Question
Fruit1 -- 50 pts Write a program called Fruit1 that reads a sequence of fruit purchases from file. Each purchase consists of the name of
- Fruit1 -- 50 pts
Write a program called "Fruit1" that reads a sequence of fruit purchases from file. Each purchase consists of the name of a fruit, the quantity purchased, and the unit cost. Store the purchases in an array list, e.g., std::vector< >, in one of three ways before you pretty-print the content to stdout as decribed below. Develop your program incrementally.
Vers 1 Create a struct called fruit that can the hold the relevant data, namely, the name of the fruit, the quantity purchased, and the unit cost. Read a data file from stdin using a while loop. Enter the data in a fruit struct which you store in the array list in the order read from file. Print the name, quantity, unit cost, product purchase cost, and cumulative total purchase cost to stdout.
Vers 2 Update the output formatting to appear like shown below. To make your life easier, the field widths used are: name (20), quantity (5), unit cost (4), product purchase cost (7), and total purchase cost (8). Be forewarned there may be an additional white space or two between the fields. All floating point numbers are printed to an accuracy of 2 decimal places.
Vers 3 Add commandline argument processing to allow selection of three mode options, namely, inorder, sortall, and sortone. See call pattern and associated output below. You must check that the correct number of arguments is given, and that the first argument is one the three mentioned (preceeded by a dash).
Vers 4 Add code needed to support option sortall which causes the data to be sorted in lexicographical order with respect to the fruit name. Similar named entries must appear in the same order as when read from file. To do this, overload operator< for struct fruit and apply std::stable_sort (more about this algorithm in class). Your pretty-print output code should work unchanged.
Vers 5 Add code needed to support option sortone which combines entries of the same named fruit into one by adding the quantities. When done, shrink the array list to contain just these entries. Your pretty-print output code should work unchanged.
- Fruit2 -- 70 pts
Write a program called "Fruit2" that reads a sequence of fruit purchases from file. Each purchase consists of the name of a fruit, the quantity purchased, and the unit cost. Store the purchases in a linked list, the implemention of which you write yourself. That is, DO NOT use std::list< >. Again, process data in one of three ways before you pretty-print the content to stdout as decribed above. Develop your program incrementally. Keep your list code as simple as possible. Do NOT place it in a class. Rather, write the code "in-place" where used (e.g., inserting node with new data, printing data from nodes, deleting nodes from list when deallocating memory at the end).
Vers 1 Copy the code from Fruit1.cpp to Fruit2.cpp while maintaing the skeleton code comments for the latter. Bring the code back to Vers 3. Declare a struct node as the basic building block for the linked list replacing the array list. Consider using a double-linked list but feel free to keep it single-linked if you prefer. Either way, you must use a dataless header node to refer to the list. Read data from file as before only this time incrementally build a linked list that stores it. Hint: To preserve the order of the data, new data must be inserted at the end of the list. Update the print loop to sweep thru the linked list nodes while printing the data to stdout using the same format as before. Add code to deallocate list memory when done.
Vers 2 Update the code to either insert new data in the order read from file OR sorted in lexicographical order with respect to the fruit name. You must add code for explictly making this happen. Do NOT rely on a sorting algorithm. Instead, find the correct place in the list for each new data entry as it gets inserted. Hint: Consider updating struct fruit to also overload operator<=. Your pretty-print output code should work unchanged. Same goes for the linked list cleanup code.
Vers 3 Update the code to either insert new data in the order read from file, sorted in lexicographical order with respect to the fruit name, OR doing the latter while consolidating similar name entries. You must once again add code for explictly making this happen. That is, find the correct place in the list for a data entry as it gets inserted. If the fruit has been purchased before, increment the quantity by the new amount. Otherwise, create and insert a new node. Hint: Consider updating struct fruit to also overload operator== and operator+= where the latter adds the quantity value associated with the new data to that of the existing old data. Your pretty-print output code should work unchanged. Same goes for the linked list cleanup code.
Fruit1.cpp
// list all headers needed #include <...> using namespace std;
struct fruit { overload operator < data declarations };
int main(int argc, char *argv[]) { prog -inorder|sortall|sortone file.txt set program mode from command line arg
declare array_list
open file while (reading more data) store data INORDER
if (mode == SORTALL || mode == SORTONE) apply std::stable_sort
if (mode == SORTONE) combine fruit quanties with same name
pretty-print array list content to stdout
free array list memory as necessary }
Fruit2.cpp
// list all headers needed #include <...> using namespace std;
struct fruit { overload operators <, <=, ==, and += data declarations };
struct node { constructor data declarations }
int main(int argc, char *argv[]) { prog -inorder|sortall|sortone file.txt set program mode from command line arg
declare linked_list
open file while (reading more data) store data INORDER | SORTALL | SORTONE
pretty-print linked list content to stdout
free linked list memory }
list
melon_cantaloupe 2.60 2.99 apples_gala 1.80 1.21 bananas 2.88 0.49 oranges_naval 2.63 0.99 apples_gala 3.00 1.21 raspberries 4.76 3.25 apples_gala 1.45 1.21 mango 4.07 1.20 blueberries 3.85 2.50 oranges_honeybell 4.20 1.08 apples_jazz 4.39 2.69 oranges_honeybell 4.22 1.08
Step by Step Solution
There are 3 Steps involved in it
Step: 1
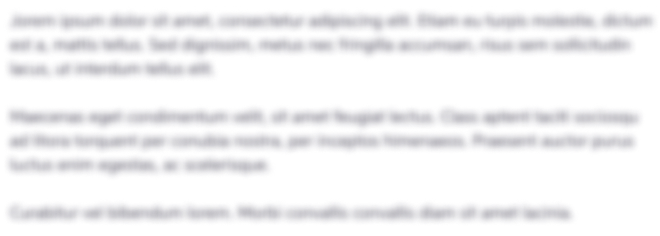
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started