Question
How do I put all four exercises in one class by writing each exercise in a separate method and invoke each method within your main
How do I put all four exercises in one class by writing each exercise in a separate method and invoke each method within your main method? (I need to call Permutation and Combination separately) Please copy and paste and show all exericses in 1 class. JAVA
NEEDED: to call each method in my main method and put all four exercises in one class
Exercise 1 & 2
1 import java.util.Scanner; 2 3 public class TheNinth { 4 5 6 7 public static void main(String[] args) { 8 9 long n, r; 10 11 Scanner scan = new Scanner(System.in); 12 13 14 15 System.out.print("Please enter \"n\" value: "); 16 17 n = scan.nextInt(); 18 19 20 21 System.out.print("Please enter \"r\" value: "); 22 23 r = scan.nextInt(); 24 25 26 27 System.out.println("Number of permutations(nPr) for given " + n + " and " + r + " values is " + permutation(n,r)); 28 29 System.out.println("Number of combinations(nCr) for given " + n + " and " + r + " values is " + combination(n,r)); 30 31 } 32 33 34 35 public static long factorial(long n) { 36 37 long i, fact = 1; 38 39 for (i = 1; i <= n; i++) { 40 41 42 43 if(fact ==0 ) 44 45 fact = 1; 46 47 fact = fact * i; 48 49 } 50 51 return fact; 52 53 } 54 55 56 57 public static long permutation(long n , long r ) { 58 59 return (factorial(n) / (factorial(n - r))); 60 61 } 62 63 64 65 public static long combination(long n , long r ) { 66 67 return (factorial(n) / (factorial(n - r) * factorial(r))); 68 69 } 70 71 }
exercise 3
1 import java.util.Random; 2 3 4 public class AverageStuff 5 { 6 7 public static double averageRandNum() 8 { 9 double sum=0; 10 int count=0; 11 for(int i=0;i<1000;i++) 12 { 13 double rnd=Math.random(); 14 15 sum+=rnd; 16 count++; 17 } 18 return (sum/count); 19 20 21 } 22 public static void main(String args[]) 23 { 24 double avg=averageRandNum(); 25 System.out.println("Average Random(1000) is: "+avg); 26 } 27 } 28 29
Exercise 4
1 public class ArrayIntersection { 2 3 public static int[] intersection(int A[], int B[]) 4 { 5 int n = A.length; 6 7 int C[] = new int[n]; 8 9 for (int i = 0; i < n; i++ ) 10 { 11 C[i] = -1; 12 } 13 14 15 int idx = 0; 16 for (int i = 0; i < n; i++ ) 17 { 18 for (int j = 0; j < n; j++ ) 19 { 20 if(A[i] == B[j]) 21 { 22 C[idx] = A[i]; 23 idx++; 24 break; 25 } 26 } 27 } 28 29 return C; 30 } 31 32 public static void main(String args[]) 33 { 34 int A[] = {1, 2, 3, 4, 5}; 35 36 int B[] = {6, 7, 8, 9, 10}; 37 int n = A.length; 38 int C[] = intersection(A, B); 39 40 System.out.print("A = "); 41 for (int i = 0; i < n; i++ ) 42 { 43 System.out.print(A[i] + " "); 44 } 45 46 System.out.println(); 47 48 System.out.print("B = "); 49 for (int i = 0; i < n; i++ ) 50 { 51 System.out.print(B[i] + " "); 52 } 53 54 System.out.print(" C = "); 55 for (int i = 0; i < n; i++ ) 56 { 57 System.out.print(C[i] + " "); 58 } 59 60 System.out.println(" "); 61 } 62 }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
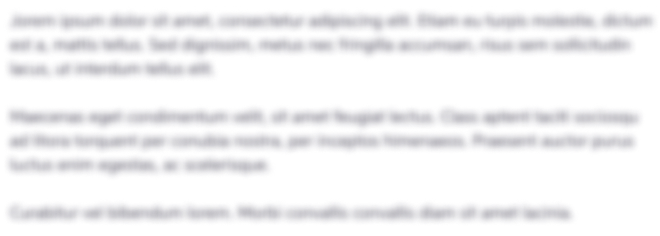
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started