Question
Hi, I have a program to do in JAVA on intelliJIDEA. Here are the instructions and the files necessary. Final operation of the application: The
Hi, I have a program to do in JAVA on intelliJIDEA. Here are the instructions and the files necessary.
Final operation of the application: The application should read and process the instructions defined in "instructions."
Instructions: buy-hero Instruction format: Hero name {string} Category (common=0, uncommon=1, rare=2, epic=3, legendary=4) {int} Cost in gold {double} Cost in armor {int} Health points {double}
buy-armor Instruction format: Number of armor pieces purchased {int} Price per armor piece {int}
do-quest Instruction format: Category (common=0, uncommon=1, rare=2, epic=3, legendary=4) {int} Cost in health points {double} Reward in gold {int} Reward in armor pieces {int}
train-hero Instruction format: Hero name {string}
Overview: The program must be executed with commands passed as parameters, i.e., args. In addition, multiple types of commands can be passed with several variables. The program must recognize the difficulty level of the quest and send the appropriate hero. Finally, the program must allow for the improvement of heroes in exchange for armor and gold.
Here's an example of usage:
buy-hero:Arthur,3,50.19,5,10.5 buy-armor:2,5 do-quest:2,1.5,6,3 train-hero:Arthur
A quest can only be solved by a hero of the same level. If this criterion is not met, the next most powerful hero is chosen. So, if there is no level 3 hero, we take the next level 4 hero. Note that the parameters in the commands are separated by commas.
When buying a hero, certain constraints must be met, such as the cost in gold and armor.
Buying armor is a critical criterion. Without successfully completing a quest, there would be no other means of obtaining armor. The price of armor per unit will be one of the parameters that the program must manage.
The program must accept the quests passed to it. In addition, the level of the heroes sent must be the same as the quest. If there are heroes that meet this criterion, they must be sent first. If there are no heroes of the same level, then a hero who follows the level in rank can be used. The program must sort through a list of heroes.
Furthermore, every time a hero is sent on a quest, they lose some of their health points. If they fall below or equal to zero, the quest fails, and the hero is removed from the list.
When upgrading a hero, the program must search for it based on the ID provided as a parameter. If the hero in question does not exist, an error message must be returned to the user. Afterward, it must be verified that there is enough gold and armor in inventory to upgrade the hero; otherwise, an error message must be returned to the user.
Program structure: Before starting to program, you will need to set up the structure of your program. For this task, we will give you more freedom in the structure of your program. Why? Because it is important that you are able to write structured code yourself.
We only ask that you have a few files and a few functions. You must submit these .java files for your submission:
A file for your starting code (Main.java) A management file that manages inventory (Bank) Two management files for commands (GuildCommand.java & GuildCommandSystem.java) One file for each hero level A file that allows for the launching of quests (Quete.java) A file that gathers all information related to the guild (Guild.java)
Note that your classes should not be heavy if you subdivide your program well.
Operation: When starting the program, we do so with arguments as parameters (args).
The Program's Final Functioning The application should read and process the instructions defined in "instructions".
Instructions: buy-hero Instruction format: Hero name {string} Category (common=0, uncommon=1, rare=2, epic=3, legendary=4) {int} Cost in money {double} Cost in armor {int} Health points {double}
buy-armor Instruction format: Number of armors purchased {int} Price per armor {int}
do-quest Instruction format: Category (common=0, uncommon=1, rare=2, epic=3, legendary=4) {int} Cost in health points {double} Reward in money {int} Reward in armor {int}
train-hero Instruction format: Hero name {string}
Overview: From the beginning, the program must be executed with commands passed as parameters, i.e., args. Moreover, multiple types of commands can be passed with several variables. The program must recognize the difficulty level of the quest and send the appropriate hero. Finally, the program must allow the improvement of heroes in exchange for armor and money.
Here is an example of usage:
buy-hero:Arthur,3,50.19,5,10.5 buy-armor:2,5 do-quest:2,1.5,6,3 train-hero:Arthur
A quest can only be solved by a hero of the same level. If this criterion is not met, then the next most powerful hero is chosen. So if you don't have a level 3 hero, then you take the level 4 hero next. Note that the parameters in the commands are separated by commas.
When buying a hero, certain constraints must be met, such as the cost in money and armor.
Buying armor is a crucial criterion. Indeed, unless a quest has been successfully completed, there would be no other means of acquiring it. The price per unit of armor will be one of the parameters that the program must manage.
The program must necessarily accept the quests that are passed to it. Moreover, the level of the heroes sent must be the same as that of the quest. If there are heroes that meet this criterion, they must be sent first. If there is no hero of the same level, then a hero that follows the level in rank can be found. The program must therefore sort through a list of heroes.
Furthermore, each time a hero is sent on a quest, he loses some of his health points. If he falls below or equal to zero, the quest fails and the hero is removed from the list.
When upgrading a hero, the program must look for him according to the ID we put as a parameter. If the hero in question does not exist, then an error message is returned to notify the user. Then we must check that we have enough money and armor in inventory to be able to upgrade it, otherwise an error message is returned to notify the user.
Program Structure Before starting to program, you will need to set up the structure of your program. For this assignment, we will give you more freedom in the structure of your program. Why? Because it is important that you be able to write structured code yourself.
We ask you only to have a few files and a few functions. You will need to submit these .java files for your submission:
A file for your starting code (Main.java) A management file that manages the inventory (Bank) Two management files for commands (GuildCommand.java & GuildCommandSystem.java) One file for each hero level One file for launching quests (Quete.java) One file that brings together all information related to the guild (Guild.java)
Note that your classes should not be heavy if you subdivide your program well.
Operation
When starting the program, it is done with arguments as parameters (args). These arguments are automatically put into an array. The program must take each of these commands and execute them with the expected result.
Example: Example 1
Input: guild:100.0,10 buy-hero:Berserker,2,52.5,6,30.5 do-quest:2,5.3,60,3 Output: Guild Bank account: 107.5 gold & 7 armours Heroes: -Berserker: level=2, HP=25.2
Example 2
Input: guild:100.0,10 buy-hero:Berserker,2,52.5,6,30.5 buy-hero:Zorro,1,36.2,2,15.0 do-quest:2,5.3,60,3 train-hero:Zorro Output: Guild Bank account: 23.3 gold & 2 armours Heroes: -Berserker: level=2, HP=25.2 -Zorro: level=2 HP=22.5
Example 3
Input: guild:100.0,10 buy-hero:Rogue,3,73.5,7,40.7 train-hero:Rogue do-quest:2,5.3,60,3 train-hero:Zorro Output: Guild Bank account: 86.5 gold & 6 armours Heroes: -Rogue: level=3, HP=36.9 Error: -You are lacking money and/or armor to upgrade Rogue. -The hero named Zorro does not appear in the list.
To calculate the price for an upgrade, the following formula should be used: 20ln(level + 10) A similar calculation is performed for armor where the "roof" is calculated as: ln(level + 10) To calculate a hero's HP, the formula is: maxHP1.5, which allows the hero to regain points of life. Finally, to calculate the lost HP if the hero does not correspond to the level of the quest, the formula is: lost_HP - (current_level - original_level) * 1.5.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
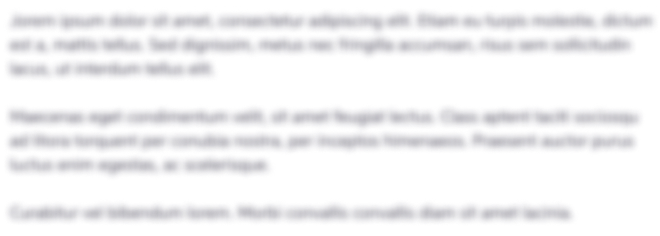
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started