Question
Greetings everyone, I need to make program in c language, here is a program that i made in the past but the instructions request 10
Greetings everyone,
I need to make program in c language, here is a program that i made in the past but the instructions request 10 prototype functions and 6 types of entries (2 arrays, 3 integers and 2 doubles) for the structure.
Futhermore, there should be two prototypes (int search(purse list[],int *count,int id) and int display(purse list[], int *count, int loc)) wont be included in the char menu function, these functions below willl be called in both void deleteEntry(purse list[], int *count) and void addEntry(purse list[], int *count).
//searches the list by id and returns the index, if there is a match or returns 100 if there is no match (the maximum size of the list is 50)
//three arguments: list, number of items, id
int search(purse list[],int *count,int id);
//displays the ids in the list, gets the id from the user and returns it
int display(purse list[], int *count, int loc);
here are the instructions:
Here is my code:
#define _CRT_SECURE_NO_DEPRECATE
#include
#include
#include
#define MAX_ENTRIES 40
//hardcodes six entries
typedef struct {
//1-int called id
int id;
//2-char array called name
char make[20];
//3-char array called brand
char model[20];
//4-int called stock
int stock;
//5-double called cost
double cost;
//6-double called price
double price;
} purse;
//runs the menu and gets the user choice
char menu();
//prints the contents of the list onto the screen
void initializeEntries(purse list[], int*count);
//prints a report to a file /*declare the file pointer, connect to the file and close the file pointer all inside this function*/
void createReport(purse list[], int *count);
//adds an item to the list
void addEntry(purse list[], int *count);
//displays the ids in the list, gets the id from the user and returns it
void display(purse list[], int *count);
//searches the list by id and returns the index, if there is a match or returns -1 //three arguments: list, number of items, id
void clearRecords(purse list[], int *count);
//removes an item from the list //takes three arguments, the list, the number of items and the location
void deleteEntry(purse list[], int *count);
//updates the sale price//takes three arguments, the list, the number of items and the location
void update(purse list[], int *count);
int main()
{
//Declare variables
purse list[MAX_ENTRIES];
char Choice;
int count = 6;
initializeEntries(list, &count);
//Greets the user
printf("Hello and welcome. This program helps you create an inventory for Exotic Car Dealership. ");
printf("To get you started, 6 cars have already been entered. ");
//while loop for selecting menu options
while (1)
{
Choice = menu();
if (Choice == 'A' || Choice == 'a') {
addEntry(list, &count);
}
else if (Choice == 'D' || Choice == 'd') {
deleteEntry(list, &count);
}
else if (Choice == 'P' || Choice == 'p') {
display(list, &count);
}
else if (Choice == 'S' || Choice == 's') {
createReport(list, &count);
}
else if (Choice == 'C' || Choice == 'c') {
clearRecords(list, &count);
}
else if (Choice == 'U' || Choice == 'u') {
update(list, &count);
}
else if (Choice == 'Q' || Choice == 'q') {
printf("Thank you and Goodbye. ");
break;
}
else printf("Enter a valid command ");
}
return 0;
}
//Function Prototype
//runs the menu and gets the user choice
char menu()
{
char Choice;
printf(" *************************************** ");
printf("Please select from the options below: ");
printf("P - Print entire inventory ");
printf("A - Add a new entry ");
printf("C - Clear all records ");
printf("S - Create a current report(save it to a file) ");
printf("D - Delete an item from the list(inventory) ");
printf("U - Update the price ");
printf("Q - Quit ");
//prompt the user
printf("What would you like to do: ");
scanf(" %c", &Choice);
return Choice;
}
//Function Prototype
//prints the contents of the list onto the screen
//returns the filled card
void initializeEntries(purse list[], int*count)
{
list[0].id = 101;
strcpy(list[0].make, "Rolls-Royce");
strcpy(list[0].model, "Ghost");
list[0].stock = 11;
list[0].cost = 120000.00;
list[0].price = 194900.00;
list[1].id = 102;
strcpy(list[1].model, "Mclaren");
strcpy(list[1].make, "P1");
list[1].stock = 5;
list[1].cost = 2000000.00;
list[1].price = 2399900.00;
list[2].id = 103;
strcpy(list[2].make, "Ferrari");
strcpy(list[2].model, "458 Speciale A");
list[2].stock = 7;
list[2].cost = 580000.00;
list[2].price = 649990.00;
list[3].id = 104;
strcpy(list[3].make, "Maserati");
strcpy(list[3].model, "GT Sport");
list[3].stock = 20;
list[3].cost = 100000.00;
list[3].price = 142200.00;
list[4].id = 105;
strcpy(list[4].make, "Lamborghini");
strcpy(list[4].model, "Huracan LP 610");
list[4].stock = 12;
list[4].cost = 220000.00;
list[4].price = 274900.00;
list[5].id = 106;
strcpy(list[5].make, "Tesla");
strcpy(list[5].model, "Model S");
list[5].stock = 30;
list[5].cost = 41700.00;
list[5].price = 62700.00;
*count = 6;
return;
}
//Prototype function that creates and saves a report in text file
//prints a report to a file
//*declare the file pointer, connect to the file and close the file pointer all inside this function*/
void createReport(purse list[], int *count)
{
//declares pointer
FILE *f;
int i;
//opens text file and add information into the text file
f = fopen("Records.txt", "w");
if (f == NULL)
{
printf("Error opening file! ");
exit(1);
}
if (*count == 0)
fprintf(f, "*****Inventory is empty***** ");
for (i = 0; i
{
//fprint to check
fprintf(f, "---Inventory Entry %d--- ", i + 1);
fprintf(f, "I.D.#: %d ", list[i].id);
fprintf(f, "MAKE: %s ", list[i].make);
fprintf(f, "MODEL: %s ", list[i].model);
fprintf(f, "QTY: %d ", list[i].stock);
fprintf(f, "COST: $%.2lf ", list[i].cost);
fprintf(f, "PRICE: $%.2lf ", list[i].price);
}
//closes text file
fclose(f);
return;
}
//Function Prototype
//adds an item to the list
//returns the new item
void addEntry(purse list[], int *count)
{
//prompt and get information
printf(" What is this car's I.D. number : ");
scanf("%d", &list[*count].id);
//prompt and get information
printf(" Please enter the Make of the car : ");
scanf(" %[^ ]s", list[*count].make);
//prompt and get information
printf(" Please enter the name of the car : ");
scanf(" %[^ ]s", list[*count].model);
//prompt and get information
printf(" What is this car's quantity : ");
scanf("%d", &list[*count].stock);
//prompt and get information
printf(" How much does this car cost from the manufacturer: ");
scanf("%lf", &list[*count].cost);
//prompt and get information
printf(" How much will you charge for this car: ");
scanf("%lf", &list[*count].price);
//prompt and get information
printf(" Your entry has been added to the catalog");
(*count)++;
return;
}
//displays the ids in the list, gets the id from the user and returns it
void display(purse list[], int *count)
{
//Declare variables
int i;
if (*count == 0)printf("*****Dealership's Inventory is empty***** ");
for (i = 0; i
{
//print to check
printf("---Inventory Entry %d--- ", i + 1);
printf("I.D.#: %d ", list[i].id);
printf("MAKE: %s ", list[i].make);
printf("MODEL: %s ", list[i].model);
printf("QTY: %d ", list[i].stock);
printf("COST: $%.2lf ", list[i].cost);
printf("PRICE: $%.2lf ", list[i].price);
}
return;
}
//Function Prototype
//removes an item from the list
//takes three arguments, the list, the number of items and the location
void deleteEntry(purse list[], int *count)
{
//Declare variables
int i, id;
printf("List of ID #'s: ");
for (i = 0; i
//print to check
printf("%d ", list[i].id);
printf(" Please enter the I.D.: ");
scanf("%d", &id);
for (i = 0; i
{
if (list[i].id == id)
{
list[i].id = list[*count - 1].id;
strcpy(list[i].make, list[*count - 1].make);
strcpy(list[i].model, list[*count - 1].model);
list[i].stock = list[*count - 1].stock;
list[i].cost = list[*count - 1].cost;
list[i].price = list[*count - 1].price;
(*count)--;
break;
}
}
return;
}
//prototype function clears records from program
void clearRecords(purse list[], int *count)
{
*count = 0;
//print the clearing of all entries
printf(" Your Inventory has been cleared of all entries.");
return;
}
//Prototype function updates information of a entry
//updates the sale price
//takes three arguments, the list, the number of items and the location
void update(purse list[], int *count)
{
//Declare variables
int i, id;
double price_up;
printf("List of ID #'s: ");
for (i = 0; i
printf("%d ", list[i].id);
//prompt and get information
printf(" Please enter the I.D.: ");
scanf("%d", &id);
//for loop
for (i = 0; i
{
if (list[i].id == id)
{
printf("---Inventory Entry %d--- ", i + 1);
printf("I.D.#: %d ", list[i].id);
printf("MAKE: %s ", list[i].make);
printf("MODEL: %s ", list[i].model);
printf("QTY: %d ", list[i].stock);
printf("COST: $%.2lf ", list[i].cost);
printf("PRICE: $%.2lf ", list[i].price);
printf("Please enter the updated price: ");
scanf("%lf", &price_up);
list[i].price = price_up;
break;
}
}
return;
}
Function descriptions: 1. //Greets the user 2. //hardcode eight (8) valid records 3. //runs the menu and gets the user choice 4. //prints the contents of the list onto the screen 5. //adds an item to the list 6. //prints a report to a file /*declare the file pointer, connect to the file called "report.txt" and close the file pointer all inside this function 7. //displays the ids in the list, gets the id from the user and returns it 8. I/searches the list by id and returns the index, if there is a match or returns 180 //three arguments: list, number of items, id if there is no match (the maximum size of the list is 50) 9. //removes an item from the list //takes three arguments, the list, the number of items and the location that was //returned by the search function 10.//updates an item in the list //takes two arguments, the list and the location that was //returned by the search function Function descriptions: 1. //Greets the user 2. //hardcode eight (8) valid records 3. //runs the menu and gets the user choice 4. //prints the contents of the list onto the screen 5. //adds an item to the list 6. //prints a report to a file /*declare the file pointer, connect to the file called "report.txt" and close the file pointer all inside this function 7. //displays the ids in the list, gets the id from the user and returns it 8. I/searches the list by id and returns the index, if there is a match or returns 180 //three arguments: list, number of items, id if there is no match (the maximum size of the list is 50) 9. //removes an item from the list //takes three arguments, the list, the number of items and the location that was //returned by the search function 10.//updates an item in the list //takes two arguments, the list and the location that was //returned by the search functionStep by Step Solution
There are 3 Steps involved in it
Step: 1
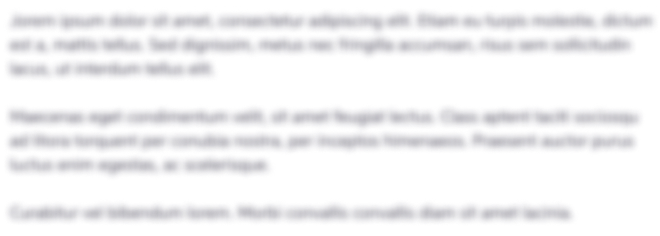
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started