Question
Hello, I have worked on the following question and I wanted to be sure if everything is right? If there are mistakes, please help me
Hello, I have worked on the following question and I wanted to be sure if everything is right? If there are mistakes, please help me by correcting them as I have given every detail. Thank you so much :)
Write a parent class that describes a parcel (like a package) and a child class that describes an overnight parcel.
A parcel is described by:
- id (which might contain numbers and letters)
- weight (described as the number of pounds)
An overnight parcel is described by id and weight and also:
- whether or not a signature is required upon deliver
1. Write the class header and the instance data variables for the Parcel class.
public class Parcel { // the parcel class has two instance variables or fields private String id; private int weight;
2.Write two constructors for the Parcel class.
- the first constructor takes in both pieces of information (id and weight)
- the second constructor takes in only the id and uses a default value for the weight (you can decide the default value)
public Parcel (String id, int weight) { this.id = id; this.weight = weight; } //2nd constructor public Parcel (String id) { this.id = id; this.weight = 23; }
3.Write getters and setters for the instance data variables of the parcel class. Include validity checks where appropriate.
public String getID() { return this.id; } public int getWEIGHT() { return this.weight; } public void setID ( String id) { this.id = id; } public void setWEIGHT (int weight) { this.weight = weight; }
4. Write a toString method. The text representation of a parcel should include the id and weight.
public String toString () { return ("The confirmation id is" + id + "the weight of the parcel is" + weight); }
5.Override the equals method inherited from object. Two parcels are the same (logically equivalent) if they have the same id and same weight.
public boolean equals (Parcel p1) { if (p1 == this) { return true; } if (!(p1 instanceof Parcel)) { return false; } return (p1.id==this.id) && (p1.weight==this.weight);
}
6. Write code that would go inside the main method of a driver/tester program.
- Create two parcel objects. Use both constructors.
- Invoke one method on each object.
- Print both objects to the console.
public static void main (String [] args) { Parcel p1 = new Parcel ("YKQ12", 23); Parcel p2 = new Parcel ("YKQ12", 23); if (p1.equals(p2)) { System.out.println("Both of the parcels are same."); } else { System.out.println("The parcels are different."); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
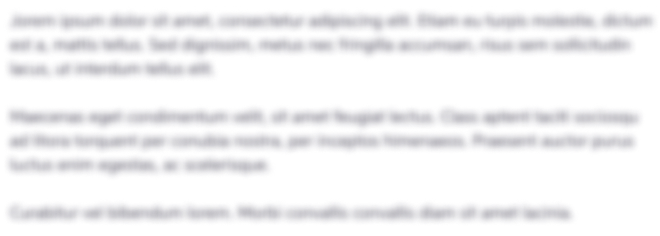
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started