Question
How am I able to solve this coding challenge Problem: Implement a Linked List class that inherits from an abstract list class. Instructions: Download the
How am I able to solve this coding challenge
Problem: Implement a Linked List class that inherits from an abstract list class. Instructions: Download the following files from D2L. project with the three files included. o List.h o Array.h o LinkedList.h The List.h and Array.h files are complete. Don't modify these files.
Implement the LinkedList class. All function signatures were created, you just need to implement them. The functions that need to be completed have a //TODO comment in the function body.
Your LinkedList function should be functionally the same as the array functions. You may test the array functions and look through the code to see how they work.
main program (Challenge1.cpp) to test your class. Make sure to test ALL the functions you implemented. You may test the array and linked list classes in a similar manner to ensure they work the same
List.H
#pragma once #include using namespace std; template class List { public: List() { length = 0; } //start with an empty list int Length() { return length; } void IncrementLength() { length++; } void DecrementLength() { length--; } //pure virtual functions that all child classes must implement virtual void Push(T element) = 0; virtual void Pop() = 0; virtual void Insert(T element, int index) = 0; virtual void Remove(int index) = 0; virtual T get(int index) = 0; virtual void print() = 0; private: int length; //the number of elements in the list };
Array.h
#pragma once #include "List.h" template class Array : public List { public: //constructors and destructors Array(); Array(int s); ~Array(); int Length() { return List::Length(); } //implement pure virtual functions void Push(T element); void Pop(); void Insert(T element, int index); void Remove(int index); T get(int index); void print(); private: void resize(); int size; //size of the currently allocated array T* arr; //pointer to the dynamic array }; template inline Array::Array():List() { size = 5; arr = new T[size]; //create dynamic array } template inline Array::Array(int s):List() { size = s; arr = new T[size]; //create dynamic array } template inline Array::~Array() { delete[] arr; //deallocate memory } template inline void Array::Push(T element) { //add an element to the end of the array if (Length() == size) resize(); //if the array is full, resize it to be larger. arr[Length()] = element; List::IncrementLength(); } template inline void Array::Pop() { //remove an element from the end of the array if (Length() > 0) { //can't pop from an empty array List::DecrementLength(); } } template inline void Array::Insert(T element, int index) { if (index >= Length() - 1) Push(element); else if (index >= 0) { if (Length() == size) resize(); //move elements to the right of index one position to the right for (int i = Length(); i > index; i--) { arr[i] = arr[i - 1]; } arr[index] = element; //put element in right location List::IncrementLength(); } } template inline void Array::Remove(int index) { //remove an element from a specific index if (index >= Length() - 1) Pop(); else if (index >= 0) { T e = arr[index]; //move elements to the right of index one position to the left for (int i = index; i < Length() - 1; i++) { arr[i] = arr[i + 1]; } List::DecrementLength(); } } template inline T Array::get(int index) { //return value in the array at given index return arr[index]; } template inline void Array::print() { //display the list with spaces between elements for (int i = 0; i < Length(); i++) { cout << arr[i] << " "; } cout << endl; } template inline void Array::resize() { //double the size of the dynamic array T* p = new T[size*2]; //allocate new memory for (int i = 0; i < size; i++) { p[i] = arr[i]; //copy elements to new memory } size *= 2; //update size delete[] arr; //deallocate previous array arr = p; //set pointer to point to new array }
LinkedList.H
#pragma once #include "List.h" template struct Node { //a linked list node T info; Node* link; }; template class LinkedList : public List { public: LinkedList(); //constructor ~LinkedList(); //destructor int Length() { return List::Length(); } //implement pure virtual functions void Push(T element); void Pop(); void Insert(T element, int index); void Remove(int index); T get(int index); void print(); private: Node* head; Node* tail; }; template inline LinkedList::LinkedList() { //TODO } template inline LinkedList::~LinkedList() { //TODO } template inline void LinkedList::Push(T element) { //TODO } template inline void LinkedList::Pop() { //TODO } template inline void LinkedList::Insert(T element, int index) { //TODO } template inline void LinkedList::Remove(int index) { //TODO } template inline T LinkedList::get(int index) { //TODO } template inline void LinkedList::print() { //TODO }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
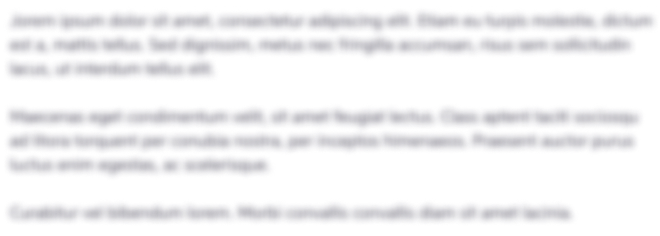
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started