Question
Getting error In member function float RND2::operator()() const: prog6.cc:52:67: error: expression cannot be used as a function ( rand ( ) % ( high
Getting error " In member function float RND2::operator()() const: prog6.cc:52:67: error: expression cannot be used as a function ( rand ( ) % ( high - low + 1 ) + low ) ) / low ( 10, prec ); " when tring to compile
Please help
main.cpp
#include
#include "binTree.h"
#define SEED 1 // seed for RNGs
#define N1 100 // size of 1st vector container #define LOW1 -999 // low val for rand integer #define HIGH1 999 // high val for rand integer
#define N2 50 // size of 2nd vector container #define LOW2 -99999 // low val for rand float #define HIGH2 99999 // high val for rand float #define PREC 2 // no of digits after dec pt
#define LSIZE 12 // no of vals printed on line #define ITEM_W 7 // no of spaces for each item
// prints single val template < class T > void print ( const T& );
// prints data vals in tree template < class T > void print_vals ( binTree < T >&, const string& );
// class to generate rand ints class RND1 { private: int low, high; public: RND1 ( const int& l = 0, const int& h = 1 ) : low ( l ), high ( h ) { } int operator ( ) ( ) const { return rand ( ) % ( high - low + 1 ) + low; } };
// class to generate rand floats class RND2 { private: int low, high, prec; public: RND2 ( const int& l = 0, const int& h = 1, const int& p = 0 ) : low ( l ), high ( h ), prec ( p ) { } float operator ( ) ( ) const { return ( static_cast < float > ( rand ( ) % ( high - low + 1 ) + low ) ) / pow ( 10, prec ); } };
// prints out val passed as argument template < class T > void print ( const T& x ) { static unsigned cnt = 0; cout << setw ( ITEM_W ) << x << ' '; cnt++; if ( cnt % LSIZE == 0 ) cout << endl; }
// prints out height of bin tree and data val in each node in inorder template < class T > void print_vals ( binTree < T >& tree, const string& name ) { cout << name << ": "; // print name of tree
// print height of tree cout << "height of tree = " << tree.height ( ) << endl << endl;
// print data values of tree in inorder cout << "Data values in '" << name << "' inorder: "; tree.inorder ( print ); cout << endl; }
// driver program: to test several member functions // of bin tree class
int main ( ) { srand ( SEED ); // set seed for RNGs
// create 1st vector and fill it with rand ints vector < int > A ( N1 ); generate ( A.begin ( ), A.end ( ), RND1 ( LOW1, HIGH1 ) );
// create bin tree with int vals in vector A, // and print all vals of tree
binTree < int > first; for (unsigned i = 0; i < A.size ( ); i++) first.insert ( A [ i ] ); print_vals ( first, "first" ); cout << endl;
// create 2nd vector and fill it with rand floats vector < float > B ( N2 ); generate ( B.begin ( ), B.end ( ), RND2 ( LOW2, HIGH2, PREC ) );
// create bin tree with float-pt vals in vector B, // and print all vals of tree
binTree < float > second; for ( unsigned i = 0; i < B.size ( ); i++ ) second.insert ( B [ i ] ); print_vals ( second, "second" ); cout << endl;
return 0; }
binTree.h
#ifndef BINTREE_H #define BINTREE_H
//#include "/home/cs340/progs/16f/p6/Node.h" #include "Node.h"
template
template
typedef enum { left_side, right_side } SIDE; SIDE rnd ( ) { return rand ( ) % 2 ? right_side : left_side; } //Used to decide random placement of Nodes, either left or right side //If the number is even, right side - else left side protected: Node
private: unsigned height( Node
/* *Public version* inorder() returns: nothing arguments: A pointer to a function that takes a class T element Purpose: Calls the private version of inorder() */ template
/* *Public version* insert() returns: nothing arugments: a reference to a constant class T, called element Purpose: calls the private version of insert() */ template
/* *Private version* height() const arguments: a pointer to a Node of class T, called ptr (holds the root) returns: the height of the tree purpose: finds the height of the tree, if there are no Nodes, returns a height of 0 */ template
return 1 + max(height(ptr->left), height(ptr->right)); //finds tree height }
/* *Private version* insert() returns: nothing arguments: A pointer to a reference Node of class T, called ptr (holds the root) A constant reference to a class T, called element (element to be inserted) purpose: Adds an item to the tree, If there is no Node, creates a new one, sets necessary pointers to NULL and sets Node data to the element. Decides where to make the new Node based on rnd() method call */ template
}
/* *Private version* inorder() returns: nothing arguments: a pointer to a Node of class T, called ptr (holds root). A pointer function that takes a class T argument Purpose: Goes through the tree using in order method, using recursion */ template
#endif
Node.h
#ifndef H_NODE #define H_NODE
// definition for class of nodes in bin tree
template < class T > class binTree; // forward declaration
template < class T > class Node { friend class binTree < T >; // binTree is friend public: // default constructor Node ( const T& x = T ( ), Node < T >* l = 0, Node < T >* r = 0 ) : data ( x ), left ( l ), right ( r ) { } private: T data; // data component Node < T > *left, *right; // left and right links }; #endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
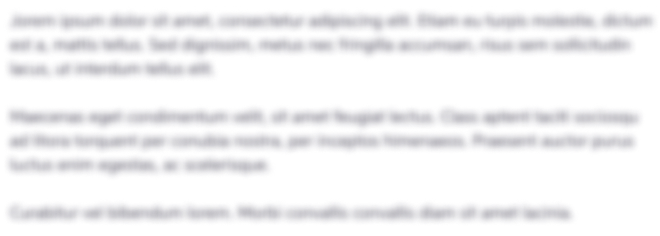
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started