Question
Here are the 4 files ********************************* package binaryTreeLAB; /* * CSC 2720, Mar.22, 2017 * Lab Instructor : Zhuojun Duan * */ public class binaryTree
Here are the 4 files *********************************
package binaryTreeLAB; /* * CSC 2720, Mar.22, 2017 * Lab Instructor : Zhuojun Duan * */
public class binaryTree { protected btNode root; /* Constructor */ public binaryTree() { root = null; } /* Function to check if tree is empty */ public boolean isEmpty() { return root == null; } /* Functions to insert data */ public void insert(int data) { root = insert(root, data); } /* Function to insert data recursively */ private btNode insert(btNode node, int data) { if (node == null) node = new btNode(data); else { if (data
/* Function for preorder traversal */ public void preorder() { preorder(root); } private void preorder(btNode r) { if (r != null) { System.out.print(r.getData() +" "); preorder(r.getLeft()); preorder(r.getRight()); } } /* * Students in the LAB should complete three methods as follows */ /* Function for inorder traversal *////////////////////////////////////////////////// /* public void inorder() { //TO DO by students } */ /* Function for postorder traversal *////////////////////////////////////////////////// /* public void postorder() { //TO DO by students }*/ /* Recursive approach to find height of binary tree /* public int findHeight(btNode root) { // TO DO by students } */
**********************************************
package binaryTreeLAB; /* * CSC 2720, Mar.22, 2017 * Lab Instructor : Zhuojun Duan * */
public class btNode {
btNode left, right; int data;
/* Constructor */ public btNode() { left = null; right = null; data = 0; } /* Constructor */ public btNode(int n) { left = null; right = null; data = n; } /* Function to set left node */ public void setLeft(btNode n) { left = n; } /* Function to set right node */ public void setRight(btNode n) { right = n; } /* Function to get left node */ public btNode getLeft() { return left; } /* Function to get right node */ public btNode getRight() { return right; } /* Function to set data to node */ public void setData(int d) { data = d; } /* Function to get data from node */ public int getData() { return data; } }
*************************************
package binaryTreeLAB;
/* * CSC 2720, Mar.22, 2017 * Lab Instructor : Zhuojun Duan * */
import java.util.ArrayList; import java.util.Collections; import java.util.List;
public class BTreePrinter { public static
printNodeInternal(Collections.singletonList(root), 1, maxLevel); }
private static
int floor = maxLevel - level; int endgeLines = (int) Math.pow(2, (Math.max(floor - 1, 0))); int firstSpaces = (int) Math.pow(2, (floor)) - 1; int betweenSpaces = (int) Math.pow(2, (floor + 1)) - 1;
BTreePrinter.printWhitespaces(firstSpaces);
List
BTreePrinter.printWhitespaces(betweenSpaces); } System.out.println("");
for (int i = 1; i
if (nodes.get(j).left != null) System.out.print("/"); else BTreePrinter.printWhitespaces(1);
BTreePrinter.printWhitespaces(i + i - 1);
if (nodes.get(j).right != null) System.out.print("\\"); else BTreePrinter.printWhitespaces(1);
BTreePrinter.printWhitespaces(endgeLines + endgeLines - i); }
System.out.println(""); }
printNodeInternal(newNodes, level + 1, maxLevel); }
private static void printWhitespaces(int count) { for (int i = 0; i
private static
return Math.max(BTreePrinter.maxLevel(node.left), BTreePrinter.maxLevel(node.right)) + 1; }
private static
return true; } }
*********************************************
package binaryTreeLAB; /* * CSC 2720, Mar.22, 2017 * Lab Instructor : Zhuojun Duan * */
import java.util.Scanner;
public class test { public static void main(String[] args) { Scanner scan = new Scanner(System.in); /* Creating object of BST */ binaryTree bst = new binaryTree(); System.out.println("Binary Search Tree Test "); char ch; int flag=0; /* Perform tree operations */ do { System.out.println("Enter integer element to insert."); bst.insert( scan.nextInt() ); System.out.println(" Do you want to continue (Type y or n) "); ch = scan.next().charAt(0); } while (ch == 'Y' || ch == 'y'); BTreePrinter.printNode(bst.root); System.out.print(" Pre order : "); bst.preorder(); /* System.out.print(" In order : "); bst.inorder(); System.out.print(" Post order : "); bst.postorder();*/ /*System.out.print(" Height of the tree is : "+bst.findHeight(bst.root));*/ }
}
please download the four classes from icollege Run the code and observe the results Students should add three methods in the binaryTree.java: public void inorder public void postorder(); public int findHeight(btNode root); Then test the three methods
Step by Step Solution
There are 3 Steps involved in it
Step: 1
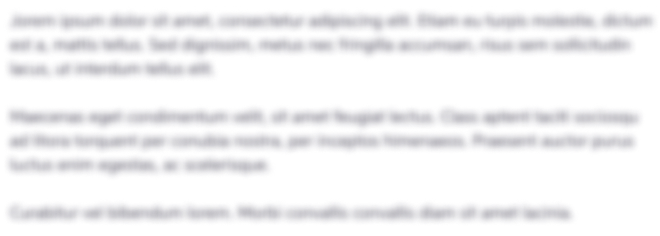
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started