Question
Hello, I need help reorganizing my classes into modules. In other words, I need each class to be in a different file, and the main
Hello, I need help reorganizing my classes into modules. In other words, I need each class to be in a different file, and the main file called main_program calls all the classes.
Please, include screenshots of your code.
Thank you very much for your help.
This is the code:
class Individual:
def __init__(self, userId, name, emailAddress):
self.userId = userId
self.name = name
self.emailAddress = emailAddress
def displayInformation(self):
print("ID: " + str(self.userId))
print("Name: " + str(self.userId))
print("Email: " + str(self.userId))
# child class Instructor inherits from Individual class
class Instructor(Individual):
def __init__(self, userId, name, emailAddress, institution, highestDegree):
super().__init__(userId, name, emailAddress)
self.institution = institution
self.highestDegree = highestDegree
def displayInformation(self):
print("Instructor ")
super().displayInformation()
print("Institution: " + str(self.userId))
print("Highest Degree: " + str(self.userId))
# child class Student inherits from Individual class
class Student(Individual):
def __init__(self, userId, name, emailAddress, program):
super().__init__(userId, name, emailAddress)
self.program = program
def displayInformation(self):
print("Student")
super().displayInformation()
print("Program of Study:" + str(self.program))
# validator class to validate user input
class Validator:
def validateId(userId, passed_max_length):
if len(userId) <= passed_max_length and userId.isdigit():
return True
else:
print("This is inputted improperly. Please try again")
return False
def validateUser(answer):
if answer:
return True
else:
print("Input is missing. Please try again.")
return False
# Validating email
def getEmail(self):
email_ok = False
while not email_ok:
user_email = input("Enter User email: ")
email_ok = self.isEmailOK(user_email)
return user_email
# method to get student id from user
def getStudentId(self):
userInput = False
while not userInput:
userId = input("Enter User Id: ")
userInput = Validator.validateId(userId, 7)
return userId
# metod to get individual name
def getName(self):
name_ok = False
while not name_ok:
user_name = input("Enter User name: ")
name_ok = self.isNameOK(user_name)
return user_name
# method to get instructor id from user
def getinstructorID(self):
userInput = False
while not userInput:
userId = input("Enter User Id: ")
userInput = Validator.validateId(userId, 5)
return userId
# method to get last institution name from user
def getLastInstitution(self):
institution_OK = False
while not institution_OK:
last_institution = input("Enter last institution: ")
institution_OK = Validator.validateUser(last_institution)
# if last_institution: # make all while loop like this
#
# institution_OK = True
return institution_OK
# method to get highet degree name from user
def getDegree(self):
userInput = False
while not userInput:
highestDegree = input("Enter highest degree: ")
userInput = Validator.validateUser(highestDegree)
return highestDegree
# method to get program name from user
def getProgram(self):
userInput = False
while not userInput:
program = input("Enter program name: ")
userInput = Validator.validateUser(program)
return program
# validating email
def isNameOK(self, passed_user_name):
bad_chars = ["!", '"', "@", "#", "$", "%", "^", "&", "*", "(", ")", "_", "=", "+", ",", "<", ">", "/", "?", ";",
":",
"[", "]", "{", "}", "\\", "."]
if passed_user_name:
for character in passed_user_name:
if character in bad_chars or character.isdigit():
print("User name is incorrect. Please try again.")
return False
return True
else:
print("User name is missing. Please try again.")
return False
# Validating email
def isEmailOK(self, passed_user_email):
bad_chars = ["!", '"', "#", "$", "%", "^", "&", "*", "(", ")", "_", "=", "+", ",", "<", ">", "/", "?", ";", ":",
"[",
"]", "{", "}", "\\"]
if passed_user_email:
for character in passed_user_email:
if character in bad_chars or character.isdigit():
print("User email is incorrect. Please try again.")
return False
return True
else:
print("User email is missing. Please try again.")
return False
my_validator = Validator()
# list to store the records
college_records = []
print("Store college records")
# while loop
while True:
name = my_validator.getName()
emailAddress = my_validator.getEmail()
user = input("Please select type of user: 1 for instructor, 2 for student, 3 exit/print ")
# user = int(input("Please select type of user: 1 for instructor, 2 for student, 3 exit/print "))
if user.isdigit(): # checks if it's a digit
user = int(user)
else:
wrong_choice = True
while wrong_choice:
print("I need an integer in 1, 2 or 3 to continue.")
user = input("Please select type of user: 1 for instructor, 2 for student, 3 exit/print ")
if user.isdigit():
wrong_choice = False
user = int(user)
# if user is instructor
if user == 1:
temp_instructor_ID = my_validator.getinstructorID()
# temp_name = my_validator.getName()
#
# temp_emailAddress = my_validator.getEmail()
temp_institution = my_validator.getLastInstitution()
temp_degree = my_validator.getDegree()
# instructor_instance = Instructor(temp_instructor_ID, temp_name, temp_emailAddress, temp_institution,
# temp_degree)
instructor_instance = Instructor(temp_instructor_ID, name, emailAddress, temp_institution,temp_degree)
college_records.append(instructor_instance)
# if user is student
elif user == 2:
temp_student_userId = my_validator.getStudentId()
# temp_student_name = my_validator.getName()
#
# temp_student_emailAddress = my_validator.getEmail()
temp_student_program = my_validator.getProgram()
# Student_instance = Student(temp_student_userId, temp_student_name, temp_student_emailAddress,temp_student_program)
Student_instance = Student(temp_student_userId, name, emailAddress,temp_student_program)
college_records.append(Student_instance)
else:
break
# loop through your college_records list and get everything printed out.
for individual in college_records:
individual.displayInformation()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
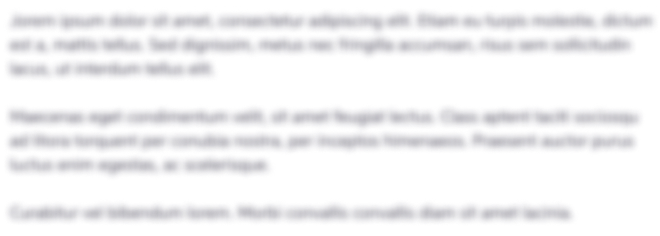
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started