Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Game Play ( handleClickAt , initializeGame, getCurrentBoardAsString, getCurrentTurn ) : * handleClickAt: * Maintain a 2 D grid to represent the game board ( e
Game Play handleClickAt initializeGame, getCurrentBoardAsString, getCurrentTurn:
handleClickAt:
Maintain a D grid to represent the game board eg boardROWSCOLS
Check if the clicked square is empty.
Update the board boardrowcol currentTurn
Alternate currentTurn between "RING" and "CROSS".
Return the appropriate TurnResult enum value GAMENOTOVER, RINGWON, CROSSWON, or DRAW
initializeGame:
Initialize the board grid with empty values eg
Set currentTurn to "RING" or randomly based on your preference
getCurrentBoardAsString:
Iterate through the board grid and construct a string representation using symbols for empty, ring, and cross egOX
getCurrentTurn:
Return the current turn's value RING or "CROSS"
Win Detection handleClickAt:
handleClickAt:
After updating the board and turn, check for wins in all directions horizontal vertical, diagonal from the last placed stone.
Use helper functions to check for lines of of the same symbol.
Update TurnResult to RINGWON or CROSSWON if a win is found.
Helper functions:
Define functions to check for lines of in a given direction eg checkLineHorizontal, checkLineVertical, checkLineDiagonal
These functions should iterate through the board, considering edge cases and preventing outofbounds access.
Computer Player handleClickAt initComputerPlayer:
initComputerPlayer:
Store the opponent's symbol RING or "CROSS"
Set a flag to enabledisable the computer player based on the argument NONE "COMPUTER"
handleClickAt:
If the computer player is enabled and it's their turn:
Choose a legal move empty square
This can be a simple strategy like:
Prioritize creating or blocking lines of
Choose corners or sides if no immediate threats or opportunities exist.
Randomly choose among remaining legal moves.
You can explore more advanced strategies using minimax, alphabeta pruning, or machine learning techniques.
public class Gomoku implements GomokuInterface
private static final int DEFAULTNUMROWS ;
private static final int DEFAULTNUMCOLS ;
private static final int SQUARESINLINEFORWIN ;
private Square board;
private Square currentTurn;
private boolean enableComputerPlayer;
private Square opponentSymbol;
private Gomoku game;
public GomokuString opponent
initComputerPlayeropponent;
public int getNumRows
return DEFAULTNUMROWS;
public int getNumCols
return DEFAULTNUMCOLS;
public int getNumInLineForWin
return SQUARESINLINEFORWIN;
public enum TurnResult
GAMENOTOVER,
RINGWON,
CROSSWON,
DRAW
@Override
public TurnResult handleClickAtint row, int col
if boardrowcol Square.EMPTY
return TurnResult.GAMENOTOVER;
boardrowcol currentTurn;
if checkWincurrentTurn
return currentTurn Square.RING TurnResult.RINGWON : TurnResult.CROSSWON;
if checkDraw
return TurnResult.DRAW;
currentTurn currentTurn Square.RING Square.CROSS : Square.RING;
return TurnResult.GAMENOTOVER;
private boolean diagonalRightint r int c
Square square boardrc;
int n ;
for int x c y r; x getNumRows && y getNumCols; x y
if squaretoString boardyxtoString
n;
else
break;
return n getNumInLineForWin;
private boolean diagonalLeftint r int c
Square square boardrc;
int n ;
for int x c y r; x getNumRows && y getNumCols; x y
if squaretoString boardyxtoString
n;
else
break;
return n getNumInLineForWin;
private boolean horizontalint r int c
Square square boardrc;
int n;
for int i c; i GomokuInterface.SQUARESINLINEFORWIN;
private boolean verticalint r int c
Square square boardrc;
int n ;
for int i r; i getNumRows;i
ifsquaretoString boardictoString
n;
else
break;
return n getNumInLineForWin;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
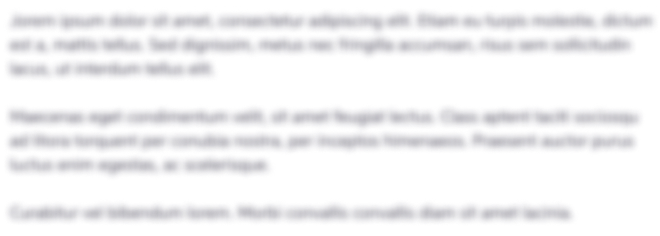
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started