack is Empty : ";
}
return val;
}
template
T Stack
{
return values[size - 1];
}
#endif
[code]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
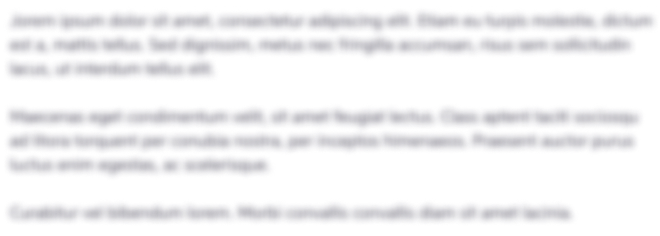
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started