Question
Hey please use Operator Overloading in these cpp files and vectors and print the vector.If possible( optional) try file handling where the price of products
Hey please use Operator Overloading in these cpp files and vectors and print the vector.If possible( optional) try file handling where the price of products would already be set there.
just add operator overloading and vector in those cpp files which are given below:
BasicDetails.h
#pragma once
#include
#include
#include
using namespace std;
class BasicDetails{
private:
int _serialNumber;
char _rowOfPlaceProduct;
int _ShelfOfProduct;
double _quantityOfProduct;
int _areaOfProductInTheStore;
protected:
int _typeOfProduct;
public:
BasicDetails();
void setSerialNumber();
int getSerialNumber()const { return this->_serialNumber; }
void setRowOfPlaceProduct();
char getRowOfPlaceProduct()const{ return this->_rowOfPlaceProduct; }
void setShelfOfProduct();
int getShelfOfProduct()const { return this->_ShelfOfProduct; }
void setQuantity();
double getQuantity() const{ return this->_quantityOfProduct; }
int getTypeOfProduct()const { return this->_typeOfProduct; }
void setTypeOfProduct();
void setAreaOfProductInTheStore();
int getAreaOfProductInTheStore() const { return this->_areaOfProductInTheStore; }
void changeQuantity();
//virtual methods
virtual double setPriceOfProduct(double num = 3.0) const; /
virtual void printProduct()const;
virtual ~BasicDetails();
};
BasicDetails.cpp
#include "BasicDetails.h"
BasicDetails::BasicDetails(){ //defualt c'tor set: serial,row,shelf,quantityand area
cout << "BasicDetails C'tor is work" << endl;
this->setSerialNumber();
this->setRowOfPlaceProduct();
this->setShelfOfProduct();
this->setQuantity();
this->setAreaOfProductInTheStore();
};
void BasicDetails::setRowOfPlaceProduct(){ //set row method
char row;
int flag = 0;
cout << "please select a row of the product from a to z (automatic a to A)" << endl;
do{
flag = 0;
cin >> row;
if (row >= 'a' && row <= 'z'){
row -= 32;
this->_rowOfPlaceProduct = row;
}
else if (row >= 'A' && row <= 'Z'){
this->_rowOfPlaceProduct = row;
}
else{
flag = 1;
cout << ("the row must be a letter from A to Z please select again") << endl;
}
} while (flag != 0);
}
void BasicDetails::setSerialNumber(){ //set serial number method
int flag = 0;
int serial;
cout << "Please select the serial number of the product" << endl;
do{
flag = 0;
cin >> serial;
if (cin.fail()){
flag = 1;
cin.clear();
cin.ignore();
cout << "the serial must be a positive number" << endl;
}
else if (serial <= 0){
flag = 1;
cout << "the serial must be a positive number" << endl;
}
else
this->_serialNumber = serial;
} while (flag != 0);
}
void BasicDetails::setShelfOfProduct(){ //set sheld method
int num;
int flag = 0;
cout << "please select the shelf of the product (1 to 5)"< do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the shel must be a integer number between 1 to 5 - please choose again" << endl; } else if (num < 1 || num > 5){ flag = 1; cout << "the shel must be a integer number between 1 to 5 - please choose again" << endl; } else this->_ShelfOfProduct = num; } while (flag != 0); } void BasicDetails::printProduct()const{ //print (virtual method) cout << this->getSerialNumber() << " " << this->getRowOfPlaceProduct() << " " << this->getShelfOfProduct() << "(" << this->getQuantity() << "," << this->getTypeOfProduct() << "," << this->getAreaOfProductInTheStore() << ") "; } void BasicDetails::setQuantity(){ //set quantity method int flag = 0; double num; cout << "please select the Quantity of the product" << endl; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the quantity must be a positive number please select again" << endl; } else if (num <= NULL){ flag = 1; cout << "the quantity must be a positive number please select again" << endl; } else this->_quantityOfProduct = num; } while (flag != 0); } void BasicDetails::changeQuantity(){ //option the change quantity method double num; int flag = 0; cout << "please select the quantity the you want to change" << endl; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the quantity must be positive number" << endl; } else if (num <= NULL){ flag = 1; cout << "the quantity must be positive number" << endl; } else this->_quantityOfProduct = num; } while (flag != 0); } void BasicDetails::setTypeOfProduct(){ //set typeOfProduct: Agricultural or milk or Package method int num; int flag = 0; cout << "please select the type of the product 1)Agricultural Product 2)Milk Product 3)Package Product" << endl; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the type must be a positive integer number between 1-3 please select again" << endl; } else if (num == 1 || num == 2 || num == 3) this->_typeOfProduct = num; else{ flag = 1; cout << "the type of the product must be 1 or 2 or 3 - please try again" << endl; } } while (flag != 0); } void BasicDetails::setAreaOfProductInTheStore(){ //set area method int num; int flag = 0; cout << "please select the Area of the product in the store: 1)High 2)Middle 3)Hide "; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); } else if (num == 1 || num == 2 || num == 3) this->_areaOfProductInTheStore = num; else{ flag = 1; cout << "the type of the product must be 1 or 2 or 3 - please select again" << endl; } } while (flag != 0); } double BasicDetails::setPriceOfProduct(double num) const{ //calculate price (virtual method) double factor = num; int flag = 0; if (this->getAreaOfProductInTheStore() == 3){ return (this->getQuantity() * 3 * factor); } else if (this->getAreaOfProductInTheStore() == 2){ return (this->getQuantity()*factor*1.5); } else if (this->getAreaOfProductInTheStore() == 1) return (this->getQuantity()*factor); return (factor*this->getQuantity()); //defualt } BasicDetails::~BasicDetails(){ //D'ctor (virtual) cout << "**the destractor of basic details is work**" << endl; } TheShop.h #include #pragma once #include "Windows.h" #include "Vegetable.h" #include "Fruit.h" #include "Cheese.h" #include "Yogurt.h" #include "Beverage.h" #include "OtherMilkProduct.h" #include "ProductPackage.h" #include "BasicDetails.h" using namespace std; class TheShop{ private: string _nameOfShop; double _factorOfShop; int _numOfProductsInTheShop; BasicDetails** _arrOfProducts; public: TheShop() void setNameOfShop(); string getNameOfShop()const { return this->_nameOfShop; } double getFactorOfShop() const { return this->_factorOfShop; } int getNumOfProductsInTheShop()const { return this->_numOfProductsInTheShop; } void manu(); void printAllPrices() const; void printDetailsOfShop() const; void changeFactorOfShop(); void addProductToarr(); bool checkSerialNumber(int serial) const; void calPriceOfAllProduct() const; }; TheShop.cpp #include "TheShop.h" TheShop::TheShop(){ //C'tor set: default name and factor this->_arrOfProducts = NULL; this->_numOfProductsInTheShop = NULL; this->_nameOfShop = "SuperShop"; this->_factorOfShop = 3; } void TheShop::calPriceOfAllProduct() const{ //calculat the price of all the products in the store method double sum=0; for (int i = 0; i sum += _arrOfProducts[i]->setPriceOfProduct(this->getFactorOfShop()); } cout << "************************" << endl; if (sum < NULL){ cout << "Total Price : the factor of shop must be suitable for this product" << endl; cout << "************************" << endl; } else{ cout << "Total Price : " << sum << endl; cout << "************************" << endl; } } void TheShop::printDetailsOfShop() const{ //print all the shop method cout << "the Name Of the Shop is: " << this->getNameOfShop() << endl; cout << "The numbers of the products in the shop is: " << this->getNumOfProductsInTheShop() << endl; cout << "the factor of the shop is : " << this->getFactorOfShop() << endl; cout << "the products that in store are:" << endl; if (this->getNumOfProductsInTheShop() == NULL) cout << "the shop is empty" << endl; else{ for (int i = 0; i < this->getNumOfProductsInTheShop(); i++) this->_arrOfProducts[i]->printProduct(); } cout << " "; } void TheShop::changeFactorOfShop(){ //change the factor of the shop method double factor; int flag = 0; cout << "please select the new factor of the shop" << endl; do{ flag = 0; cin >> factor; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the factor must be a positive number" << endl; } else if (factor <= NULL){ flag = 1; cout << "the factor must be a positive number" << endl; } else this->_factorOfShop = factor; } while (flag != 0); } void TheShop::printAllPrices() const{ //print all the prices in the shop method int flag = 0; if (this->getNumOfProductsInTheShop() == NULL){ cout << "************************" << endl; cout << "No products in the store" << endl; cout << "************************" << endl; } else{ flag = 1; cout << "************************" << endl; for (int i = 0; i < this->getNumOfProductsInTheShop(); i++){ double num = this->_arrOfProducts[i]->setPriceOfProduct(this->getFactorOfShop()); if (num < NULL) cout << "the price of product number " << i + 1 << " is: " << "the factor of shop must be suitable for this product " << endl; else cout << "the price of product number " << i + 1 << " is: " << this->_arrOfProducts[i]->setPriceOfProduct(this->getFactorOfShop()) << endl; } } if (flag == 1) cout << "************************" << endl; } void TheShop::addProductToarr(){ //add one product to the store int flag = 0; int choice; BasicDetails** temp = new BasicDetails*[this->getNumOfProductsInTheShop() + 1]; for (int i = 0; i < this->getNumOfProductsInTheShop(); i++) temp[i] = this->_arrOfProducts[i]; delete[] this->_arrOfProducts; cout << "Product Type : " << endl; cout << "0) Vegetable" << endl; cout << "1) Fruit" << endl; cout << "2) Cheese" << endl; cout << "3) Yogurt" << endl; cout << "4) Beverage" << endl; cout << "5) Other Milk Product" << endl; cout << "6) Product Package" << endl; cout << "your choice :"; do{ flag = 0; cin >> choice; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the num should be a integer positive number - please choose again" << endl; } else if (choice < 0 || choice > 6){ flag = 1; cout << "the number should be between 1 to 6" << endl; } } while (flag != 0); switch (choice) { case 0: temp[this->getNumOfProductsInTheShop()] = new Vegetable; break; case 1: temp[this->getNumOfProductsInTheShop()] = new Fruit; break; case 2: temp[this->getNumOfProductsInTheShop()] = new Cheese; break; case 3: temp[this->getNumOfProductsInTheShop()] = new Yogurt; break; case 4: temp[this->getNumOfProductsInTheShop()] = new Beverage; break; case 5: temp[this->getNumOfProductsInTheShop()] = new OtherMilkProduct; break; case 6: temp[this->getNumOfProductsInTheShop()] = new ProductPackage; break; default: cout << "Please try again" << endl; return; } this->_numOfProductsInTheShop++; this->_arrOfProducts = temp; if (this->checkSerialNumber(this->_arrOfProducts[this->getNumOfProductsInTheShop() - 1]->getSerialNumber())){ cout << "*********************************" << endl; cout << "This product is already exist! - the product has been deleted by system" << endl; cout << "*********************************" << endl; _arrOfProducts[this->getNumOfProductsInTheShop() - 1]->~BasicDetails(); delete[] _arrOfProducts[this->getNumOfProductsInTheShop() - 1]; this->_numOfProductsInTheShop--; } } bool TheShop::checkSerialNumber(int serial) const{ //check serial number method for (int i = 0; i < this->getNumOfProductsInTheShop() - 1; i++) if (this->_arrOfProducts[i]->getSerialNumber() == serial) return true; return false; } void TheShop::setNameOfShop(){ //set name of shop method string name; cout << "please select the new name of the shop" << endl; cin.ignore(); getline(cin, name); this->_nameOfShop = name; } void TheShop::manu(){ //the manu of the program int flag = 0; int choice; while (1) { cout << "..::::::::MENU::::::::.." << endl; cout << "Welcome To " << this->getNameOfShop() << " SupeMarket" << endl; cout << "1) change the name of the store" << endl; cout << "2) Add a new Product" << endl; cout << "3) Calc total prices" << endl; cout << "4) change factor of shop" << endl; cout << "5) Print all prices" << endl; cout << "6) Print all store" << endl; cout << "7) EXIT" << endl; cout << "Your choice : "; do{ flag = 0; cin >> choice; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the num should be a integer positive number - please choose again" << endl; } else if (choice < 0 || choice > 7){ flag = 1; cout << "the number should be between 1 to 6" << endl; } } while (flag != 0); switch (choice) { case 1: this->setNameOfShop(); break; case 2: this->addProductToarr(); break; case 3: this->calPriceOfAllProduct(); break; case 4: this->changeFactorOfShop(); break; case 5: this->printAllPrices(); break; case 6: this->printDetailsOfShop(); break; case 7: cout << "BYE BYE" << endl; return; default: cout << "Please try again" << endl; break; } } } //TheShop::~TheShop(){ //D'ctor // if (this->_arrOfProducts != NULL){ // for (int i = 0; i < this->getNumOfProductsInTheShop(); i++){ // delete[] this->_arrOfProducts[i]; // } // delete this->_arrOfProducts; // } //} AgriculturalProduct.h #pragma once #include "BasicDetails.h" class AgriculturalProduct : public BasicDetails{ //AgriculturalProduct "Haklai" class include: Name,Num of Seasons and name of suppliers (defualt type = 1) private: string _nameOfAgriculturalProduct; int _numOfYearSeasons; int _numOfSuppliers; protected: int _typeOfAgriculturalProduct; //two types : Fruit and Vegtable public: AgriculturalProduct(); //defualt C'tor void setTypeOfAgriculturalProduct(int num); //set and get methods int getTypeOfAgriculturalProduct() const { return this->_typeOfAgriculturalProduct; } void setNameOfAgriculturalProduct(); string getNameOfAgriculturalProduct() const { return this->_nameOfAgriculturalProduct; } void setNumOfSuppliers(); int getNumOfSuppliers() const { return this->_numOfSuppliers; } virtual double setPriceOfProduct(double num = 3.0) const; //default is 3 (price depends on a factor default factor is 3) void setNumOfYearSeasons(); int getNumOfYearSeasons() const {return this->_numOfYearSeasons; } virtual void printProduct()const; // virutal print method virtual ~AgriculturalProduct(); //virtual D'ctor }; AgriculturalProduct.cpp #include "AgriculturalProduct.h" AgriculturalProduct::AgriculturalProduct(){ //defualt C'tor Set type,name,numOfYearSeasons and numOfSuppliers cout << "AgriculturalProduct c'tor is work" << endl; this->_typeOfProduct = 1; this->setNameOfAgriculturalProduct(); this->setNumOfYearSeasons(); this->setNumOfSuppliers(); } void AgriculturalProduct::setNameOfAgriculturalProduct(){ //set name method string name; cout << "please select the name of the product" << endl; cin >> name; this->_nameOfAgriculturalProduct = name; } void AgriculturalProduct::setNumOfYearSeasons(){ //set num of year seasons int num; int flag = 0; cout << "please select the number of seasons of the product" << endl; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "please select a integer number between 1-4" << endl; } else if (num < 1 || num > 4){ flag = 1; cout << "please select a integer number between 1-4" << endl; } else this->_numOfYearSeasons = num; } while (flag != 0); } void AgriculturalProduct::setNumOfSuppliers(){ //set num of suppliers int num; int flag = 0; cout << "please select the num of suppliers" << endl; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the number of suppliers must be a positive integer number - please choose again" << endl; } else if (num <= NULL){ flag = 1; cout << "the number of suppliers must be a positive integer number - please choose again" << endl; } else this->_numOfSuppliers = num; } while (flag != 0); } double AgriculturalProduct::setPriceOfProduct(double num) const{ //calculate the price of the product return (BasicDetails::setPriceOfProduct(num) * 3 * (5 - this->getNumOfYearSeasons()) + (5 * this->getNumOfSuppliers()) + this->getTypeOfProduct()); } void AgriculturalProduct::printProduct()const{ BasicDetails::printProduct(); cout << this->getNameOfAgriculturalProduct() << "(" << this->getTypeOfAgriculturalProduct() << "," << this->getNumOfYearSeasons() << "," << this->getNumOfSuppliers() << ")" << endl; } AgriculturalProduct::~AgriculturalProduct(){ //virtual c'tor cout << "**the destractor of AgriculturalProduct is work**" << endl; } ProductPackage.h #pragma once #include "BasicDetails.h" class ProductPackage : public BasicDetails{ //productPackege Class include: everything that in BasicDetails class, numOfProducts,Names,numofcolors (defualt type = 3) private: int _numOfProducts; string * _namesOfProducts; double _numOfColors; public: ProductPackage(); //defualt C'tor //get and set methods void setNumOfProducts(); double getNumOfProducts()const { return this->_numOfProducts; } void setNamesOfProducts(); void printNamesOfProducts() const; void setNumOfColors(); double getNumOfColors() const { return this->_numOfColors; } //virtual methods virtual double setPriceOfProduct(double num = 3.0) const; //calculate price with a defualt factor (can be changed by user) virtual void printProduct()const; //print virtual ~ProductPackage(); //virtual D'ctor }; ProductPackage.cpp #pragma once #include "BasicDetails.h" class ProductPackage : public BasicDetails{ //productPackege Class include: everything that in BasicDetails class, numOfProducts,Names,numofcolors (defualt type = 3) private: int _numOfProducts; string * _namesOfProducts; double _numOfColors; public: ProductPackage(); //defualt C'tor //get and set methods void setNumOfProducts(); double getNumOfProducts()const { return this->_numOfProducts; } void setNamesOfProducts(); void printNamesOfProducts() const; void setNumOfColors(); double getNumOfColors() const { return this->_numOfColors; } //virtual methods virtual double setPriceOfProduct(double num = 3.0) const; //calculate price with a defualt factor (can be changed by user) virtual void printProduct()const; //print virtual ~ProductPackage(); //virtual D'ctor }; MilkProduct.h #pragma once #include "BasicDetails.h" class MilkProduct : public BasicDetails{ //milkProduct Class include: name,numofColors,NumOfFat (defualt type = 2) private: string _nameOfMilkProduct; double _numOfColorsInOneMilkProduct; double _numOfFat; protected: int _typeOfMilkProduct; public: MilkProduct(); //defualt C'tor int getTypeOfMilkProduct()const { return this->_typeOfMilkProduct; } //set and get methods void setNameOfMilkProduct(); string getNameOfMilkProduct()const { return this->_nameOfMilkProduct; } void setNumOfColorsInOneMilkProduct(); double getNumOfColorsInOneMilkProduct() const { return this->_numOfColorsInOneMilkProduct; } void setNumOfFat(); double getNumOfFat() const { return this->_numOfFat; } //virtual methods virtual void printProduct()const; //print virtual double setPriceOfProduct(double num = 3.0) const; //calculate price with a defualt factor (can be changed by user) virtual ~MilkProduct(); //virtual D'ctor }; MilkProduct.cpp #include "MilkProduct.h" MilkProduct::MilkProduct(){ //C'tor set: type,name,color and numOfFat cout << "MILK PRODUCT CONSTRACOT IS ON" << endl; this->_typeOfProduct = 3; this->setNameOfMilkProduct(); this->setNumOfColorsInOneMilkProduct(); this->setNumOfFat(); } void MilkProduct::setNameOfMilkProduct(){ //set name method string name; cout << "please select the name of the milk product" << endl; cin >> name; this->_nameOfMilkProduct = name; } void MilkProduct::setNumOfColorsInOneMilkProduct(){ //set num method int num; int flag = 0; cout << "please select the number of color in the milk product" << endl; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the color in the product should be a integer positive number - please choose again" << endl; } else if (num < NULL){ flag = 1; cout << "the color in the product should be a integer positive number - please choose again" << endl; } else this->_numOfColorsInOneMilkProduct = num; } while (flag != 0); } void MilkProduct::setNumOfFat(){ //set num of fat method double num; int flag = 0; cout << "please select the number of fat" << endl; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the num of fat shuold be a positive number - please choose again" << endl; } else if (num < NULL){ flag = 1; cout << "the num of fat shuold be a positive number - please choose again" << endl; } else this->_numOfFat = num; } while (flag != 0); } double MilkProduct::setPriceOfProduct(double num) const{ //calculate price (virtual method) return (((BasicDetails::setPriceOfProduct(num) + this->getNumOfColorsInOneMilkProduct()) - this->getNumOfFat()) * this->getTypeOfProduct()); } void MilkProduct::printProduct()const{ //print (virtual method) BasicDetails::printProduct(); cout << this->getNameOfMilkProduct() << " (" << this->getTypeOfMilkProduct() << "," << this->getNumOfColorsInOneMilkProduct() << "," << this->getNumOfFat() << ") "; } MilkProduct::~MilkProduct(){ //D'ctor (virtual) cout << "**the destractor of milk product is work**" << endl; } Bevarage.h #pragma once #include "MilkProduct.h" class Beverage : public MilkProduct //Beverage "Mashke" class include: Everything that in MilkProduct class { public: Beverage(); //defualt C'tor //virtual methods virtual double setPriceOfProduct(double num = 3.0) const { return (MilkProduct::setPriceOfProduct()); } //calculate price with a defualt factor (can be changed by user) depends on MilkProduct price virtual void printProduct() const { MilkProduct::printProduct(); cout << endl; } //virtual print method virtual ~Beverage(); //virtual D'ctor }; Bevarage.cpp #include "Beverage.h" Beverage::Beverage(){ //C'tor - set only the type this->_typeOfMilkProduct = 1; } Beverage::~Beverage() { } Cheese.h #pragma once #include "MilkProduct.h" class Cheese :public MilkProduct { //cheese Class "Gvina" include: Everything that in MilkProduct class,num of extra cheese private: int _numOfExtraCheese; public: Cheese(); //defualt C'tor void setNumOfExtraCheese(); //set and get methods int getNumOfExtraCheese()const { return this->_numOfExtraCheese; } //virtual mehods virtual void printProduct()const; //print virtual double setPriceOfProduct(double num = 3.0) const; //calculate price with a defualt factor (can be changed by user) virtual ~Cheese(); //virtual D'ctor }; Cheese.cpp #include "Cheese.h" Cheese::Cheese(){ // this->_typeOfMilkProduct = 3; this->setNumOfExtraCheese(); } void Cheese::setNumOfExtraCheese(){ //set numOfExtraCheese method int num; int flag = 0; cout << "please select number of extra" << endl; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the num of extra cheese should be a integer positive number - please choose again" << endl; } else if (num < NULL) { flag = 1; cout << "the num of extra cheese should be a integer positive number - please choose again" << endl; } else this->_numOfExtraCheese = num; } while (flag != 0); } double Cheese::setPriceOfProduct(double num) const{ //calculate price (virtual method) return (MilkProduct::setPriceOfProduct(num) + this->getNumOfExtraCheese()); } void Cheese::printProduct()const{ //print (virtual method) MilkProduct::printProduct(); cout << "(" << this->getNumOfExtraCheese() << ")" << endl; } Cheese::~Cheese(){ // D'ctor (virtual) cout << "**the destractor of cheese is work**" << endl; } Fruit.h #pragma once #include "AgriculturalProduct.h" class Fruit: public AgriculturalProduct{ //fruit class include: everything that in agriculturalProduct class, amount of sugar private: double _amountOfSugar; public: Fruit(); //defualt C'tor void setAmountOfSugar(); //get and set methods double getAmountOfSugar()const { return this->_amountOfSugar; } //virtual methods virtual void printProduct()const; //print virtual double setPriceOfProduct(double num = 3.0) const; //calculate price with a defualt factor (can be changed by user) virtual ~Fruit(); //virtual D'ctor }; Fruit.cpp #include "Fruit.h" Fruit::Fruit(){ // cout << "the c'tor of fruit is on" << endl; this->_typeOfAgriculturalProduct = 2; this->setAmountOfSugar(); } void Fruit::setAmountOfSugar(){ //set amount of sugar method double num; int flag = 0; cout << "please select the amount of suger in the fruit - (per 100 gram)" << endl; do{ flag = 0; cin >> num; if (cin.fail()){ flag = 1; cin.clear(); cin.ignore(); cout << "the num must be a positive number (not include NULL)" << endl; } else if (num < NULL){ flag = 1; cout << "the num must be a positive number (not include NULL)" << endl; } else{ double amount = (num / 100); this->_amountOfSugar = amount; } } while (flag != 0); } double Fruit::setPriceOfProduct(double num) const{ //calculate price (virtual method) return (AgriculturalProduct::setPriceOfProduct(num) + (this->getAmountOfSugar() / 2)); } void Fruit::printProduct()const{ //print (virtual method) BasicDetails::printProduct(); cout << this->getNameOfAgriculturalProduct() << "(" << this->getTypeOfAgriculturalProduct() << "," << this->getNumOfYearSeasons() << "," << this->getNumOfSuppliers() << ")" << "(" << this->getAmountOfSugar() << ")" << endl; } Fruit::~Fruit(){ //C'tor (virtual) cout << "**the destractor of fruit is work**" << endl; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
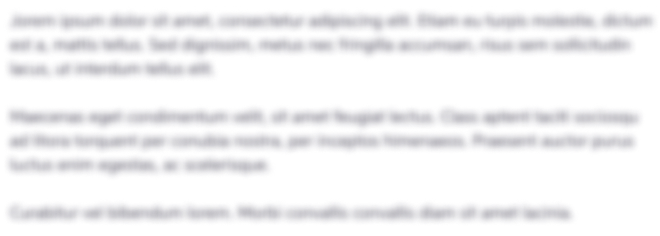
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started