Question
hi, need help for this project. Objectives: Gain practice writing simple methods that return a value. Gain practice writing complete classes, including instance variables, constructors
hi, need help for this project.
Objectives:
Gain practice writing simple methods that return a value.
Gain practice writing complete classes, including instance variables, constructors and query and update methods.
Write a program consisting of a supplier class and a client class.
You have been hired by a local Flower Shop to write a program that maintains a record of inventory items and total sales. Your program will report this information on request and will update it as flower orders are processed.
Specification: The flower shop sells only four (4) base items, but also has two (2) special combinations (there is no price break for combinations -- they just make placing an order easier). Here's a summary
a rose costs $1.99 | a carnationcosts $1.49 | a daisy costs $0.49 | a vase costs $3.99 |
Spring Special consists of 3 roses 6 carnations 4 daisies in a vase | Dozen Roses consists of 12 roses |
The flower shop also gives a free packet of flower fertilizer for each 12 flowers in a purchase. For example, if a purchase contains 2 flowers, they get 0 packets. If a purchase contains 12 flowers, they get 1 packet. If a purchase contains 13 flowers, they still get one packet.
Your program will consist of 2 classes:
a supplier class called Order that contains all the information about an order to be placed at the flower shop. Specifically, the Order class is made up of:
Four (4) instance variables called rose, carnation, daisy and vase, which contain the numbers of each of these items in the order.
Four (4) query methods, each of which returns the value of the corresponding instance variable. For example, a method called getCarnationCount( ) returns the number of carnations in the order.
Three (3) constructors, defined in the javadoc for this class. The different constructors put the order together in different ways, but the resulting order should always contain the desired numbers of each item.
a client class called FlowerShop that contains and maintains important information about the flower shop, including an inventory of critical items and a record of total sales. The Florist class is made up of:
Four (4) class constants of type double that store the unit price of each base product. For example,costOfDaisy = 0.49
Six (6) instance variables: one for each of the four items in inventory (roses, carnations, daisies, andvases), one for fertilizer packets, one for totalSales.
Two (2) query methods: getTotalSales( ) and printInventory( ).
One (1) update method, processOrder( ).
One (1) test method, which is provided in starter file. The test method calls each of the other methods (directly or indirectly) and provides one way of testing your code. Do not make any changes to the test method!
Additional Resources Here is a starter file for class FlowerShop.
View complete documentation for both classes.
Here are simplified class specifications for both classes (class constants not shown in the FlowerShopclass):
Order | FlowerShop |
- int vase - int rose - int carnation - int daisy | - int stockVases - int stockRoses - int stockCarnations - int stockDaisies - int stockFertilizer - double totalSales |
+ Order( ) + Order( int SpecialCount, int DozenCount ) + Order( int roseCount, int carnationCount, int DaisyCount, int vaseCount ) + int getRoseCount( ) + int getCarnationCount( ) + int getDaisyCount( ) + int getVaseCount( ) | + Florist( ) + double getTotalSales( ) + void printInventory + double processOrder( Order request ) + void test( ) |
Suggestions:
Develop the supplier class ( Order ) first and test it thoroughly using BlueJ to construct instances of the class and exercise the methods. Once this is working...
Develop the client class, then test it by using BlueJ to create several orders and "place" them at the flower shop. Determine in advance how each order should impact the inventory and total sales and make sure that everything is working properly.
Don't forget to document your classes properly. Be sure to use descriptive names for variables and constants and include javadoc comments in your code. The javadocs BlueJ generates should resemble those referenced in assignment above. (Use the 'Generate Documentation' menu selection in BlueJ, not the 'Interface')
Documentation:
Use javadoc comments before each class definition and before each method definition. Use @author, @version, @param and @return tags where appropriate. Refer to the Javadoc Basics help file for more info.
Include simple in-line comments where appropriate to set off sections of code and to explain why you are doing something that is not obvious.
After your program is complete, select Tools/ProjectDocumentation within BlueJ to create the .html pages. These will be placed in a folder called doc inside your project folder.
Grading:
/10 - program compiles and produces correct result from different test /4 - uses all correct instance variables and methods with correct signatures /2 - uses descriptive variable names and named constants /4 - // comments and javadocs are complete
this is addition website and codes:
http://facweb.northseattle.edu/dschaffe/CSC142/HW4/HW4-FlowerShop_doc/
/** * The FlowerShop class maintains inventory and total sales records * for a flower shop and also processes orders and requests * for reports. * * @author CSC 142 * @version 1.0 */ public class FlowerShop {
// add class constants
// add instance variables
// add constructors
// add QUERY methods
// add UPDATE method
// be sure to use Javadoc comments in all methods!
// TEST method
/** * This test method generates 3 orders, one using each of the * different constructors, then "places" the orders. One of * three return values from processOrder is printed. Finally, * an inventory report is requested and then the total sales are * checked using getTotalSales and printed. * */ public void test() { final String dashes = "---------------------------"; Order order1 = new Order(); Order order2 = new Order( 2, 3 ); Order order3 = new Order( 1, 3, 5, 8 ); processOrder( order3 ); processOrder( order2 ); double price = processOrder( order1 ); // use return value System.out.println( dashes ); System.out.println( "Price for one Spring Special = " + price ); System.out.println( dashes ); System.out.println( "Contents of order #3:" ); System.out.println( order3.getVaseCount() + " vases" ); System.out.println( order3.getRoseCount() + " roses" ); System.out.println( order3.getCarnationCount() + " carnations" ); System.out.println( order3.getDaisyCount() + " daisies" ); System.out.println( dashes ); printInventory(); double balance = getTotalSales(); System.out.println( dashes ); System.out.println( "Total sales = " + balance ); System.out.println( dashes ); }
}
that all I have.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
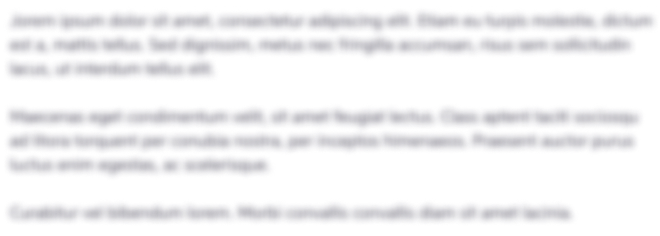
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started