Question
Help me with this code here is the instructions i need help debugging it For this assignment, you are going to pretend that you were
Help me with this code here is the instructions i need help debugging it
For this assignment, you are going to pretend that you were just hired into a job where a previous developer left the company and you are taking their spot. The code they left is incomplete, has bugs and logic flaws in it, and needs to be documented and modularized according to the standards discussed this week in class.
Here is what the program needs to do (note that these instructions should look familiar to you from IT 533 and/or the pre-assessment for IT 534):
------------------------
Write a Python program that gathers information about instructors and students according to the rules provided:
What type of individual we're dealing with (instructor or student)
If the individual is a student, we need to get their Student ID (this is required, and must be a number that is 7 or less digits long)
We also need to obtain their program of study (this is required)
If the individual is an instructor, we need to get their Instructor ID (this is required, and must be a number that is 5 or less digits long)
We also need to obtain the name of the last institution they graduated from (this is required) and highest degree earned (this is required)
The individual's name (this is required, and must be primarily comprised of upper- and lower-case letters. It also cannot contain any of the following characters: ! " @ # $ % ^ & * ( ) _ = + , < > / ? ; : [ ] { } \ ).
The individual's email address (this is required, and must be primarily comprised of alphanumeric characters. It also cannot contain any of the following characters: ! " ' # $ % ^ & * ( ) = + , < > / ? ; : [ ] { } \ ).
Write your program such that if a user inputs any of the above information incorrectly, the program asks the user to re-enter it before continuing on. Your program should accept any number of individuals until the person using the program says they are done.
You will store the information you collect in classes that you will append to a list called "college_records". There will be an Instructor class and a Student Class (which should be pretty simple), and your submission must use inheritance principles. Create a method that displays all collected information for an individual called "displayInformation".
You must also create a class called "Validator" that has methods attached to it that validate submitted information.
When your submission is through gathering data, print out all entries in the college_records list.
Your submission should use principles of augmentation where appropriate and be as flexible as possible.
------------------------
The code you need to modify can be found at this link. Your job is to thoroughly test and debug it; reorganize your classes into modules; and produce documentation (using Pydoc) for your work using the principles discussed in the lectures this week.
Be sure to also put comments in your code that clearly mark how you are performing your program logic. In the submission comments of this assignment, please place the repository URL of your file submission.
class Person():
def __init__(self, name, email, id, program, degree, institution):
self.name = name
self.email = email
self.id = id
self.program = program
self.degree = degree
self.institution = institution
def displayInformation(self):
print("Name: " + self.name)
print("Email: " + self.name)
print("ID: " + self.id)
class Student():
def __init__(program):
self.program = program
def displayInformation():
print("Program: " + self.program)
class Instructor():
def __init__(degree, institution):
super().__init__(name, email, id, degree, institution)
self.degree = degree
self.institution = institution
def displayInformation():
print("Degree: " + self.degree)
print("Institution: " + self.institution)
class Validator():
def __init__(self):
self.validate_name = ['!','"','@','#','$','%','^','&','*','(',')','_','=','+',',','<','>','/','?',';',':','[',']','{','}','\\']
self.validate_email = ['!','"','\'','#','$','%','^','&','*','(',')','=','+',',','<','>','/','?',';',':','[',']','{','}','\\']
def validate_name(self, passed_name):
if passed_name and str:
has_bad_chars = False
for character in passed_name:
if character in validate_name:
return True
else:
return False
else:
print("Your name cannot be blank")
return False
def validate_email(self, passed_email):
if passed_email and str:
has_bad_chars = False
for character in passed_email:
if character in validate_email:
has_bad_chars = True
else:
has_bad_chars = False
if not has_bad_chars:
return True
else:
print("Your email has bad characters in it")
return False
else:
print("Your email cannot be blank")
return False
def validate_user_type(self, passed_type):
if passed_type.lower == "s" or passed_type.lower == "i":
return True
else:
print("The provided user type is invalid")
return False
def validate_student_id(self, passed_id):
if passed_id.len() <= 7 and int(passed_id):
return True
else:
print("The provided student ID is invalid")
return False
def validate_instructor_id(self, passed_id):
if passed_id.len() <= 5 and int(passed_id):
return True
else:
print("The provided student ID is invalid")
return False
def validate_value(self, passed_value):
if passed_value:
return True
else:
print("You must provide a value")
return False
college_records = []
my_validator = Validator()
while True:
ind_type_valid = False
while ind_type_valid == False:
ind_type = input("Are you a Student or Instructor? Type: 'S' or 'I' ")
ind_type_valid = my_validator.validate_user_type(ind_type)
ind_name_valid = False
while ind_name_valid == False:
your_name = input("Enter your name: ")
name_valid = my_validator.validate_name(your_name)
ind_email_valid = False
while ind_email_valid == False:
your_email = input("Enter your email: ")
ind_email_valid = my_validator.validate_email(your_email)
if ind_type.lower == "s":
ind_id_valid = False
while ind_id_valid == False:
your_id = input("Enter your Student ID: ")
ind_id_valid = my_validator.validate_stud_id(your_id)
ind_program_valid = False
while ind_program_valid == False:
your_program = input("Enter your Program of Study: ")
ind_program_valid = my_validator.validate_value(your_program)
tmp_student = (your_name, your_email, your_id, your_program)
college_records.append(tmp_student)
else:
ind_id_valid = False
while ind_id_valid == False:
your_id = input("Enter your Instructor ID: ")
ind_id_valid = my_validator.validate_instructor_id(your_id)
ind_degree_valid = False
while ind_degree_valid == False:
your_degree = input("Enter your Highest Degree: ")
ind_degree_valid = my_validator.validate_value(your_degree)
ind_institution_valid = False
while ind_institution_valid == False:
your_institution = input("Enter the Last Instiution you Graduated from: ")
ind_institution_valid = my_validator.validate_value(your_institution)
tmp_instructor = Instructor{your_name, your_email, your_id, your_degree, your_institution}
college_records.append(tmp_instructor)
continue = input("Would you like to add another record (Y/N)? ")
if continue.lower == "n":
break
while college_records:
print(college_records)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
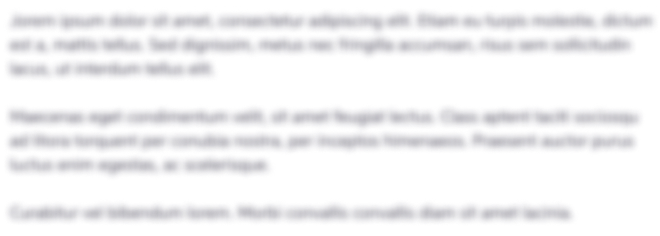
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started