Question
Hello, I was wondering if I could help on this problem and how it was approached. Thank You. Example: public class FunFunFun { public static
Hello, I was wondering if I could help on this problem and how it was approached. Thank You. Example: public class FunFunFun { public static void main(String[] args) { FunFunFun app = new FunFunFun(); System.out.println(app.fastCountDown(3)); app.gcd(1440,408,0); System.out.println( "T(4) = " + app.eTime(4) ); } public String fastCountDown( /*parameter here */ ) { /* code here */ } public int gcd( /*parameter(s) here */ ) { /* code here */ } public int eTime( /*parameter here */ ) { /* code here */ } }
1ST METHOD: fastCountDown()
Write a recursive method that counts down by two's. Should return a string of all numbers counted with a space after each one. Here's the output for System.out.println(fastCountDown(7)):
7 5 3 1
Here's the output for System.out.println(fastCountDown(6)):
6 4 2 0
2ND METHOD gcd()
Euclid's Algorithm
The greatest common divisor (gcd) of two positive integers is the largest integer that divides evenly into both of them. For example, the greatest common divisor of 102 and 68 is 34 since both 102 and 68 are multiples of 34, but no integer larger than 34 divides evenly into 102 and 68.
We can efficiently compute the gcd using the following property, which holds for positive integers p and q:
If p > q, the gcd of p and q is the same as the gcd of q and p % q.
The function gcd() is a compact recursive function whose reduction step is based on this property. It should end up returning the greatest common divisor of the two numbers. Here's the output:
gcd(1440, 408) gcd(408, 216) gcd(216, 24) gcd(192, 24) gcd(24, 0) return 24 return 24 return 24 return 24 return 24
The tricky part of this program is the indentation. Hint: You need to add 3 spaces for each call to gcd() which requires knowing what the current level is in relation to the first call so that you can loop (probably) adding spaces to the output string. This is easily accomplished by passing an additional argument to gcd().
3RD METHOD: eTime()
Exponential Time exponential growth
One legend says that the world will end when a certain group of monks solves the Towers of Hanoi problem in a temple with 64 golden discs on three diamond needles. We can estimate the amount of time until the end of the world (assuming that the legend is true). If we define the function T(n) to be the number of move directives issued by Towers of Hanoi program to move n discs from one peg to another, then the recursive code implies that T(n) must satisfy the following equation:
T(n) = 2 T(n - 1) + 1 for n > 1, with T(1) = 1
Such an equation is known in discrete mathematics as a recurrence relation. We can often use them to derive a closed-form expression for the quantity of interest. For example, T(1) = 1, T(2) = 3, T(3) = 7, and T(4) = 15. In general, T(n) = 2n - 1.
Knowing the value of T(n), we can estimate the amount of time required to perform all the moves. If the monks move discs at the rate of one per second, it would take more than one week for them to finish a 20-disc problem, more than 31 years to finish a 30-disc problem, and more than 348 centuries for them to finish a 40-disc problem (assuming that they do not make a mistake). The 64-disc problem would take more than 5.8 billion centuries.
Write a method eTime() that calculates T(n), obviously keeping n fairly small. (Why?) The output, given System.out.println( "T(4) = " + app.eTime(4) ); is
T(4) = 15
Step by Step Solution
There are 3 Steps involved in it
Step: 1
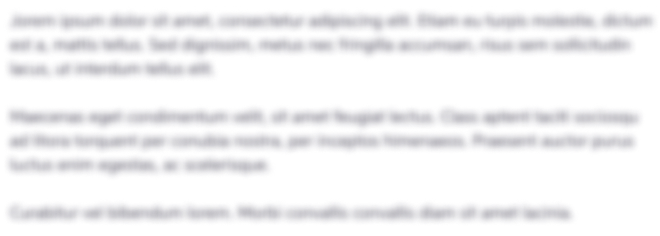
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started