Question
Hello can you help me get this Program up and Running? I have four different classes. Also, how would I incorporate a player's ability to
Hello can you help me get this Program up and Running? I have four different classes. Also, how would I incorporate a player's ability to place a bet...let's say $200? Please bold any changes. Thank you. :)
import java.util.Scanner;
public class BlackJackGame {
public static void main (String args [])
{
Scanner input = new Scanner(System.in);
Deck theDeck = new Deck (1, true);
Player me = new Player("Suz");
Player dealer = new Player("Dealer");
me.addCard(theDeck.dealNextCard());
dealer.addCard(theDeck.dealNextCard());
me.addCard(theDeck.dealNextCard());
dealer.addCard(theDeck.dealNextCard());
System.out.println("Cards are dealt: ");
me.printHand(true);
dealer.printHand(false);
boolean meDone = false;
boolean dealerDone = false;
String ans;
while (!meDone || !dealerDone) {
if (!meDone) {
System.out.print("Hit [H] or Stay[S])? ");
ans.input.next();
//if player hits, then add next card to deck and store if player is busted
if (ans.compareToIgnoreCase("H") == 0) {
meDone = !me.addCard(theDeck.dealNextCard());
me.printHand(true);
//if busted, done
} else {
meDone = true;
}
}
//dealer
if (!dealerDone) {
System.out.println("The Dealer hits. ");
dealerDone = !dealer.addCard(theDeck.dealNextCard());
dealer.printHand(false);
} else {
System.out.println("The Dealer stays. ");
dealerDone = true;
}
System.out.println();
}
input.close();
//print final hands
me.printHand(true);
dealer.printHand(true);
int mySum = me.getHandSum();
int dealerSum = dealer.getHandSum();
if (mySum > dealerSum && mySum <= 21 || dealerSum >21) {
System.out.println("You win.");
} else if {
System.out.println("Dealer wins.");
}
}
}
/**
*
* @author suzettepalmer
* @since 10/25/2017
*/
public class Card {
private int myNumber;
/**
* Number of card: Ace = 1, Jack = 11, Queen = 12, King = 13
*/
public Card(int aNumber) {
if (aNumber >=1 && aNumber <= 13)
{
/**
* @param aNumber = Number of the Card
*/
this.myNumber = aNumber;
} else {
System.err.println(aNumber + "is not valid.");
System.exit(1); //tells program error and exits program
}
}
/**
* @return Number of card
*/
public int getNumber()
{
return myNumber;
}
public String toString() //returns name of card
{
String numStr = "Error";
switch(this.myNumber)
{
case 1:
numStr = "Ace";
break;
case 2:
numStr = "Two";
break;
case 3:
numStr = "Three";
break;
case 4:
numStr = "Four";
break;
case 5:
numStr = "Five";
break;
case 6:
numStr = "Six";
break;
case 7:
numStr = "Seven";
break;
case 8:
numStr = "Eight";
break;
case 9:
numStr = "Nine";
break;
case 10:
numStr = "Ten";
break;
case 11:
numStr = "Jack";
break;
case 12:
numStr = "Queen";
break;
case 13:
numStr = "King";
break;
}
return numStr;
}
}
import java.util.Random;
/**
* Java deck shuffling
* @author suzettepalmer
* @since 10/23/2017
*/
public class Deck {
/**
* array of cards in deck, where top card is the first index
*/
private Card [] myCards;
/**
* Number of cards that are in deck
*/
private int numCards;
/**
*
* @param shuffle: shuffle before play
*/
public Deck(int numCards, boolean shuffle)
{
Random rng = new Random();
Card temp;
//perform swap to prevent data from being lost
int j;
for (int i = 0; i < this.numCards; i++) {
j = rng.nextInt(this.numCards);
temp = this.myCards[i];
this.myCards[i] = this.myCards[j];
this.myCards[j] = temp;
}
this.numCards = 52;
this.myCards = new Card [this.numCards];
// initiate card index
int c = 0;
//for each number
for (int n = 1; n <= 13; n++) {
//add new card to deck
this.myCards[c] = new Card(n);
c++;
}
}
public void shuffle()
{
/**
* Deal top card
* @return dealt card
*/
}
public Card dealNextCard() {
Card top = this.myCards [0];
//shift cards by 1 to the left
for (int c = 1; c < this.numCards; c++) {
this.myCards[c-1] = this.myCards[c];
}
this.myCards[this.numCards-1] = null; //when last card is used.
//decrement the number of cards in deck
this.numCards--;
return top;
}
/**
* Print top of cards on deck
* @param numToPrint (# of cards from the top of deck to print)
*/
public void printDeck(int numToPrint)
{
for (int c =0; c //printf give string and formatting guidelines; allows formating string, %3d print integer with width of 3 and padded to L with spaces, %s print string print new line System.out.printf("% 3d/%d%s ", c+1, this.numCards, this.myCards[c].toString()); System.out.printf(" "); } } } /** * * @author suzettepalmer * */ public class Player { private String name; private Card[] hand = new Card [10]; //allocating memory block for cards drawn private int numCards; public Player (String aName) { this.name = aName; //set player's hand to zero this.emptyHand(); } public void emptyHand() { /* * whether game is still occurring or not */ for (int c = 0; c <10; c++) { this.hand[c] = null; } this.numCards = 0; //defaults to 0 if not specified } public boolean addCard(Card, aCard) { if (this.numCards == 10) { System.err.printf("%s's hand already has 10 cards; " + "cannot add another ", this.name); System.exit(1); } this.hand[this.numCards] = aCard; this.numCards++; return (this.getHandSum() <= 21); } public int getHandSum() { int handSum = 0; int cardNum; int numAces = 0; for (int c = 0; c cardNum = this.hand[c].getNumber(); if (cardNum == 1) { numAces++; handSum += 11; } else if (cardNum > 10) { handSum += 10; } else { handSum += cardNum; } } while (handSum >21 && numAces > 0) { handSum -= 10; numAces--; } return handSum; } public void printHand(boolean showFirstCard) { System.out.printf("%s's cards : ", this.name); for (int c = 0; c System.out.printf(" %s ", this.hand[c].toString()); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
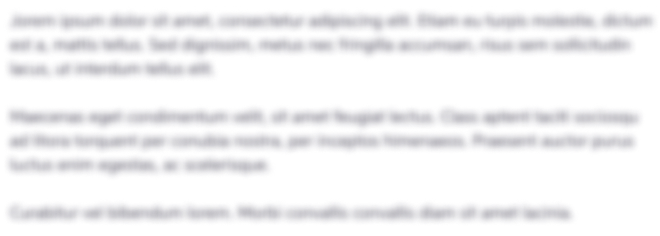
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started