Question
Hello, guys! I hope you'd able to help me with this question in java about doubly linked list. I am trouble with method1: deleteLetter(char c),
Hello, guys! I hope you'd able to help me with this question in java about doubly linked list.
I am trouble with method1: deleteLetter(char c), method 2: removeFirstLast(), method 3: makePallindrome. method4: lettetPresent(char l)
Method 1
public void deleteLetter(char c){
//your code here
}
This method deletes all instances of the letter provided as the parameter from the doubly lined list. For example: myList.delete(n), all n characters will be deleted from the doubly linked list. In the example below, annex to would be reduced to aex. See Figure 1.
Figure1
Method 2
public void removeFirstLast(){
//your code here
}
This method removes the character(s) that are at the head and tail of the doubly linked list. For example: myList.removeFirstLast(), on a doubly linked list containing annex would reduce it to nne. See Figure2.
Figure2
Method 3
public void makePallindrome(){
//your code here
}
This method takes the existing letters in the doubly linked list and makes it a palindrome (using the tail as the pivot for the mirror image). For example: myList.makePallindrome(), on a doubly linked list containing aex would result in a doubly linked list that contains aexea. See Figure3.
Figure3
Method 4
public boolean letterPresent(char l){
//your code here
}
This method returns true if the char in the method parameter is present in the doubly linked list and false if it is not present. For example: myList.letterPresent(a) and myList.letterPresent(z) will return true and false respectively if called on a double linked list containing annex.
import java.util.*;
class Node
{
private char letter;
private Node next, prev;
public Node(char c)
{
letter = c;
next = prev = null;
}
public char getLetter()
{
return letter;
}
public Node getNext()
{
return next;
}
public void setNext(Node n)
{
next = n;
}
public Node getPrev()
{
return prev;
}
public void setPrev(Node p)
{
prev = p;
}
}
public class L1Example
{
Node head, tail;
public L1Example()
{
head = tail = null;
}
public boolean isPalindrome()
{
//your code here
return false;
}
////////////////////////////////////////////////////////////////////////////////////
public void deleteLetter(char c)
{
}
public void removeFirstLast()
{
//this method deletes the first node
if (head == null)
{
return;
}
if (head.getNext() == null)
{
head = null;
return;
}
head = head.getNext();
head.prev() = null;
//this is a reference
Node c = head;
// this method deletes the last node.
while (c.getNext() != null)
c = c.getNext();
c.prev.next() = null;
}
public void makePallindrome()
{
}
/*public boolean letterPresent(char l)
{
}*/
////////////////////////////////////////////////////////////////////////////////////
public String concatinateBackward()
{
Node curr = tail;
String backward=" ";
while(curr!=null)
{
backward = backward+curr.getLetter();
curr = curr.getPrev();
}
return backward;
}
public void firstBecomesLast()
{
}
public void insert(char c)
{
Node nd = new Node(c);
nd.setNext(head);
if(head != null)
head.setPrev(nd);
else
tail = nd;
head = nd;
}
public void insert2(char c)
{
}
public static void main(String[] args)
{
L1Example myList = new L1Example();
myList.insert('a');
myList.insert('b');
myList.insert('c');
myList.insert('c');
myList.insert('d');
myList.insert('e');
System.out.println("The list read from tail to head:" +myList.concatinateBackward());
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
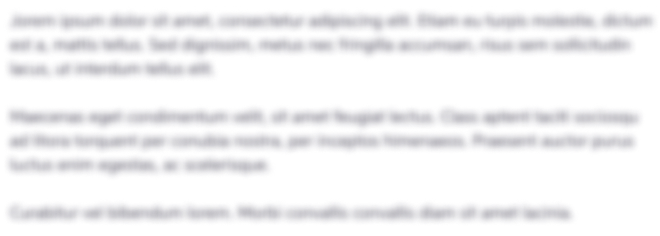
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started