Question
from datetime import datetime def CreateUsers(): print('##### Create users, passwords, and roles #####') with open('user.txt', 'a') as UserFile: while True: username = GetUserName() if username.upper()
from datetime import datetime
def CreateUsers(): print('##### Create users, passwords, and roles #####') with open('user.txt', 'a') as UserFile: while True: username = GetUserName() if username.upper() == "END": break userpwd = GetUserPassword() userrole = GetUserRole() UserDetail = username + "|" + userpwd + "|" + userrole + " " UserFile.write(UserDetail) UserFile.close()
def GetUserName(): username = input("Enter username or END to Quit: ") return username
def GetUserPassword(): userpwd = input("Enter pwd: ") return userpwd
def GetUserRole(): while True: userrole = input("Enter role (Admin or User): ") if userrole == "Admin" or userrole == "User": return userrole else: print('Please input a valid userrole (Admin or User).') UserFile = open("user.txt","r") while True: UserDetail = UserFile.readline() if not UserDetail: break UserDetail = UserDetail.replace(" ", "") UserList = UserDetail.split("|") username = UserList[0] userpassword = UserList[1] userrole = UserList[2] print("User Name: ", username, " Password: ", userpassword, " Role: ", userrole)
def Login(): with open('user.txt', 'r') as UserList: while True: UserDetail = UserList.readline() if not UserDetail: return "None", "None" UserDetail = UserDetail.replace(" ", "") UserList = UserDetail.split("|") UserName = input("Enter User Name: ") if UserName == UserList[0]: UserRole = UserList[2] return UserRole, UserName
def GetEmpName():
empname = input("Enter employee name: ")
return empname
def GetDatesWorked():
fromdate = input("Enter Start Date (mm/dd/yyyy: ")
todate = input("Enter End Date (mm/dd/yyyy")
def GetHoursWorked():
hours = float(input('Enter amount of hours worked: '))
return hours
def GetHourlyRate():
hourlyrate = float(input ("Enter hourly rate: "))
return hourlyrate
def GetTaxRate():
taxrate = float(input ("Enter tax rate: "))
return taxrate
def CalcTaxAndNetPay(hours, hourlyrate, taxrate):
grosspay = hours * hourlyrate
incometax = grosspay * taxrate
netpay = grosspay - incometax
return grosspay, incometax, netpay
def printinfo(DetailsPrinted):
TotEmployees = 0
TotHours = 0.00
TotGrossPay = 0.00
TotTax = 0.00
TotNetPay = 0.00
EmpFile = open("Employees.txt","r")
while True:
rundate = input ("Enter start date for report (MM/DD/YYYY) or All for all data in file: ")
if (rundate.upper() == "ALL"):
break
try:
rundate = datetime.strptime(rundate, "%m/%d/%Y")
break
except ValueError:
print("Invalid date format. Try again.")
print()
continue # skip next if statement and re-start loop
while True:
EmpDetail = EmpFile.readline()
if not EmpDetail:
break
EmpDetail = EmpDetail.replace(" ", "") #remove carriage return from end of line
EmpList = EmpDetail.split("|")
fromdate = EmpList[0]
if (str(rundate).upper() != "ALL"):
checkdate = datetime.strptime(fromdate, "%m/%d/%Y")
if (checkdate < rundate):
continue
todate = EmpList[1]
empname = EmpList[2]
hours = float(EmpList[3])
hourlyrate = float(EmpList[4])
taxrate = float(EmpList[5])
grosspay, incometax, netpay = CalcTaxAndNetPay(hours, hourlyrate, taxrate)
print(fromdate, todate, empname, f"{hours:,.2f}", f"{hourlyrate:,.2f}", f"{grosspay:,.2f}", f"{taxrate:,.1%}", f"{incometax:,.2f}", f"{netpay:,.2f}")
TotEmployees += 1
TotHours += hours
TotGrossPay += grosspay
TotTax += incometax
TotNetPay += netpay
EmpTotals["TotEmp"] = TotEmployees
EmpTotals["TotHrs"] = TotHours
EmpTotals["TotGrossPay"] = TotGrossPay
EmpTotals["TotTax"] = TotTax
EmpTotals["TotNetPay"] = TotNetPay
DetailsPrinted = True
if (DetailsPrinted): #skip of no detail lines printed
PrintTotals (EmpTotals)
else:
print("No detail information to print")
def PrintTotals(EmpTotals):
print()
print(f'Total Number Of Employees: {EmpTotals["TotEmp"]}')
print(f'Total Hours Worked: {EmpTotals["TotHrs"]:,.2f}')
print(f'Total Gross Pay: {EmpTotals["TotGrossPay"]:,.2f}')
print(f'Total Income Tax: {EmpTotals["TotTax"]:,.2f}')
print(f'Total Net Pay: {EmpTotals["TotNetPay"]:,.2f}')
if __name__ == "__main__":
##################################################
########## Write the line of code to call the method CreateUsers
def function_CreateUsers():
print()
print("##### Data Entry #####")
########## Write the line of code to assign UserRole and UserName to the function Login##
def Login(GetUserRole, UserName):
DetailsPrinted = False ###
EmpTotals = {} ###
########## Write the if statement that will check to see if UserRole is equal to NONE (NOTE: code will show red error lines until this line is written)####
if GetUserRole == 'NONE':
print(UserName," is invalid.")
else:
# only admin users can enter data
##### write the if statement that will check to see if the UserRole is equal to ADMIN (NOTE: code will show red error lines until this line is written)
if GetUserRole == 'ADMIN':
EmpFile = open("Employees.txt", "a+")
while True:
empname = GetEmpName()
if (empname.upper() == "END"):
break
fromdate, todate = GetDatesWorked()
hours = GetHoursWorked()
hourlyrate = GetHourlyRate()
taxrate = GetTaxRate()
EmpDetail = fromdate + "|" + todate + "|" + empname + "|" + str(hours) + "|" + str(hourlyrate) + "|" + str(taxrate) + " "
EmpFile.write(EmpDetail)
# close file to save data
EmpFile.close()
printinfo(DetailsPrinted)
Question:
The first part of my program is working fine (def Create Users). The second part of my program is not working. I can not enter the information for the employees (employee name, start/end dates, hours worked, hourly rate, tax rate, gross pay, net pay) Thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
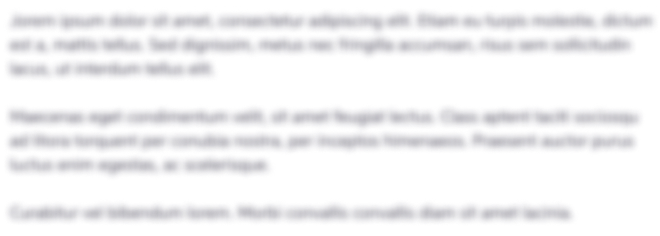
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started