Question
GV DIE CLASS: import java.util.*; import java.awt.*; import java.awt.event.*; import javax.swing.*; import javax.swing.border.*; public class GVdie extends JPanel implements MouseListener, Comparable{ private int myValue, displayValue;
GV DIE CLASS:
import java.util.*; import java.awt.*; import java.awt.event.*; import javax.swing.*; import javax.swing.border.*;
public class GVdie extends JPanel implements MouseListener, Comparable{
private int myValue, displayValue;
private boolean selected, scored;
private int mySize;
private int dotSize;
private int left;
private int right;
private int middle;
private Color SELECTED_COLOR = Color.pink;
private Color SCORED_COLOR = Color.gray;
private Color BACKGROUND = Color.white;
private int NUM_ROLLS;
private javax.swing.Timer myTimer;
public GVdie(int size) { mySize = size; selected = false; dotSize = mySize / 5; int spacer = (mySize - (3*dotSize))/4; left = spacer; right = mySize - spacer - dotSize; middle = (mySize - dotSize) /2; setBackground(BACKGROUND); setForeground(Color.black); setSize(size,size); setPreferredSize(new Dimension(size, size)); setMinimumSize(new Dimension(size, size)); setMaximumSize(new Dimension(size, size));
Border raised = BorderFactory.createRaisedBevelBorder(); Border lowered = BorderFactory.createLoweredBevelBorder(); Border compound = BorderFactory.createCompoundBorder(raised, lowered); setBorder(compound);
displayValue = myValue = (int) (Math.random()*6)+1; setNumRolls(6); myTimer = new javax.swing.Timer(250, new Animator()); addMouseListener(this); }
public GVdie() { this(100); }
public boolean isSelected(){ return selected; }
public boolean isScored(){ return scored; }
public void setBlank(){ displayValue = 0; myValue = 0; scored = false; selected = false; repaint(); }
public void setScored(boolean h){ scored = h; if(scored){ selected = false; setBackground(SCORED_COLOR); }else{ setBackground(BACKGROUND); } repaint(); }
public void setSelected(boolean h){ selected = h; if(selected){ scored = false; setBackground(SELECTED_COLOR); }else{ setBackground(BACKGROUND); } repaint(); }
public void setForeground(Color c){ super.setForeground(c); }
public void roll (){ myValue = (int) (Math.random()*6)+1;
// start the animated roll myTimer.restart();
}
public void setDelay (int msec){ if (msec > 0) myTimer = new javax.swing.Timer(msec, new Animator()); }
public void setNumRolls (int num){ NUM_ROLLS = 0; if (num > 0) NUM_ROLLS = num; }
public int getValue(){ return myValue; }
public void paintComponent(Graphics g){ super.paintComponent(g);
switch (displayValue){ case 1: g.fillOval (middle,middle,dotSize,dotSize); break; case 2: g.fillOval (left,left,dotSize,dotSize); g.fillOval (right,right,dotSize,dotSize); break; case 3: g.fillOval (middle,left,dotSize,dotSize); g.fillOval (middle,middle,dotSize,dotSize); g.fillOval (middle,right,dotSize,dotSize); break; case 5: g.fillOval (middle,middle,dotSize,dotSize); // fall throught and paint four more dots case 4: g.fillOval (left,left,dotSize,dotSize); g.fillOval (left,right,dotSize,dotSize); g.fillOval (right,left,dotSize,dotSize); g.fillOval (right,right,dotSize,dotSize); break; case 6: g.fillOval (left,left,dotSize,dotSize); g.fillOval (left,middle,dotSize,dotSize); g.fillOval (left,right,dotSize,dotSize); g.fillOval (right,left,dotSize,dotSize); g.fillOval (right,middle,dotSize,dotSize); g.fillOval (right,right,dotSize,dotSize); break; }
}
public void mouseClicked(MouseEvent e){ // do not allow scored die to be selected if(scored){ return; } if(selected){ selected = false; setBackground(BACKGROUND); }else{ selected = true; setBackground(SELECTED_COLOR); } repaint();
}
public void mousePressed(MouseEvent e){}
public void mouseReleased(MouseEvent e){}
public void mouseExited(MouseEvent e){}
public void mouseEntered(MouseEvent e){}
public int compareTo(Object o){ GVdie d = (GVdie) o; return getValue() - d.getValue(); }
private class Animator implements ActionListener{ int count = 0; public void actionPerformed(ActionEvent e){ displayValue = (int) (Math.random()*6)+1; repaint(); count++; // Should we stop rolling? if (count == NUM_ROLLS){ count=0; myTimer.stop(); displayValue = myValue; repaint(); } } }
}
Project 3 Farkle GUI Model-View Design Pattern A common design strategy is to divide applications into at least two parts: the user interface (View) and a back end that takes care of the data (Model). For this project, we provide a completed Model class that simulates a dice game, called Farkle, that you will eventually implement yourself in Project 4. For now, you do not need to know any of the game rules. The Model-View pattern enforces clear responsibilities between the model and view. For example, the GUI (view) should not be responsible for maintaining any data or knowing how the data are processed. The sole responsibility of the GUI is to support user interaction with the Model. GUI Features The game supports multiple players using six dice . a player rolls all six dice to start and then selects one or more dice. .a player then chooses to roll again or pass the dice. Only unselected dice are rolled. . the next player is chosen by clicking a radio button . players can display the best score by selecting File -> Best Score . players can start a new game at any time by selecting File -> New Game . players can quit at any time by selecting the File ->Quit Step 1: Create a New Bluel Project Step 2: Use Existing ydie Rather than writing your own Die class, we are providing a completed class for you. Create a new class in Blue! called yddeand delete all of the provided code. Copy and paste the provided code from (GVdie.java) into the newly created class. It should compile with no errors. Do not make any changes to this code but you are encouraged to read through the source code to see how it works. Project 3 Farkle GUI Model-View Design Pattern A common design strategy is to divide applications into at least two parts: the user interface (View) and a back end that takes care of the data (Model). For this project, we provide a completed Model class that simulates a dice game, called Farkle, that you will eventually implement yourself in Project 4. For now, you do not need to know any of the game rules. The Model-View pattern enforces clear responsibilities between the model and view. For example, the GUI (view) should not be responsible for maintaining any data or knowing how the data are processed. The sole responsibility of the GUI is to support user interaction with the Model. GUI Features The game supports multiple players using six dice . a player rolls all six dice to start and then selects one or more dice. .a player then chooses to roll again or pass the dice. Only unselected dice are rolled. . the next player is chosen by clicking a radio button . players can display the best score by selecting File -> Best Score . players can start a new game at any time by selecting File -> New Game . players can quit at any time by selecting the File ->Quit Step 1: Create a New Bluel Project Step 2: Use Existing ydie Rather than writing your own Die class, we are providing a completed class for you. Create a new class in Blue! called yddeand delete all of the provided code. Copy and paste the provided code from (GVdie.java) into the newly created class. It should compile with no errors. Do not make any changes to this code but you are encouraged to read through the source code to see how it worksStep by Step Solution
There are 3 Steps involved in it
Step: 1
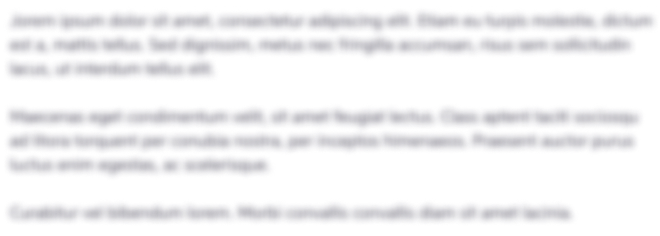
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started