Question
Here is the question: In Assignment 4, you created a Card class that represents a standard playing card. Use this to design and implement a
Here is the question:
-
In Assignment 4, you created a Card class that represents a standard playing card. Use this to design and implement a class called DeckOfCards that stores 52 objects of the Card class using an array. Include methods to shuffle the deck, deal a card, return the number of cards left in the deck, and a toString to show the contents of the deck. The shuffle methods should assume a full deck. Document your design with a UML Class diagram. Create a separate driver class that first outputs the populated deck to prove it is complete, shuffles the deck, and then deals each card from a shuffled deck, displaying each card as it is dealt along with the number of cards left in the deck.
Hint: The constructor for DeckOfCards should have nested for loops for the face values (1 to 13) within the suit values (1 to 4) calling the two parameter constructor. The shuffle method does not have to simulate how a deck is physically shuffled; you can achieve the same effect by repeatedly swapping pairs of cards chosen at random.
Testing: Include two complete runs to demonstrate the random effect of shuffling.
Here is my code:
// Assignment5Q2.java
import java.util.Random;
public class Assignment5Q2 { public static void main(String[] args) { //creating a Deck, displaying it DeckOfCards deck = new DeckOfCards();
System.out.println("Deck:"); System.out.println(deck);
System.out.println(" Shuffling deck and dealing each card "); //shuffling deck.shuffleDeck(); //looping until all cards have been dealt while (deck.getCardsLeft() > 0) { //dealing and displaying card System.out.println("--------------------------"); System.out.println("Dealt card: " + deck.dealACard()); System.out.println("Number of cards left: " + deck.getCardsLeft()); } } }
public class Card{ //class Card // public final static int HEARTS = 1; // public final static int DIAMONDS = 2; // public final static int CLUBS = 3; // public final static int SPADES = 4; private final static String[] faces={" ", "Ace", "Two", "Three", "Four", "Five" , "Six", "Seven", "Eight", "Nine", "Ten", "Jack" , "Queen", "King"}; private final static String[] suits={" ", "Hearts", "Clubs", "Diamonds", "Spades"};
private int suit; private int face;
// Default constructor public Card(){ Random rand = new Random(); // Assingning random values in the specified range face = rand.nextInt(13) + 1; suit = rand.nextInt(4) + 1; }
// Parameterised constructor public Card(int face, int suit){ // It sets the values only if the values are in the specified range if(face >= 1 && face <= 13) this.face = face; // else // throw new IllegalArgumentException("The face value should be in range [1 13].");
if(suit >= 1 && suit <= 4) this.suit = suit; // else // throw new IllegalArgumentException("The suit value should be in range [1 4]."); }
public int getFace(){ return face; }
// Returns the text of face public String getFaceText(){ return faces[face]; }
public int getSuit(){ return suit; }
public void setFace(int face){ if(face >= 1 && face <= 13) this.face = face; // else // throw new IllegalArgumentException("The face value should be in range [1 13]."); }
public void setSuit(int suit){ if(suit >= 1 && suit <= 4) this.suit = suit; // else // throw new IllegalArgumentException("The suit value should be in range [1 4]."); }
//toString method @Override public String toString(){ return faces[face] + " of " + suits[suit]; } }
public class DeckOfCards { // array to store cards in deck private Card[] deck; // number of cards remaining to deal private int cardsLeft; // constructor
public DeckOfCards() { // initializing array deck = new Card[52]; cardsLeft = 0; // using a nested loop to create all 4*13 cards, add to array for (int suit = 1; suit <= 4; suit++) { for (int face = 1; face <= 13; face++) { Card card = new Card(face, suit); deck[cardsLeft] = card; cardsLeft++; } } }
// method to shuffle the deck public void shuffleDeck() { // looping for half the size of deck for (int i = 0; i < deck.length * 10; i++) { // generating a random index between 0 and deck.length-1 int randIndex1 = (int) (Math.random() * deck.length); int randIndex2 = (int) (Math.random() * deck.length); // swapping elements at indices i and randIndex Card temp = deck[randIndex1]; deck[randIndex1] = deck[randIndex2]; deck[randIndex2] = temp; } }
// method to deal a card from shuffled deck public Card dealACard() { // returning null if no cards left if (cardsLeft == 0) { return null; }
// getting top card, updating cardsLeft, returning stored card Card c = deck[cardsLeft - 1]; cardsLeft--;
return c; }
// returns the number of cards left public int getCardsLeft() { return cardsLeft; }
// returns a String containing all cards info @Override public String toString() { String data = "";
// appending each card's info to a string and returning it for (int i = 0; i < cardsLeft; i++) { data += deck[i] + " "; }
return data.trim(); } }
Please fix any issues in my code or comment if it's all correct. Thank you.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
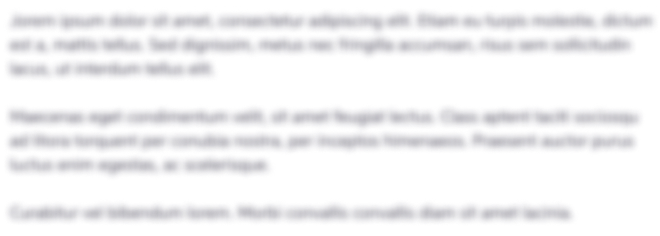
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started