Question
Homework 12 C++ For this program you will create an application for a travel agent that is booking summer vacations for students. The travel agent
Homework 12 C++
For this program you will create an application for a travel agent that is booking summer vacations for students. The travel agent has three trip types available, Beach, Mountain and Europe. Each trip has a different cost per night per person. There is a potentially never-ending line of students that want to book their vacations. The students have the option to review the details of the vacation before deciding to purchase the vacation. Once a student has decided to book a vacation they will need to provide their name, how many people are taking the vacation and how many nights they would like. At the end of the day, the travel agent would like to see a summary of vacations sold and the total sales.
Requirements
Vacations purchased are dynamically allocated and tracked within a vector
Vacations are polymorphically processed with at least one pure virtual function
A STL map will keep track of the price per night for each vacation type
You must appropriately deallocate memory in the destructors (and any other memory you dynamically allocate when you run your program).
Example interaction of the program
Welcome to SMU Summer Vacation Planning
What type of vacation are you interested in?
1. Beach
2. Mountain
3. Europe
Make your selection: 1
You have selected the following vacation:
Vacation Type: Beach
Cost per night for one person:
1 $500
2 $550
3 $600
4 $650
5 $700
6 $750
Would you like to keep it? (Enter y or n)n
Welcome to SMU Summer Vacation Planning
What type of vacation are you interested in?
1. Beach
2. Mountain
3. Europe
Make your selection: 2
You have selected the following vacation:
Vacation Type: Mountain
Cost per night for one person:
1 $100
2 $150
3 $200
4 $250
5 $300
6 $350
Would you like to keep it? (Enter y or n)y
Thank you for selecting a vacation.
What is the name for the reservation?SMU Student
How many attendees?2
How many nights?4
Your vacation costs $500
Is there another student wishing to purchase a vacation?(Enter y or n)y
Welcome to SMU Summer Vacation Planning
What type of vacation are you interested in?
1. Beach
2. Mountain
3. Europe
Make your selection: 3
You have selected the following vacation:
Vacation Type: Europe
Cost per night for one person:
1 $700
2 $750
3 $800
4 $850
5 $900
6 $950
Would you like to keep it? (Enter y or n)y
Thank you for selecting a vacation.
What is the name for the reservation?Mustang
How many attendees?4
How many nights?2
Your vacation costs $3000
Is there another student wishing to purchase a vacation?(Enter y or n)n
Sales Summary:
Type Name Attendees Nights Cost
Mountain SMU Student 2 4 $500
Europe Mustang 4 2 $3000
You made $3500 in sales today.
Vacation Interface
(Beach, Mountain and Europe interface not provided)
class Vacation {
public:
Vacation();
virtual ~Vacation() = default;
virtual string vacationDetails() = 0;
virtual string itenerary();
virtual int getTotalCost();
void setPurchaserName(const std::string&);
std::string getPurchaserName() const;
void setattendeeCount(const int&);
int getattendeeCount() const;
void setnights(const int&);
int getnights() const;
private:
int attendeeCount;
int nights;
string purchaserName;
map
};
Things to remember
Each file in your project must have File header comments. Header comments should include your name, class, project number, and a short description of what the file does.
Comment your code so whoever is reading it understands the intention.
Format your code. Proper formatting makes more code readable and is essential to writing good software.
Grading
Program correctness is worth 75% and design/formatting makes up the remaining 25%. See the page titled "Program Grading Rubric" on Canvas for details.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
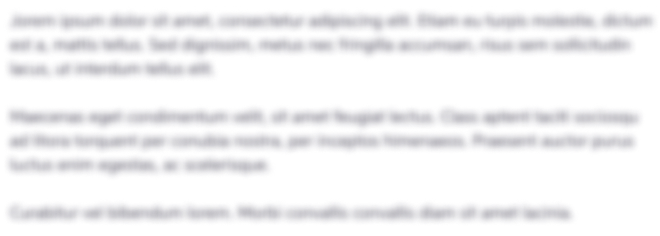
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started