Question
Hello i am mainly stuck on the arraylist. i wonderstand how to make arraylist, but what i confused is despoiting the balance into the arraylist
Hello i am mainly stuck on the arraylist. i wonderstand how to make arraylist, but what i confused is despoiting the balance into the arraylist and transfering them etc.
The goal of this assignment is to give you some experience building a program that uses objects. You must work in teams of 2 to complete this assignment. You will use the BankAccount class you created in Lab 3 to create a banking program. The program must display a menu to the user to allow the user to select a transaction like deposit, withdraw, transfer, create a bank account, and quit the program. The program should allow a user to perform multiple transactions until they quit the program by selecting the quit option. The program must perform all operations on the BankAccount objects your program will store. The operations must search through the collection of BankAccount objects and find the correct object to perform the operation on. When a user creates an account, your program should create a new BankAccount object with the user's input values and store it in an Array or ArrayList.
private String name;
private int accountNumber;
private int PIN;
private double balance;
public BankAccount(int accN, int pin) {
accountNumber = accN;
PIN = pin;
}
public BankAccount () {
name = "Undefined";
accountNumber = 0;
PIN = 0;
balance = 0;
}
public String getName() {
return name;
}
public int getAccountNumber() {
return accountNumber;
}
public int getPIN() {
return PIN;
}
public double getBalance() {
return balance;
}
public void setName(String name) {
this.name = name;
}
public void setAccountNumber(int accountNumber) {
this.accountNumber = accountNumber;
}
public void setPIN(int pIN) {
PIN = pIN;
}
public void setBalance(double balance) {
this.balance = balance;
}
public void withdrawal(double amount) {
if(amount <= balance) {
balance = balance - amount;
}
}
public void deposit(double amount) {
balance += amount;
}
public void transferFunds (double amount, BankAccount toBankAccount) {
if(amount >= balance) {
toBankAccount.setBalance(amount + toBankAccount.getBalance());
balance -= amount;
}
}
@Override
public String toString() {
return "{id:"+accountNumber+", name:"+name+", pin:"+PIN+", balance:$"+balance+"}";
}
}
#########
public class BankAccountTest {
public static void main(String[] args) {
BankAccount myAccount = new BankAccount(526323450, 1234);
myAccount.setName("Pravesh");
myAccount.setBalance(345.64);
System.out.println(myAccount);
BankAccount anotherAccount = new BankAccount(); //default constructor
System.out.println(anotherAccount);
}
}
/*
Sample run:
{id:526323450, name:Pravesh, pin:1234, balance:$345.64}
{id:0, name:Undefined, pin:0, balance:$0.0}
*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
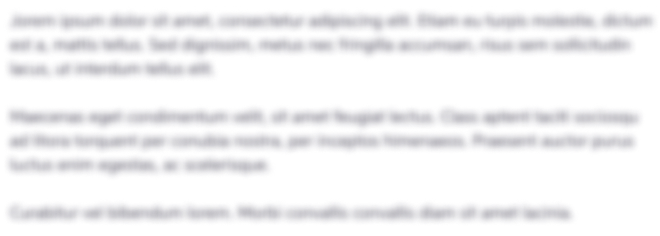
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started