Question
Hey, I need help with this assignment. Thanks. I have the code for the Ellipsoid class needed for the other parts. I am using JGrasp
Hey, I need help with this assignment. Thanks. I have the code for the Ellipsoid class needed for the other parts.
I am using JGrasp Ide for java.
import java.text.DecimalFormat; /** * Program that formualtes an ellipsoid. This program takes input from the user. * * Project 4. * @author david estrada - comp 1210 - section 008. * @version 02/05/2020. */ public class Ellipsoid { //Fields private String label = ""; private double a = 0; private double b = 0; private double c = 0; //constructor /** *@param labelIn establish the label *@param aIn establishes a axes. *@param bIn establishes b axes. *@param cIn establishes c axes. */ public Ellipsoid(String labelIn, double aIn, double bIn, double cIn) { setLabel(labelIn); setA(aIn); setB(bIn); setC(cIn); } //Methods /** *@return getLabel */ public String getLabel() { return label; } /** * * @param labelIn this retrieves a label. * @return setLabel */ public boolean setLabel(String labelIn) { if (labelIn == null) { return false; } else { label = labelIn.trim(); return true; } } /** * * @return getA */ public double getA() { return a; } /** * * @param aIn this returms a boolean. * @return setA */ public boolean setA(double aIn) { if (aIn > 0) { a = aIn; return true; } else { return false; } } /** * * @return getB */ public double getB() { return b; } /** * * @param bIn this returms a boolean. * @return setB */ public boolean setB(double bIn) { if (bIn > 0) { b = bIn; return true; } else { return false; } } /** * * @return getC */ public double getC() { return c; } /** * * @param cIn this returna a boolean. * @return setC */ public boolean setC(double cIn) { if (cIn > 0) { c = cIn; return true; } else { return false; } } /** * * @return volume */ public double volume() { double volume = ((4 * Math.PI * a * b * c) / 3); return volume; } /** * *@return surfaceArea */ public double surfaceArea() { double thePowers = (Math.pow((a * b), 1.6) + Math.pow((a * c), 1.6) + Math.pow((b * c), 1.6)) / 3; double surfaceArea = 4 * Math.PI * Math.pow(thePowers, (1.0 / 1.6)); return surfaceArea; } /** * * @return toString */ public String toString() { DecimalFormat decimalFormat = new DecimalFormat("#,##0.0###"); return "Ellipsoid \"" + getLabel() + "\" with axes a = " + decimalFormat.format(a) + ", b = " + decimalFormat.format(b) + ", c = " + decimalFormat.format(c) + " units has: \tvolume = " + decimalFormat.format(volume()) + " cubic units" + " \tsurface area = " + decimalFormat.format(surfaceArea()) + " square units"; } }
Project: Ellipsoid List App Page 4 of 7 EllipsoidList.java Requirements: Create an Ellipsoidlist class that stores the name of the list and an ArrayList of Ellipsoid objects. It also includes methods that return the name of the list, number of Ellipsoid objects in the Ellipsoidl.ist total volume, total surface area, average volume, and average surface for all Ellipsoid objects in the EllipsoidlistThe toString method returns a String containing the name of the list followed by cach Ellipsoid in the ArrayList, and a summary info method returns summary information about the list (see below) Design: The Ellipsoid List class has two fields, a constructor, and methods as outlined below. (1) Fields (or instance variables): (1) a String representing the name of the list and (2) an ArrayList of Ellipsoid objects. These are the only fields (or instance variables) that this class should have, and both should be private. (2) Constructor: Your Ellipsoidlist class must contain a constructor that accepts a parameter of type String representing the name of the list and a parameter of type ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
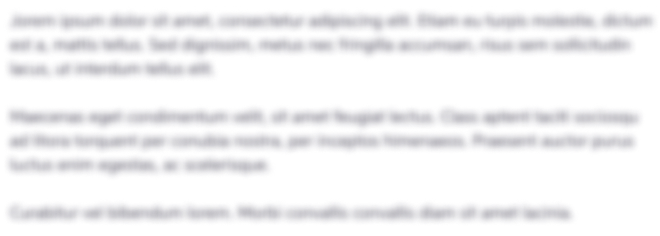
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started