Question
Given the two basesAandB, compute the transformation matrixMsuch thatMA=B. The code has already the two matrices initialised, and the first few steps for findingMare already
Given the two basesAandB, compute the transformation matrixMsuch thatMA=B.
The code has already the two matrices initialised, and the first few steps for findingMare already written (that is, the code initialises also [AT|BT]). You are requiredto write down the missing lines of code which compute the reduced REF of [AT|BT](this results in [I5|MT]). For this computation, you are allowed to use only therow addition and row swap operations already implemented.Please print out the content of the embedded matrix using the provided functionPrintEmbedded(M, N), and print the explanation of which rows were involved inyour operations similarly as in the example codePermutation.py.
Submit yournamended code as the solution.For the correct list of operations, i.e., computation ofM.(30 points)For showing the current content ofATandBTwith the help ofPrintEmbedded(M, N).(5 points)For printing the explanation of which operations are applied.(5 points)
# In this exercise you need to compute the transformation matrix M of the two bases A and B. # The only available operations for the reduced REF are row addition and row swapping
# ------------------------------------------- # Prints the content of matrix M # -------------------------------------------
def PrintMatrix(M): for i in range(0, 5): print('|{:>3d}{:>3d}{:>3d}{:>3d}{:>3d} |'.format(M[i][0],M[i][1],M[i][2],M[i][3],M[i][4]))
# ------------------------------------------- # Prints embedded matrices M and N # -------------------------------------------
def PrintEmbedded(M,N): for i in range(0, 5): print('|{:>2d}{:>3d}{:>3d}{:>3d}{:>3d} '.format(M[i][0],M[i][1],M[i][2],M[i][3],M[i][4]) +'{:>2d}{:>3d}{:>3d}{:>3d}{:>3d} |'.format(N[i][0],N[i][1],N[i][2],N[i][3],N[i][4]))
# ------------------------------------------- # Prints embedded product M x N = P # -------------------------------------------
def PrintProduct(M,N,P): for i in range(0, 5): if (i==2): print('|{:>2d}{:>3d}{:>3d}{:>3d}{:>3d} |'.format(M[i][0],M[i][1],M[i][2],M[i][3],M[i][4]) +' x |{:>2d}{:>3d}{:>3d}{:>3d}{:>3d} |'.format(N[i][0],N[i][1],N[i][2],N[i][3],N[i][4]) +' = |{:>2d}{:>3d}{:>3d}{:>3d}{:>3d} |'.format(P[i][0],P[i][1],P[i][2],P[i][3],P[i][4])) else: print('|{:>2d}{:>3d}{:>3d}{:>3d}{:>3d} |'.format(M[i][0],M[i][1],M[i][2],M[i][3],M[i][4]) +' |{:>2d}{:>3d}{:>3d}{:>3d}{:>3d} |'.format(N[i][0],N[i][1],N[i][2],N[i][3],N[i][4]) +' |{:>2d}{:>3d}{:>3d}{:>3d}{:>3d} |'.format(P[i][0],P[i][1],P[i][2],P[i][3],P[i][4]))
# -------------------------------------------------- # In Matrix M add row_j to row_i # --------------------------------------------------
def AddRows(i, j, M):
for k in range(0, 5): M[i][k] += M[j][k] # row_i = row_i + row_j
# ---------------------------------- # In Matrix M, swap row_i with row_j # ----------------------------------
def SwapRows(i, j, M):
tmp = M[i] M[i] = M[j] M[j] = tmp
# ---------------------------- # Returns a 5x5 Matrix of zeros # ---------------------------- def empty5x5Matrix(): return [[0 for i in range(0,5)] for i in range(0,5)] # ---------------------------------------------------------------------------------- # Returns the transpose of the square matrix M # ----------------------------------------------------------------------------------
def Transpose(M):
transpose = empty5x5Matrix() for i in range(0, 5): for j in range(0, 5): transpose[i][j] = M[j][i] return transpose
# ------------------------------------------- # Computes product N of square matrices M1, M2 # ------------------------------------------- def SqProd(M1,M2,N):
for i in range(0, 5): for j in range(0, 5): N[i][j]=0 for k in range (0, 5): N[i][j]+=(M1[i][k]*M2[k][j]) # -------------------------------------------
# Data initialisation
A=[ [-1, 1, 0, 0, 0], [ 0,-1, 1, 0, 0], [ 1, 0, 0, 0, 0], [ 0, 0,-1, 1, 0], [ 0, 0, 0,-1, 1]]
B=[[ 1,-1, 0, 0, 0], [ 0, 1,-1, 0, 0], [ 0, 0, 1,-1, 0], [ 0, 0, 0, 1,-1], [ 0, 0, 0, 0, 1]]
print ("The basis A ") PrintMatrix(A)
print (" The basis B ")
PrintMatrix(B)
print (" The task is to compute the transformation matrix M such that M x A = B ")
AT = Transpose(A) BT = Transpose(B)
print (" We adopt the embedded representation with matrix AT, the transpose of A, on the left and matrix BT, the transpose of B, on the right ") PrintEmbedded(AT,BT)
# ------------------------------------------- # DO NOT CHANGE ANYTHING ABOVE THIS LINE # -------------------------------------------
# # ADD YOUR CODE HERE # # Comment on the manipulated rows using print operation # apply functions AddRows and SwapRows to manipulate AT and BT accordingly and # apply function PrintEmbedded to print the current content of embedded AT and BT #
# ------------------------------------------- # DO NOT CHANGE ANYTHING BELOW THIS LINE # -------------------------------------------
# final test of correctness, by matrix multiplication of M and A we obtained B
ProductResult = empty5x5Matrix()
M = Transpose(BT)
SqProd(M, A, ProductResult)
print (" We compute the product of M and A obtaining B ")
PrintProduct(M, A, ProductResult)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
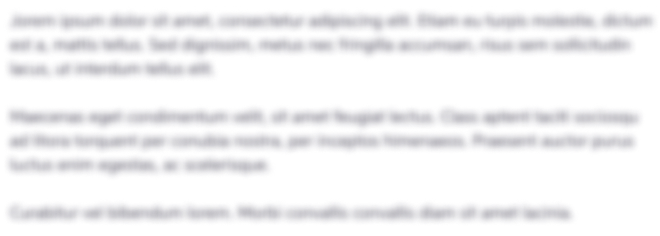
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started