Question
Higher Number Types There are many types of numbers beyond the naturals, integers, rationals, reals, and irrationals. You may have heard of imaginary numbers. You
Higher Number Types
There are many types of numbers beyond the naturals, integers, rationals, reals, and irrationals. You may have heard of imaginary numbers. You may not be familiar with complex numbers and hypercomplex numbers. The hypercomplex numbers include some extensions including quaternions, octonions, sedenions, tessarines, coquaternions, and biquaternions.
In this programming assignment, you will develop a package called HigherNumbers. Your package will include two classes, a ComplexNumber class and a Quaternion class.
Complex Numbers
A complex number has the form realPart + imaginaryPart * i, where realPart and imaginaryPart are real numbers and i has the value A complex number is written as (realPart, imaginaryParti). For example, a complex number with realPart 2.00 and imaginaryPart 5.00 is written (2.00, 5.00i).
To add two complex numbers, we add the two realParts and the two imaginaryParts; subtraction is done similarly. The product of two complex numbers (a, bi) and (c, di) is ((ac bd), (bc + ad)i). (See examples below.)
Design a class called ComplexNumber. Your class should have the following methods:
- A default constructor that sets the realPart and the imaginaryPart both to 0 (both of type double)
- A constructor that takes parameters for the realPart and the imaginaryPart
- Get methods for the realPart and the imaginaryPart
- A method toString that returns the string representation of a ComplexNumber. (format each number with exactly 2 digits)
- A method add that takes a ComplexNumber as a parameter which is added to the current object
- A method subtract that takes a ComplexNumber as a parameter that is subtracted from the current object
- A method multiply that takes a ComplexNumber as a parameter which the current object is multiplied by
- A method isEqual that takes a ComplexNumber as a parameter and determines whether it is equal in value to the current object. They are equal if their realParts and imaginaryParts are exactly equal.
Quaternions
A quaternion has the form a + b * i + c * j + d * k, where a, b, c, and d are real numbers, and each of i, j, k have the value . A quaternion is written as (a + bi + cj + dk). For example, a quarternion with a = 5.63, b = 2.78, c = 1.99, d = 3.49 is written (5.63 + 2.78i + 1.99j + 3.49k).
Design a class called Quaternion. Your class should have the following methods:
- A default constructor that sets a, b, c, and d all to 0 (all of type double)
- A constructor that takes parameters for a, b, c, and d
- Get methods for a, b, c, and d
- A method toString that returns the string representation of a Quaternion. (format each number with exactly 2 digits)
- A method add that takes a Quaternion as a parameter which is added to the current object
- A method subtract that takes a Quaternion as a parameter that is subtracted from the current object
- A method multiply that takes a Quaternion as a parameter which the current object is multiplied by
- A method isEqual that takes a Quaternion as a parameter and determines whether it is equal in value to the current object. They are equal if their corresponding a, b, c, and d are exactly equal.
Your program must run with the exact driver program on the next page. Note that there are no user prompts. The input is in a file of type .txt and is provided here right after the main program. Run the program from the command line using input redirection. If your main program is HigherNumberTester.java and the input file is named highernums.text, after you have compiled your program, type java HigherNumberTester< highernums.txt > testout.txt from the command line. This will read input from highernums.txt and put the output in testout.txt. The expected output is shown right after the input file.
import HigherNumbers.*;
import java.util.Scanner;
/**
* This is a main program to test the HigherNumbers package.
*/
public class HigherNumberTester
{
/**
* The main program. It will read in 5 pairs of
* complex numbers c1 and c2, and for each pair will tell:
* - whether or not they are equal
* - add c2 to c1 and report the sum
* - subtract c2 from c1 and report the difference (should be
* the original value of c1
* - multiply c1 by c2 and report the product
*
* Then it will read in 5 pairs of quaternions q1 and q2,
* and for each pair will tell:
* - whether or not they are equal
* - multiply q1 by q2 and report the product
*/
public static void main(String args[])
{
Scanner in = new Scanner (System.in);
double r, i, i2, a, b, c, d;
ComplexNumber c1, c2;
Quaternion q1, q2;
System.out.println ("Testing complex numbers");
for (int count = 0; count < 5; count++) {
r = in.nextDouble();
i = in.nextDouble();
c1 = new ComplexNumber(r, i);
r = in.nextDouble();
i = in.nextDouble();
c2 = new ComplexNumber(r, i);
if (c1.isEqual(c2)) {
System.out.println (c1.toString() + " is equal to " + c2.toString());
}
else {
System.out.println (c1.toString() + " is not equal to " + c2.toString());
}
System.out.println ("c1 is " + c1.toString());
c1.add(c2);
System.out.println ("After adding " + c2.toString() + ", c1 is now "
+ c1.toString());
c1.subtract(c2);
System.out.println ("After subtracting " + c2.toString() + ", c1 is now "
+ c1.toString());
c1.multiply(c2);
System.out.println ("After multiplying by " + c2.toString() + ", c1 is now "
+ c1.toString());
}
System.out.println ("Testing quaternions");
for (int count = 0; count < 5; count++) {
a = in.nextDouble();
b = in.nextDouble();
c = in.nextDouble();
d = in.nextDouble();
q1 = new Quaternion (a, b, c, d);
a = in.nextDouble();
b = in.nextDouble();
c = in.nextDouble();
d = in.nextDouble();
q2 = new Quaternion (a, b, c, d);
if (q1.isEqual(q2)) {
System.out.println (q1.toString() + " is equal to " + q2.toString());
}
else {
System.out.println (q1.toString() + " is not equal to " + q2.toString());
}
System.out.println ("q1 is " + q1.toString());
q1.add(q2);
System.out.println ("After adding " + q2.toString() + ", q1 is now "
+ q1.toString());
q1.subtract(q2);
System.out.println ("After subtracting " + q2.toString() + ", q1 is now "
+ q1.toString());
q1.multiply(q2);
System.out.println ("After multiplying by " + q2.toString() + ", q1 is now "
+ q1.toString());
}
}
}
Here is the input file:
3 2 4 5 -16.75 0 3452.53 892.2
0 0 0 0 22.5 3.7 22.5 3.7 25 2 0.001 0.02
5.2 6.7 3.9 8.5
5.2 6.7 3.9 8.5
-15.5 18 3838.73 5.98
78.3 89.45 28.71 -19.58
0 0 0 0
0 0 0 0
1 0 1 0
1 0.5 0.5 0.75
1 0 1 0
1 0 1 0
Step by Step Solution
There are 3 Steps involved in it
Step: 1
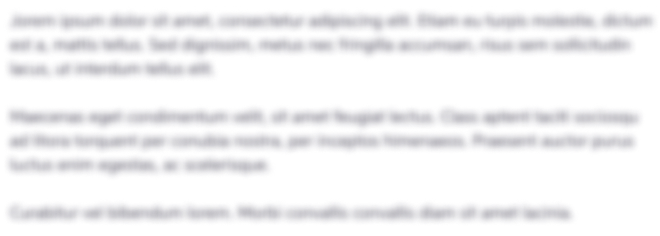
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started