Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Here is the partial code, please complete it: // Please read the given project assignment document for the project description with an illustrartive diagram. //
Here is the partial code, please complete it:
// Please read the given project assignment document for the project description with an illustrartive diagram. // You are given a partially completed program to handle a linked list that contains a book database. // Each book has the corresponding information: book name, library name, and a linked list of authors. // Please read the instructions above each required function and follow the directions carefully. // If you modify any of the given code, return types, or parameters, you risk failing test cases. // // Note, Textbook Section 2.10 gives a case study on complex linked list operations. // This project is based on that case study. Make sure you read the code in section 2.10. #includeQuestion 1 [100 points] You need to complete the following functions (example outputs are given below) Points Option key Action Function name aAdd a new book to the void addBook (char* name, char* library) 5 database c Add an author for a bookvoid addAuthor (char* bookName, char* 10 authorName) Search for a book in the struct book* searchBook (char* name) database Remove a book from the void removeBook (char* bookName) database Display the last author char* lastAuthor (char* bookName) added for a book 5 15 15 b Display the list of books struct bookList* bookslnThisLibrary (char*25 available in a particular libraryName) library Display the list of books |struct bookList* sortByName() sorted alphabetically by name Exit the programm 25 (already implemented) As a sample database, consider the following book information. To test your code you can use the 'Add new book' and 'Add an author' options to build this database/ linked list: Book name Library Authors Intro to Logic Design Java for Everyone Computer Organization Logic in Computer Science Noble Hayden Hayden Noble Alan Marcovitz Cav Horstmanrn David Patterson, John Hennesey Michael Huth, Mark Rvan Your code should work with any other book information/ database. Submit the completed p05.c#include #include #include // included to check for memory leaks // #define CRTDBG_MAP_ALLOC // for Visual Studio Only // #include // for Visual Studio Only // #pragma warning(disable: 4996) // for Visual Studio Only // global linked list 'list' contains the list of books struct bookList { struct book *book; struct bookList *next; } *list = NULL; // currently empty // structure "book" contains the book's name, library's name and linked list of authors struct book { char name[30]; char library[30]; struct author *authors; }; // linked list 'authors' contains name of authors struct author { char name[30]; struct author *next; }; // forward declaration of functions that have already been implemented // Do not modify thse functions void flushStdIn(); void actionOnList(char); void helper(char); void deleteDatabase(struct bookList*); void displayList(struct bookList*); // The following forward declarations are for functions that require implementation. Their empty definition is at the bottom of the file. // Do not modify the return types and input parameter data types // return type // name and parameters // points void addBook(char*, char*); // 5 void addAuthor(char*, char*); // 10 struct book* searchBook(char*); // 5 void removeBook(char*); // 15 char* lastAuthor(char*); // 15 struct bookList* booksInThisLibrary(char*); // 25 struct bookList* sortByName(); // 25 // Total: 100 points int main() { char ch = '\0'; printf(" CSE 220 project 5: Library database "); do { printf(" Please select an option: "); printf("\t a: Add a new book to the database "); printf("\t c: Add an author for a book "); printf("\t s: Search for a book in the database "); printf("\t r: Remove a book from the database "); printf("\t l: Display the last author added for a book "); printf("\t b: Display the list of books available in a particular library "); printf("\t n: Display the list of books sorted by name "); printf("\t e: Exit "); ch = tolower(getchar()); flushStdIn(); actionOnList(ch); } while (ch != 'e'); deleteDatabase(list); list = NULL; // _CrtDumpMemoryLeaks(); // check for memory leaks (VS will let you know in output if they exist) return 0; } // flush out ' ' characters void flushStdIn() { int c; do c = getchar(); while (c != ' ' && c != EOF); } // branch to different tasks void actionOnList(char c) { switch (c) { case 'a': case 'c': case 's': case 'r': case 'l': case 'b': case 'n': helper(c); break; case 'e': break; default: printf("Invalid input! "); } } // This function asks the user for information depending on what option the user selects from the menu and displays the expected result. // This function is already implemented completely. Read the function to understand how and what parameters are given as input to the functions that you will implement. // It is always helpful to understand how the code works before implementing new features. // Do not change anything in this function or you risk failing the automated test cases. void helper(char c) { if (c == 'a') { char bookName[100]; char libraryOfBook[30]; printf(" Please enter the following info of the new book. "); printf(" Book name: "); fgets(bookName, sizeof(bookName), stdin); bookName[strlen(bookName) - 1] = '\0'; // remove the trailing ' ' printf(" Library: "); fgets(libraryOfBook, sizeof(libraryOfBook), stdin); libraryOfBook[strlen(libraryOfBook) - 1] = '\0'; // remove the trailing ' ' struct book* result = searchBook(bookName); if (result == NULL) // add book if not already present in database { addBook(bookName, libraryOfBook); printf(" Book added to library database. "); } else printf(" That book is already in the database. "); } else if (c == 's' || c == 'r' || c == 'c' || c == 'l') { // actions which are common to commands s,r,c,l char bookName[30]; printf(" Please enter the book's name: "); fgets(bookName, sizeof(bookName), stdin); bookName[strlen(bookName) - 1] = '\0'; // remove the trailing ' ' struct book* result = searchBook(bookName); if (result == NULL) printf(" That book is not in the database. "); else if (c == 's') { printf(" Library: %s ", result->library); if(result->authors == NULL) printf("No author in database for this book. "); else { printf(" Authors: "); // modified code start struct author* tempAuthor = result->authors; while (tempAuthor != NULL) { printf("%s ", tempAuthor->name); tempAuthor = tempAuthor->next; } /* while(result->authors != NULL) { printf("%s ", result->authors->name); result->authors = result->authors->next; } */ // modified code end } } // command specific actions below else if (c == 'r') { removeBook(bookName); printf(" Book removed from the library database "); } else if (c == 'c') { char authorName[30]; printf(" Please enter the name of the author: "); fgets(authorName, sizeof(authorName), stdin); authorName[strlen(authorName) - 1] = '\0'; // remove the trailing ' ' addAuthor(bookName, authorName); printf(" Author added. "); } else if (c == 'l') { char* result = lastAuthor(bookName); if (result == NULL) printf(" No authors listed for this book. "); else printf(" Last author: %s ", result); } } else if (c == 'b') { char libraryName[30]; printf(" Please enter the library name: "); fgets(libraryName, sizeof(libraryName), stdin); libraryName[strlen(libraryName) - 1] = '\0'; // remove the trailing ' struct bookList* result = booksInThisLibrary(libraryName); printf(" List of books in %s library: ", libraryName); displayList(result); deleteDatabase(result); result = NULL; } else // c = 'n' { struct bookList* result = sortByName(); printf(" List of books sorted by name: "); displayList(result); deleteDatabase(result); result = NULL; } } // This function recursively removes all books from the linked list. // Notice that all of the authors for all of the books are removed as well // This function is already called at the right places. void deleteDatabase(struct bookList* books) { struct author* a1; if (books != NULL) { deleteDatabase(books->next); while (books->book->authors != NULL) { a1 = books->book->authors; books->book->authors = books->book->authors->next; free(a1); // delete authors linked list } free(books->book); free(books); // delete book linked list } } // This function prints the list of books for 'n: sort by name' and 'b: books in particular library' options. // It may be useful to trace this code before you get started void displayList(struct bookList* inputList) { if (inputList == NULL) { printf(" No books to list ! "); // check if list is empty return; } while (inputList != NULL) // traverse the list to display { printf(" Book name: %s", inputList->book->name); printf(" Library: %s ", inputList->book->library); printf("Authors: "); struct author* ptr = inputList->book->authors; if (ptr == NULL) // check if authors are added for the book { printf("No authors in database. "); } else { while (ptr != NULL) // traverse list of authors to display { printf("%s ", ptr->name); ptr = ptr->next; } } inputList = inputList->next; } } // a: Add a new book to the database (5 points) // This function should add book to the head of the linked list. // You can assume that the book is not already on the list. // The input parameters are book's name and library name. void addBook(char* name, char* library) { // Enter your code here } // c: Add an author for a book (10 points) // This function should add the author's name to the tail of the linked list of the book's authors. // Assume that the book is already on the book list and the new author's name is not on its authors list. // The input parameters are the book's name and the new author's name void addAuthor(char* bookName, char* authorName) { // Enter your code here } // s: Search for a book in the database (5 points) // This function should search for the book in the linked list. // If the book exists on the list, then return a pointer to the requested book (of type 'struct book*'). If not, return NULL. // Assume that no 2 books will have the same name. // The input parameter is the book's name struct book* searchBook(char* name) { // Enter your code here return NULL; // added this line to avoid compile error for empty function. // Remove this line when you code for this function. } // r: Remove a book from the database (15 points) // This function should remove the book using proper memory management to ensure no memory leaks. // Assume that the book exists on the list. // The input parameter is the book's name void removeBook(char* bookName) { // Enter your code here } // l: Display the last author added for a book (15 points) // This function returns the last author's name for that book, which will be at the tail of the linked list of that book's authors. // If the book does not have any authors, then return NULL. // Assume that the book is already on the list. // The input parameter is the book's name char* lastAuthor(char* bookName) { // Enter your code here return NULL; // added this line to avoid compile error for empty function. // Remove this line when you code for this function. } // b: Display the list of books available in a particular library (25 points) // This function is used to construct a new linked list from the global linked list 'list'. // The returned list should contain the books whose library name (bookList->book->library) matches with the input parameter libraryName. // No sorting is required for this list. // The list that you return will be cleaned up for you by the deleteDatabase() function (see helper() function). // Notice that the returned list will need to contain author information too. // This function should NOT modify the global linked list 'list'. struct bookList* booksInThisLibrary(char* libraryName) { // Enter your code here return NULL; // added this line to avoid compile error for empty function. // Remove this line when you code for this function. } // n: Display the list of books by name (25 points) // This function is used to construct a new linked list from the global linked list 'list'. // The returned list should be sorted alphabetically by book's name (bookList->book->name). // Notice that the returned list will need to contain all book and author information. // This function should NOT modify the global linked list. struct bookList* sortByName() { // Enter your code here return NULL; // added this line to avoid compile error for empty function. // Remove this line when you code for this function. }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
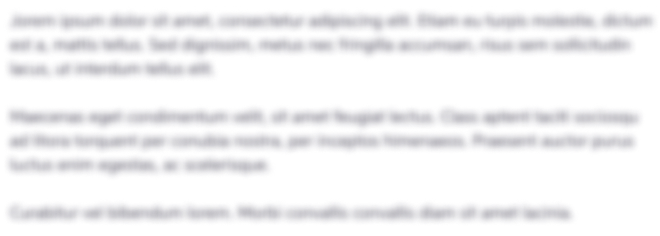
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started