Question
HELP ME HOMEWORK C++. NOTE: CODE HERE is I need you to help me write code because I confused to do it. DO NOT CHANGE
HELP ME HOMEWORK C++. NOTE: CODE HERE is I need you to help me write code because I confused to do it. DO NOT CHANGE COMPLEX.H AND IMAGINARY.H THIS IS CLASS-DEFAULT. Thank you so much.
This assignment will teach you proper class design. Making ADTs, writing constructors, overloading operators, separate header and implementation files, etc. This assignment is a reprise of an early CSCI 40 assignment called "simple calculator". Whereas for that assignment you had to do addition, subtraction, multiplication, division, and modulus, for this assignment you only have to do addition, subtraction, multiplication, and exponentiation. However, for this assignment, you will be doing complex math. When your code works right, you'll enter a line like this: 1 1 + 2 3 This means, 1+1i + 2+3i So your code will output: 3+4i To implement this assignment, you must: 1) Implement a class to handle imaginary numbers. Overload operators for +, -, *, etc. in it. Imaginary numbers work kinda like ints, except when you multiply two imaginary numbers together you get an int (and a negative int at that) rather than another imaginary. Look inside imagine.cc to see what functions you need to write. 2) Implement a class to handle complex numbers. A complex number is a combination of an int and an imaginary number (so your complex class should include the imaginary class). You must overload various operators for it as well. Complex.cc has a list of all the functions you must write. They are just stubs right now. Multiplication is probably the most complicated, but remember FOIL from algebra, and you can use the overloads for the imaginary class to make it simple. You must handle all I/O for these classes as well, with main controlling everything. If an error occurs in input, you must output -1 and quit. When the user inputs 0 0, this means the program is over and you must quit.
complex.h
#pragma once #include
class Complex { private: int real; Imaginary imagine; public: //YOU: Implement all these functions Complex(); //Default constructor Complex(int new_real, Imaginary new_imagine); //Two parameter constructor Complex operator+(const Complex &rhs) const; Complex operator-(const Complex &rhs) const; Complex operator*(const Complex &rhs) const; bool operator==(const Complex &rhs) const; Complex operator^(const int &exponent) const; friend ostream& operator<<(ostream &lhs,const Complex& rhs); friend istream& operator>>(istream &lhs,Complex& rhs); };
complex.cc
#include
//Class definition file for Complex
//YOU: Fill in all of these functions //There are stubs (fake functions) in there now so that it will compile //The stubs should be removed and replaced with your own code.
Complex::Complex() { real = 0; imagine = 0; }
Complex::Complex(int new_real, Imaginary new_imagine) { real = new_real; imagine = new_imagine; }
Complex Complex::operator+(const Complex &rhs) const { Complex num; num.real = real + rhs.real; num.imagine = imagine + rhs.imagine; return num; }
Complex Complex::operator-(const Complex &rhs) const { Complex num; num.real = real - rhs.real; num.imagine = imagine - rhs.imagine; return num; }
Complex Complex::operator*(const Complex &rhs) const { Complex num; num.real = real * rhs.real; num.imagine = imagine * rhs.imagine; return num; }
bool Complex::operator==(const Complex &rhs) const { if (real == rhs.real && imagine == rhs.imagine) { return true; } else{ return false; } }
Complex Complex::operator^(const int &exponent) const { CODE HERE }
//This function should output 3+5i for Complex(3,5), etc. ostream& operator<<(ostream &lhs,const Complex& rhs) { //Output a Complex here lhs <<"(" << rhs.real << ") + (" << rhs.imagine << ")i"; return lhs; }
//This function should read in two ints, and construct a // new Complex with those two ints istream& operator>>(istream &lhs,Complex& rhs) { //Read in a Complex here // int newReal, newImaginary; // cout << "Enter real part: "; lhs >> rhs.real; // cout << "Enter imaganiary part: "; lhs>> rhs.imagine; return lhs; }
imaginary.h
#pragma once #include
class Imaginary { private: int coeff; //If 5, then means 5i public: Imaginary(); Imaginary(int new_coeff); int get_coeff() const; Imaginary operator+(const Imaginary& rhs) const; //This is a "constant method" Imaginary operator-(const Imaginary& rhs) const; int operator*(const Imaginary& rhs) const; Imaginary operator*(const int& rhs) const; Imaginary operator=(const int& rhs); Imaginary operator=(const Imaginary& rhs); bool operator==(const Imaginary& rhs) const; friend ostream& operator<<(ostream& lhs, const Imaginary& rhs); friend istream& operator>>(istream& lhs, Imaginary& rhs); };
imaginary.cc
#include "imaginary.h" #include
//Sample Code - I have done the addition operator for you so you can see //what a functioning operator should look like. Given this function below, //in main() you could write the following code: // Imaginary foo(3); //foo is 3i // Imaginary bar(5); //bar is 5i // foo = foo + bar; //foo will become 8i //In the above example, this function would get called on foo, with //bar being passed in as the parameter named rhs (right hand side). Imaginary Imaginary::operator+(const Imaginary& rhs) const { return Imaginary(coeff+rhs.coeff); //My coeff is 3; rhs.coeff is 5. So construct a new one with a coeff of 8. }
//These you will need to implement yourself. //They currently are just stub functions Imaginary::Imaginary() { //Default cstor //coeff = ?? coeff = 0; }
Imaginary::Imaginary(int new_coeff) { //One parameter cstor //coeff = ?? coeff = new_coeff; }
int Imaginary::get_coeff() const { //Get function return get_coeff(); }
Imaginary Imaginary::operator-(const Imaginary& rhs) const { return Imaginary(coeff-rhs.coeff); }
Imaginary Imaginary::operator*(const int& rhs) const { //5i * 2 = 10i
CODE HERE }
int Imaginary::operator*(const Imaginary& rhs) const { //i * i = -1
CODE HERE }
//This function is functional Imaginary Imaginary::operator=(const Imaginary& rhs) { coeff = rhs.coeff; return rhs; }
//This function is functional Imaginary Imaginary::operator=(const int& rhs) { coeff = rhs; return Imaginary(rhs); }
bool Imaginary::operator==(const Imaginary& rhs) const { return (true); }
//This function is done for you. It will allow you to cout variables of type Imaginary. //For example, in main you could write: // Imaginary foo(2); // cout << foo << endl; //And this would print out "2i" ostream& operator<<(ostream& lhs, const Imaginary& rhs) { lhs << showpos; lhs << rhs.coeff << "i"; //Will echo +4i or +0i or -3i or whatever lhs << noshowpos; return lhs; }
istream& operator>>(istream& lhs, Imaginary& rhs) { int i; lhs >> i; rhs.coeff = i; return lhs; }
main.cc
#include
CODE HERE
using namespace std;
int main() { cout << boolalpha; //Print "true" instead of "1" when outputting bools Imaginary i,j; //These three lines are test code, delete them later cin >> i >> j; //Read two Imaginaries in - won't work till cstors done cout << i+j << endl; //Output the sum of them while (true) { //YOU: Read in a complex number
CODE HERE
//YOU: If it is 0 0, then break or exit
CODE HERE exit(EXIT_SUCCESS);
//YOU: Read in an operator (+,-,*,==,or ^)
CODE HERE
//YOU: Read in the second operand (another complex or an int)
CODE HERE
//YOU: Output the result
CODE HERE } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
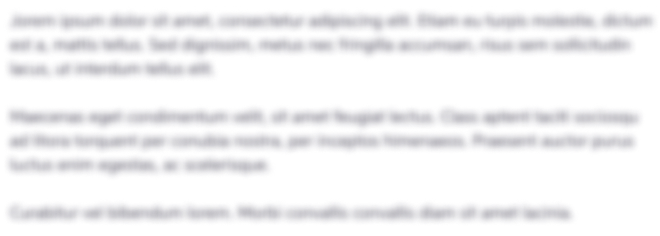
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started