Question
Hi, I'm working on this assignment, and I managed to complete the code, but the second part calls for a pseudocode, which is not a
Hi, I'm working on this assignment, and I managed to complete the code, but the second part calls for a pseudocode, which is not a strong suit for me. Can you look at what I have, tell if it's clear, and explain what's happening?
I have my code and pseudocode below:
My code:
#include
#include
#include
#include "CSVparser.hpp"
using namespace std;
//============================================================================
// Global definitions visible to all methods and classes
//============================================================================
// forward declarations
double strToDouble(string str, char ch);
// define a structure to hold bid information
struct Bid {
string bidId; // unique identifier
string title;
string fund;
double amount;
Bid() {
amount = 0.0;
}
};
//============================================================================
// Static methods used for testing
//============================================================================
/**
* Display the bid information to the console (std::out)
*
* @param bid struct containing the bid info
*/
void displayBid(Bid bid) {
cout << bid.bidId << ": " << bid.title << " | " << bid.amount << " | "
<< bid.fund << endl;
return;
}
/**
* Prompt user for bid information using console (std::in)
*
* @return Bid struct containing the bid info
*/
Bid getBid() {
Bid bid;
cout << "Enter Id: ";
cin.ignore();
getline(cin, bid.bidId);
cout << "Enter title: ";
getline(cin, bid.title);
cout << "Enter fund: ";
cin >> bid.fund;
cout << "Enter amount: ";
cin.ignore();
string strAmount;
getline(cin, strAmount);
bid.amount = strToDouble(strAmount, '$');
return bid;
}
/**
* Load a CSV file containing bids into a container
*
* @param csvPath the path to the CSV file to load
* @return a container holding all the bids read
*/
vector
cout << "Loading CSV file " << csvPath << endl;
// Define a vector data structure to hold a collection of bids.
vector
// initialize the CSV Parser using the given path
csv::Parser file = csv::Parser(csvPath);
try {
// loop to read rows of a CSV file
for (int i = 0; i < file.rowCount(); i++) {
// Create a data structure and add to the collection of bids
Bid bid;
bid.bidId = file[i][1];
bid.title = file[i][0];
bid.fund = file[i][8];
bid.amount = strToDouble(file[i][4], '$');
//cout << "Item: " << bid.title << ", Fund: " << bid.fund << ", Amount: " << bid.amount << endl;
// push this bid to the end
bids.push_back(bid);
}
} catch (csv::Error &e) {
std::cerr << e.what() << std::endl;
}
return bids;
}
/**
* Partition the vector of bids into two parts, low and high
*
* @param bids Address of the vector
* @param begin Beginning index to partition
* @param end Ending index to partition
*/
int partition(vector
// Initialize low and high indices
int low = begin, high = end;
// Calculate the midpoint to choose the pivot
int mid = begin + (end - begin) / 2;
string pivot = bids[mid].title; // Pivot is the title of the bid at the midpoint
// Continue partitioning until low and high indices meet
while (low <= high) {
// Move low index to the right while bid title is less than pivot
while (bids[low].title < pivot) {
++low;
}
// Move high index to the left while bid title is greater than pivot
while (bids[high].title > pivot) {
--high;
}
// If low and high indices have not crossed
if (low <= high) {
// Swap the bids at low and high indices
swap(bids[low], bids[high]);
// Move low and high indices closer
++low;
--high;
}
}
// Return the index where partitioning is complete
return high;
}
/**
* Perform a quick sort on bid title
* Average performance: O(n log(n))
* Worst case performance O(n^2))
*
* @param bids address of the vector
* @param begin the beginning index to sort on
* @param end the ending index to sort on
*/
void quickSort(vector
//set mid equal to 0
int mid = 0;
/* Base case: If there are 1 or zero bids to sort,
partition is already sorted otherwise if begin is greater
than or equal to end then return*/
if (begin >= end) {
return;
}
/* Partition bids into low and high such that
midpoint is location of last element in low */
mid = partition(bids, begin, end);
// recursively sort low partition (begin to mid)
quickSort(bids, begin, mid);
// recursively sort high partition (mid+1 to end)
quickSort(bids, mid + 1, end);
}
/**
* Perform a selection sort on bid title
* Average performance: O(n^2))
* Worst case performance O(n^2))
*
* @param bid address of the vector
* instance to be sorted
*/
void selectionSort(vector
//define min as int (index of the current minimum bid)
int min;
// check size of bids vector
// set size_t platform-neutral result equal to bid.size()
size_t pos = bids.size();
// pos is the position within bids that divides sorted/unsorted
// for size_t pos = 0 and less than size -1
for (pos = 0; pos < bids.size() - 1; ++pos) {
// set min = pos
min = pos;
// loop over remaining elements to the right of position
for (unsigned int i = pos + 1; i < bids.size(); ++i) {
// if this element's title is less than minimum title
if (bids.at(i).title < bids.at(pos).title) {
// this element becomes the minimum
min = i;
// swap the current minimum with smaller one found
// swap is a built in vector method
swap(bids.at(pos), bids.at(min));
}
}
}
}
/**
* Simple C function to convert a string to a double
* after stripping out unwanted char
*
* credit: http://stackoverflow.com/a/24875936
*
* @param ch The character to strip out
*/
double strToDouble(string str, char ch) {
str.erase(remove(str.begin(), str.end(), ch), str.end());
return atof(str.c_str());
}
/**
* The one and only main() method
*/
int main(int argc, char* argv[]) {
// process command line arguments
string csvPath;
switch (argc) {
case 2:
csvPath = argv[1];
break;
default:
csvPath = "eBid_Monthly_Sales.csv";
}
// Define a vector to hold all the bids
vector
// Define a timer variable
clock_t ticks;
int choice = 0;
while (choice != 9) {
cout << "Menu:" << endl;
cout << " 1. Load Bids" << endl;
cout << " 2. Display All Bids" << endl;
cout << " 3. Selection Sort All Bids" << endl;
cout << " 4. Quick Sort All Bids" << endl;
cout << " 9. Exit" << endl;
cout << "Enter choice: ";
cin >> choice;
switch (choice) {
case 1:
// Initialize a timer variable before loading bids
ticks = clock();
// Complete the method call to load the bids
bids = loadBids(csvPath);
cout << bids.size() << " bids read" << endl;
// Calculate elapsed time and display result
ticks = clock() - ticks; // current clock ticks minus starting clock ticks
cout << "time: " << ticks << " clock ticks" << endl;
cout << "time: " << ticks * 1.0 / CLOCKS_PER_SEC << " seconds" << endl;
break;
case 2:
// Loop and display the bids read
for (int i = 0; i < bids.size(); ++i) {
displayBid(bids[i]);
}
cout << endl;
break;
case 3:
//Initialize timer variable
ticks = clock();
//Call selectionSort() method
selectionSort(bids);
// Calculate elapsed time
ticks = clock() - ticks;
//Print results
cout << bids.size() << " bids sorted" << endl;
cout << "time: " << ticks << " clock ticks" << endl;
cout << "time: " << ticks * 1.0 / CLOCKS_PER_SEC << " seconds" << endl;
// Note: The reported time may differ from the expected value in the assignment.
break;
case 4:
//Initialize timer variable
ticks = clock();
//Call quickSort() method
quickSort(bids, 0, bids.size() - 1);
// Calculate elapsed time
ticks = clock() - ticks;
//Print results
cout << bids.size() << " bids sorted" << endl;
cout << "time: " << ticks << " clock ticks" << endl;
cout << "time: " << ticks * 1.0 / CLOCKS_PER_SEC << " seconds" << endl;
break;
// Note: The reported time may differ from the expected value in the assignment.
}
}
cout << "Good bye." << endl;
return 0;
}
Pseudocode:
Start
Input CSV Path
if CSV path provided
Set csvPath
Initialize bids vector
Display Menu
Get User Choice
Switch (User Choice)
Case 1: Load Bids
Initialize Timer
Call loadBids
Display Number of Bids
Calculate Elapsed Time
Case 2: Display All Bids
Loop through bids vector
Display Each Bid
Case 3: Selection Sort
Initialize Timer
Call selectionSort
Display Sorted Bids
Calculate Elapsed Time
Case 4: Quick Sort
Initialize Timer
Call quickSort
Display Sorted Bids
Calculate Elapsed Time
Case 9: Exit
Display 'Good bye'
End
End
Step by Step Solution
There are 3 Steps involved in it
Step: 1
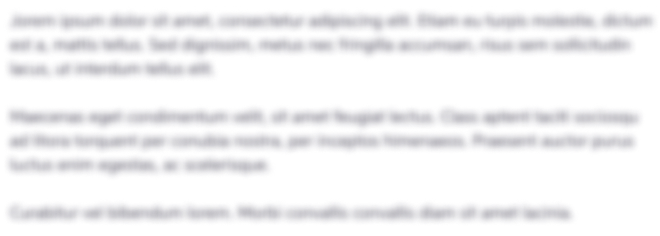
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started