Question
how to solve that a static method hasOnePeak that returns true if its LinkedList argument has a single peak, and false otherwise. This means that
how to solve that a static method hasOnePeak that returns true if its LinkedList argument has a single peak, and false otherwise. This means that the sequence of values consists wholly of an increasing part followed by a decreasing part (so the LL would have to have at least 3 elements before it could possibly have a peak). You may assume no two consecutive values in the array are the same (i.e., no flat areas). Your solution must use a constant amount of space (e.g., no additional arrays, ArrayLists, or LinkedLists) and run in linear time.
Hint: it might help to think about the list as representing a line graph, like MT 1 Problem 6 this semester, and draw the examples below. Any peaks in the examples below are shown in red.
list hasOnePeak(list) 2 4 7 false 2 7 4 true 7 4 2 false 2 4 false 2 false 2 4 7 9 3 2 true 2 4 7 9 7 3 7 1 false 7 5 4 false 2 4 2 3 false (has second increasing part at the end) 5 4 3 false We have provided a test driver for your method that tests it on several hard-coded cases, and compares the actual results with the expected results. The test driver source file, PeakTester.java, contains the stub for the hasOnePeak method.
public class PeakTester {
public static boolean hasOnePeak(LinkedList list) {
}
public static void main(String args[]) {
testPeak("", false); testPeak("3", false); testPeak("3 4", false); testPeak("4 2", false); testPeak("3 4 5", false); testPeak("5 4 3", false); testPeak("3 4 5 3", true); testPeak("3 4 5 3 2", true); testPeak("3 7 9 11 8 4 3 1", true); testPeak("3 5 4", true); testPeak("4 3 5", false); testPeak("2 4 3 5", false); testPeak("5 2 4 3 5", false); testPeak("5 2 4 3", false); testPeak("2 4 3 5 2", false); // 2 peaks }
/* Assumes the following format for listString parameter, shown by example * (first one is empty list): * "", "3", "3 4", "3 4 5", etc. */ private static LinkedList makeList(String listString) { Scanner strscan = new Scanner(listString);
LinkedList list = new LinkedList();
while (strscan.hasNextInt()) { list.add(strscan.nextInt()); }
return list; }
/* Test hasOnePeak method on a list form of input given in listString * Prints test data, result, and whether result matched expectedResult * * listString is a string form of a list given as a space separated * sequence of ints. E.g., * "" (empty string), "3" (1 elmt string), "2 4" (2 elmt string), etc. * */ private static void testPeak(String listString, boolean expectedResult) {
LinkedList list = makeList(listString);
boolean result = hasOnePeak(list); System.out.print("List: " + list + " hasOnePeak? " + result); if (result != expectedResult) { System.out.print("...hasOnePeak FAILED"); } System.out.println(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
You can solve this problem by iterating through the linked list and checking if there is a single pe...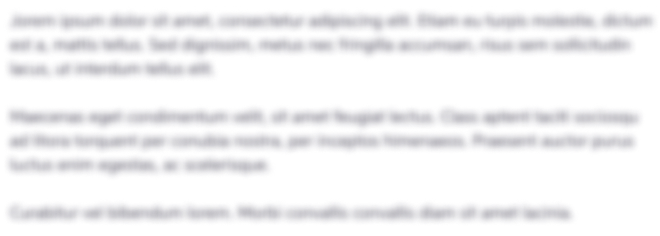
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started