Question
Goal: Your assignment is to write a C++ program to read in a list of appointment records from a file into an appointment database. Your
Goal: Your assignment is to write a C++ program to read in a list of appointment records from a file into an appointment database. Your program will then allow the user to enter a prefix for a name to search for in the database, your program will list out all appointments which have that prefix. The assignment introduces you to building a program using multiple files, implementing an ADT, C++ structs, and unit test programs.
Appointment Database Interface: You will use an ADT for the appointment database. In a software development team, developers will agree ahead of time on component interfaces (e.g. an ADT interface), so they can work in parallel. If someone doesnt follow the agreement, there will be build errors and an angry development manager. We too are going to agree on a common definition for the appointment database interface. You must use the definitions describe below exactly as specified.
The first part defines the Appointment ADT, which stores one appointment record:
// Stores information about one appointment
struct Appointment{
string firstName;
string lastName;
string start;
string end;
};
string GetStartTime(const Appointment&);
string GetEndTime(const Appointment&);
long long int GetTimeDifference(const Appointment&);
string GetFullName(const Appointment&);
int GetCharge(const Appointment&);
void OutputAppointment(const Appointment&, ostream&);
It should not take you long to implement the interface functions relating to Appointment, because you wrote the logic for all of them in Lab 1. The only difference is that you have to use the specified names for the functions, and the parameter is an Appointment data structure rather than a string parameter.
The second part defines the AppointmentDB ADT, which stores a list of appointment records:
const int MAX_APPOINTMENTS = 10;
struct AppointmentDB{
Appointment appointments[MAX_APPOINTMENTS];
size_t count;
};
void InitAppointmentDB(AppointmentDB&);
size_t LoadAppointmentDB(AppointmentDB&, istream&);
bool GetAppointment(const AppointmentDB&, size_t, Appointment&);
int FindAppointmentByNamePrefix(const AppointmentDB&, const string&, size_t = 0);
size_t OutputAppointmentDB(const AppointmentDB&, ostream&, bool = false);
You will need to implement these five functions:
1) InitAppointmentDB initializes the appointment database to have zero Appointment records. This is a one liner.
2) LoadAppointmentDB reads appointment records from an open ifstream (istream to be general) until there is no more data or an error occurs. This function uses a loop. On each iteration, it will read the four fields as strings, call the validate functions you wrote in Lab 1, and if the data passes validation and there is room left in the array,
fill in the first unused Appointment record with the data you read. This function will return the count of records that failed validation or which would not fit in the array because there was no room left. NOTE: the type of the second parameter is actually istream. Using this type instead of ifstream makes writing a unit test program easier.
3) GetAppointment checks whether the specified index is within range of the Appointment records currently stored in the appointments array (i.e., the array in the AppointmentDB struct). If it is not in range the function returns false. Otherwise, it will return true and set the Appointment reference parameter to the Appointment record at the specified index in the appointment array.
4) FindAppointmentByNamePrefix will scan the database of appointment records (const AppointmentDB&) looking for the first occurrence of a record that begins with the prefix (const string&). The function will start looking in the array of appointments starting at the start position. It returns the index of the first record it finds, or else -1 if it cannot find one.
5) OutputAppointmentDB will output all records in the AppointmentDB to the stream specified by the ostream& parameter again, use ostream and not ofstream so either cout or a file stream could be passed to the function. The bool parameter to this function chooses how the output will be printed: by default (false), output the four records in each Appointment directly, separated by a space; if the bool parameter is set to true, output using OutputAppointment instead.
Filenames you must use: Your main program will be built from 3 source files:
appointment-main.cpp -- main program appointment.h header file for appointment database ADT appointment.cpp implementation of appointment database ADT
Getting Started:
Developers often set some milestones where they do a partial implementation of their changes, and stabilize the code, and then continue onto the next milestone. It would help you if you schedule 3 milestones for this assignment.
Milestone 0: Create appointment.h and appointment.cpp. Copy in the definition of the ADT into appointment.h. Add stubs for the five interface functions in appointment.cpp. Download appointments-unit-test.cpp. Try compiling using the command g++ -std=c++11 appointments-unit-test.cpp appointment.cpp. Ensure the code builds with no errors before moving on.
Milestone 1: First implement GetStartTime, GetEndTime, GetTimeDifference, GetFullName, GetCharge, and OutputAppointment. You can adapt the code you wrote in Lab 1. Move the validation functions you wrote to appointment.cpp as well. These functions are not part of the ADT interface, but they are internal functions that your ADT implementation will use.
Next implement InitAppointmentDB, GetAppointment, and OutputAppointmentDB. The first can be done in one line, the other two take a bit more code but are still relatively short (each can be written in at most 10-15 lines or so). Now its time to write LoadAppointmentDB so you can read data into the appointment database. Finally implement FindAppointmentByNamePrefix.
Now try compiling and running the unit test program. If the program terminates with an assert, look for the line number of the assert. It should refer to a line in appointments-unit-test.cpp. Check what is wrong, and fix your code. When it finally passes the unit test, then your ADT implementation is probably right.
Milestone 2: Now its time to take your main program from Lab 1 and try to update it to run against your ADT. You will want to have a main program and an additional function to present the menu of options to the user and return the users choice (do input validation to ensure the user enters valid input valid meaning an integer, and in the correct range).
Heres an outline of what your main program should do:
1) Immediately begin a loop where you call the menu function to obtain the users choice (see below for list of choices),
2) If the user chooses 1. Load Appointments from File, prompt the user for the file name to read from. Load the contents of the file using LoadAppointmentDB. Print a status message indicating the database was loaded from a file, and how many records were successfully read. Print message if the input file couldnt be successfully opened, do not end the program in this case. 3) If the user chooses 2. Save Appointments to File, prompt the user for the file name to write to. Save the contents of the file using OutputAppointmentDB. Print a status message indicating the database was saved to a file, and how many records were successfully written. Print message if the output file couldnt be successfully opened, do not end the program in this case. 4) If the user chooses 3. List Appointments, print a header and then print the database to the screen (i.e., using cout). Make sure to output using the exact format in the examples below. Use the formatted output option for OutputAppointmentDB. Note the header is not part of OutputAppointmentDB. 5) If the user chooses 4. Find Appointments, ask the user for a prefix to search for in the appointments database. Use a loop to call FindAppointmentByNamePrefix to find all records that start with this prefix. 6) If the user chooses 5. Clear Appointment Database, call InitAppointmentDB to clear the database. Print a status message indicating the database was cleared (see below). 7) If the user chooses 6. Exit, the loop (and thus the program) ends.
The following screenshots illustrate the operation of the program:
Option 1 with Empty File:
Option 1 with data-01.txt:
Option 2 (after loading data-01.txt via Option 1):
Option 3 (after loading data-01.txt via Option 1):
Option 4 with prefix J (after loading data-01.txt via Option 1):
Option 5 (after loading data-01.txt via Option 1):
Program Style
Please refer to the Lab 1 assignment document for appropriate style.
In addition, there are some more style rules for working with ADTs:
1) You must not access the ADT in any other way than calling the interface functions defined in the .h file. 2) You must not change the ADT definition in any way. Adding new ADT interface functions is not allowed.
Finally, always pass a struct as a reference parameter to avoid needless copying of information onto the stack.
Submitting your code
As described earlier, your solution will consist of three files: appointment-main.cpp, appointment.h, and appointment.cpp. You must use these filenames.
Before submitting,
1) Create a new directory
2) Copy your three files to this new directory
3) Bring up a Command Prompt or Terminal.
4) Use cd to position yourself into this directory
5) Run the command g++ -std=c++11 appointment-main.cpp appointment.cpp
6) Make sure your file compiles with no warnings and errors, and links. An a.exe or a.out should be created.
7) Download the test files data-01.txt and data-02.txt. Run your test program on these two input files. Test all menu options on each file, ensure your program works correctly in all cases (compare to screenshots above for data-01.txt).
8) Download appointments-unit-test.cpp to the same directory.
9) Run the command g++ -std=c++11 appointment-unit-test.cpp appointment.cpp this replaces the executable you created previously.
10) Make sure your file compiles with no warnings and errors, and links. An a.exe or a.out should be created.
11) If the program terminates with an assert, look at code at the indicated line number in appointments-unit- test.cpp and figure out what doesnt match. If your output is wrong, then it should tell the character at which the expected output and your output differ, indicating what part of the text is different.
When you are able to pass all these tests, submit appointment.cpp, appointment.h, and appointment-main.cpp. Submit these three files and no other files.
Grading
Correctness is essential. Make sure your solution builds as specified above and correctly handles all the input files described earlier in this document. In addition, the unit test must pass all tests. Reminder: turning in code that does not compile will result in a grade of 0.
Even if your solution operates correctly, points will be taken off for:
Not following the design described above Not adhering to style guidelines described above Using techniques not presented in class Programming error not caught by other testing
Step by Step Solution
There are 3 Steps involved in it
Step: 1
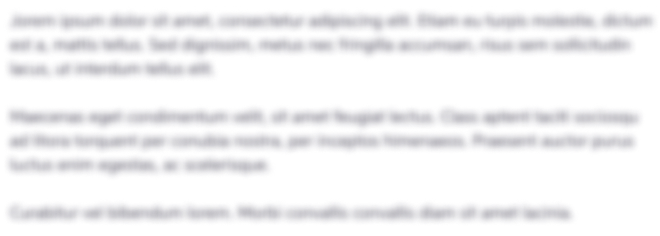
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started