Question
Hello! I need help with writing protions of the code and altering it so the operands are actual numbers and not variables such as, a,
Hello! I need help with writing protions of the code and altering it so the operands are actual numbers and not variables such as, a, b, c. Please edit and write out the missing methods. Thanks! Below I have posted the requirements and method descriptions and the code I have written partially. I have already created an arraystack class.
public class InfixExpression {
private String str;
public InfixExpression(String str) {
this.str = str;
clean();
if (!isValid()) {
throw new IllegalArgumentException();
}
}
@Override
public String toString() {
return str;
}
private boolean isBalanced() {
StackInterface
int characterCount = str.length();
boolean isBalanced = true;
int index = 0;
char nextCharacter = ' ';
while (isBalanced && (index
nextCharacter = str.charAt(index);
switch (nextCharacter) {
case '(':
case '[':
case '{':
openDelimiterStack.push(nextCharacter);
break;
case ')':
case ']':
case '}':
if (openDelimiterStack.isEmpty())
isBalanced = false;
else {
char openDelimiter = openDelimiterStack.pop();
isBalanced = isPaired(openDelimiter, nextCharacter);
}
break;
default:
break;
}
index++;
}
if (!openDelimiterStack.isEmpty()) {
isBalanced = false;
}
return isBalanced;
}
private boolean isPaired(char open, char close) {
return ((open == '(' && close == ')') || (open == '[' && close == ']') || (open == '{' && close == '}'));
}
private boolean isValid() {
return false;
}
private void clean() {
String newStr = "";
if (str.contains(" ")) {
str.replace(" ", "");
}
for (int i = 0; i
newStr = newStr + str.charAt(i) + " ";
}
str = newStr;
}
// Modify operands will not be abcdefghijklmnopqrstuvwyxz. They will be numbers
// 1, 2, 3, ... infinity.
public String getPostfixExperssion() {
StackInterface
String postFix = "";
int index = 0;
while (!str.isEmpty()) {
char nextCharacter = str.charAt(index);
index++;
switch (nextCharacter) {
case 'a':
case 'b':
case 'c':
case 'd':
case 'e':
case 'f':
case 'g':
case 'h':
case 'i':
case 'j':
case 'k':
case 'l':
case 'm':
case 'n':
case 'o':
case 'p':
case 'q':
case 'r':
case 's':
case 't':
case 'u':
case 'v':
case 'w':
case 'x':
case 'y':
case 'z':
postFix = postFix + nextCharacter;
break;
case '^':
operatorStack.push(nextCharacter);
case '+':
case '-':
case '*':
case '/':
while ((!operatorStack.isEmpty())
&& (precedenceOf(nextCharacter)
postFix = postFix + operatorStack.peek();
operatorStack.pop();
}
operatorStack.push(nextCharacter);
break;
case '(':
operatorStack.push(nextCharacter);
break;
case ')':
char topOperator = operatorStack.pop();
while (topOperator != '(') {
postFix = postFix + topOperator;
topOperator = operatorStack.pop();
}
break;
default:
break;
}
}
while (!operatorStack.isEmpty()) {
char topOperator = operatorStack.pop();
postFix = postFix + topOperator;
}
return postFix;
}
private int precedenceOf(char nextCharacter) {
// TODO Auto-generated method stub
return 0;
}
// Modfiy to have case for operands 1, 2, 3, ... infinity
// Need to parse integers for operands
public int evaluate() {
if (!isValid()) {
throw new IllegalArgumentException();
}
String postFix = getPostfixExperssion();
StackInterface
int index = 0;
while (!postFix.isEmpty()) {
char nextCharacter = postFix.charAt(index);
index++;
switch (nextCharacter) {
case '+':
case '-':
case '*':
case '/':
case '^':
char operandTwo = valueStack.pop();
char operandOne = valueStack.pop();
char result = evaluateOperands(nextCharacter, operandOne, operandTwo);
valueStack.push(result);
break;
default:
break;
}
}
return valueStack.peek();
}
private char evaluateOperands(char nextCharacter, char operandOne, char operandTwo) {
// TODO Auto-generated method stub
return 'a';
}
}
XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
public class Tester {
public static void main(String[] args) {
StackInterface
stack.push(1);
stack.push(5);
stack.push(3);
System.out.println("-----------Testing ArrayStack-----------");
System.out.println("Pushing 1, 5, 3, and peeking for a 3:");
System.out.println(stack.peek()); // Gives 3
stack.pop();
System.out.println("Poping 3, and peeking for a 5:");
System.out.println(stack.peek()); // Gives 5
System.out.println("Checking to see if empty:");
System.out.println(stack.isEmpty()); // Gives false
stack.clear();
System.out.println("Clearing stack and seeing if empty:");
System.out.println(stack.isEmpty()); // Gives true
//System.out.println(stack.peek()); Gives an EmptyStackException
System.out.println("");
System.out.println("-----------Testing InfixExpression-----------");
//InfixExpression infixEx
}
}
XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
import java.util.Arrays;
import java.util.EmptyStackException;
public class ArrayStack
private T[] stack;
private int topIndex;
private int DEFAULT_CAPACITY = 10;
public ArrayStack() {
@SuppressWarnings("unchecked")
T[] tempStack = (T[]) new Object[DEFAULT_CAPACITY];
stack = tempStack;
topIndex = -1;
}
@Override
public void push(T newEntry) {
ensureCapacity();
stack[topIndex + 1] = newEntry;
topIndex++;
}
@Override
public T pop() {
if (isEmpty()) {
throwEmptyStackException();
}
T top = stack[topIndex];
stack[topIndex] = null;
topIndex--;
return top;
}
@Override
public T peek() {
if (isEmpty()) {
throwEmptyStackException();
}
return stack[topIndex];
}
@Override
public boolean isEmpty() {
return topIndex
}
@Override
public void clear() {
while (!isEmpty()) {
pop();
}
topIndex = 0;
}
private void throwEmptyStackException() {
if (isEmpty()) {
throw new EmptyStackException();
}
}
private void ensureCapacity() {
if (topIndex == stack.length - 1) {
int newLength = 2 * stack.length;
stack = Arrays.copyOf(stack, newLength);
}
}
}
Naming requirements (not following any of these may result in a score of 0) You will have exactly four source code files: Stacklnterface.java (an interface you are provided) ArrayStack.java (your array-based implementation of Stacklnterface), InfixExpression.java, and Tester.java You will use the default package (this means there should be no package statements in any of your files). Preliminaries: Review the algorithms in chapter 5 for: Determining whether parentheses in an algebraic expression are balanced correctly Converting an infix expression to a postfix expression Evaluating a postfix expression Read chapter 6 to understand how to implement a stack using an array Overall goal: Naming requirements (not following any of these may result in a score of 0) You will have exactly four source code files: Stacklnterface.java (an interface you are provided) ArrayStack.java (your array-based implementation of Stacklnterface), InfixExpression.java, and Tester.java You will use the default package (this means there should be no package statements in any of your files). Preliminaries: Review the algorithms in chapter 5 for: Determining whether parentheses in an algebraic expression are balanced correctly Converting an infix expression to a postfix expression Evaluating a postfix expression Read chapter 6 to understand how to implement a stack using an array Overall goalStep by Step Solution
There are 3 Steps involved in it
Step: 1
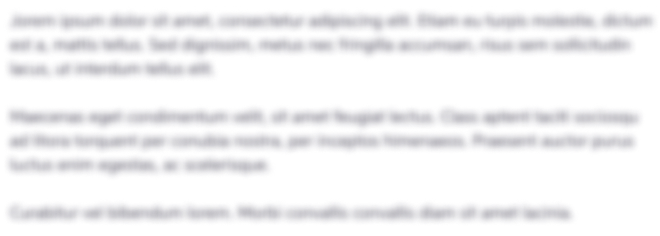
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started