Question
Hello Experts, Please answer the following question written in C. The source code and output is below. Will UPVOTE. Write a program called parkingSimulator.c that
Hello Experts, Please answer the following question written in C. The source code and output is below. Will UPVOTE.
Write a program called parkingSimulator.c that contains the following main() function: int main() { Car car1, car2, car3, car4, car5, car6, car7, car8, car9; ParkingLot p1, p2; // Set up 9 cars initializeCar(&car1, "ABC 123", 0); initializeCar(&car2, "ABC 124", 0); initializeCar(&car3, "ABD 314", 0); initializeCar(&car4, "ADE 901", 0); initializeCar(&car5, "AFR 304", 0); initializeCar(&car6, "AGD 888", 0); initializeCar(&car7, "AAA 111", 0); initializeCar(&car8, "ABB 001", 0); initializeCar(&car9, "XYZ 678", 1); // Set up two parking lots initializeLot(&p1, 1, 4, 5.5, 20.0); initializeLot(&p2, 2, 6, 3.0, 12.0); printLotInfo(p1); printLotInfo(p2); printf(" "); // Simulate cars entering the lots carEnters(&p1, &car1, 7, 15); carEnters(&p1, &car2, 7, 25); carEnters(&p2, &car3, 8, 0); carEnters(&p2, &car4, 8, 10); carEnters(&p1, &car5, 8, 15); carEnters(&p1, &car6, 8, 20); carEnters(&p1, &car7, 8, 30); carEnters(&p2, &car7, 8, 32); carEnters(&p2, &car8, 8, 50); carEnters(&p2, &car9, 8, 55); printf(" "); printLotInfo(p1); printLotInfo(p2); printf(" "); // Simulate cars leaving the lots carLeaves(&p2, &car4, 9, 0); carLeaves(&p1, &car2, 9, 5); carLeaves(&p1, &car6, 10, 0); carLeaves(&p1, &car1, 10, 30); carLeaves(&p2, &car8, 13, 0); carLeaves(&p2, &car9, 15, 15); carEnters(&p1, &car8, 17, 10); carLeaves(&p1, &car5, 17, 50); carLeaves(&p2, &car7, 18, 0); carLeaves(&p2, &car3, 18, 15); carLeaves(&p1, &car8, 20, 55); printf(" "); printLotInfo(p1); printLotInfo(p2); printf(" "); // Display the total revenue printf("Total revenue of Lot 1 is $%4.2f ", p1.revenue); printf("Total revenue of Lot 2 is $%4.2f ", p2.revenue); }
Create the following Time struct related functions: // Sets the hours and minutes amount for the given time t based // on the specified hours h. (e.g., 1.25 hours would be 1 hour // and 15 minutes) void setHours(Time *t, double h) { ... } // Takes two Time objects (not pointers) and computes the difference // in time from t1 to t2 and then stores that difference in the diff // Time (which must be a pointer) void difference(Time t1, Time t2, Time *diff) { ... }
Create the following Car struct related functions: // Initialize the car pointed to by c to have the given plate and // hasPermit status. The car should have its lotParkedIn set to // 0 and enteringTime to be -1 hours and -1 minutes. void initializeCar(Car *c, char *plate, char hasPermit) { ... }
Create the following ParkingLot struct related functions: // Initialize the lot pointed to by p to have the given number, // capacity, hourly rate and max charge values. The currentCarCount // and revenue should be at 0. void initializeLot(ParkingLot *p, int num, int cap, double rate, double max) { ... } // Print out the parking lot parameters so that is displays as // follows: // Parking Lot #2 - rate = $3.00, capacity 6, current cars 5 void printLotInfo(ParkingLot p) { ... }
Add appropriate functions called carEnters() and carLeaves() so that the main method above works correctly and produces the following OUTPUT results exactly:
Parking Lot #1 - rate = $5.50, capacity 4, current cars 0 Parking Lot #2 - rate = $3.00, capacity 6, current cars 0
Car ABC 123 enters Lot 1 at 7:15. Car ABC 124 enters Lot 1 at 7:25. Car ABD 314 enters Lot 2 at 8:00. Car ADE 901 enters Lot 2 at 8:10. Car AFR 304 enters Lot 1 at 8:15. Car AGD 888 enters Lot 1 at 8:20. Car AAA 111 arrives at Lot 1 at 8:30, but the lot is full. Car AAA 111 cannot get in. Car AAA 111 enters Lot 2 at 8:32. Car ABB 001 enters Lot 2 at 8:50. Car XYZ 678 enters Lot 2 at 8:55.
Parking Lot #1 - rate = $5.50, capacity 4, current cars 4 Parking Lot #2 - rate = $3.00, capacity 6, current cars 5
Car ADE 901 leaves Lot 2 at 9:00 paid $3.00. Car ABC 124 leaves Lot 1 at 9:05 paid $11.00. Car AGD 888 leaves Lot 1 at 10:00 paid $11.00. Car ABC 123 leaves Lot 1 at 10:30 paid $20.00. Car ABB 001 leaves Lot 2 at 13:00 paid $12.00. Car XYZ 678 leaves Lot 2 at 15:15. Car ABB 001 enters Lot 1 at 17:10. Car AFR 304 leaves Lot 1 at 17:50 paid $20.00. Car AAA 111 leaves Lot 2 at 18:00 paid $12.00. Car ABD 314 leaves Lot 2 at 18:15 paid $12.00. Car ABB 001 leaves Lot 1 at 20:55 paid $20.00.
Parking Lot #1 - rate = $5.50, capacity 4, current cars 0 Parking Lot #2 - rate = $3.00, capacity 6, current cars 0
Total revenue of Lot 1 is $82.00 Total revenue of Lot 2 is $39.00
This code simulates a few cars parking in two parking lots. To make it work, you will have to follow the directions below carefully. You MAY NOT alter the main() function code in any way. Follow the steps below and pay attention to the clear direction as to parameter types and what each function should do. Make sure to use comments in your code and make sure that it is neat and organized Just to make one thing clear.. even though we will have multiple cars parking in the parking lots... at this point the parking lot does NOT keep an array of cars Create a struct called Time that stores an hour and minute amount. This could be used to represent a time of day or an amount of time that has elapsed Create a struct called Car that stores a plateNumber for a car (which must be achar pointer). A single character which indicates whether or not the car has a permit, an enteringTime which is a Time struct that indicates the time that the car entered the lot, and a lotParkedln number which is an int indicating the number of the lot that the car is parked in Create a struct called ParkingLot that stores a lotNumber (as an integer) to represent it's unique ID, an hourlyRate (a double) representing the amount that a car owner would pay per hour to be parked in that lot, a maxCharge (a double) indicating the maximum amount that the car owner would pay to park the car for the day, an integer capacity representing the maximum number of cars that can park in that lot, a currentCarCount indicating the number of cars currently parked in the lot, and finally a revenue (a double) that keeps track of the amount of money taken in by that lot so far Create the following Time struct related functionsStep by Step Solution
There are 3 Steps involved in it
Step: 1
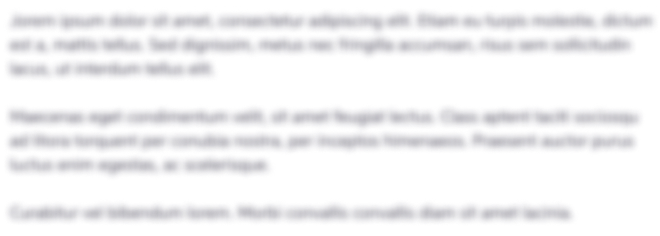
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started