Question
How would I code in C language to create a game in which a player goes through a series of rooms in which they can
How would I code in C language to create a game in which a player goes through a series of rooms in which they can find a prize or a monster to fight?
A player will enter the number of rooms (greater than 1) they want to endure. The program will dynamically allocate an array of Rooms and populate it with prizes and monsters. Then, the player will enter each room in which an action will occur:
- If the player enters a room with a prize, they will get a random value between 5 and 10, inclusive, added to their hitPoints.
- If the player enters a room with a monster, the player will lose hitPoints based on the monsters hitPoints and the players experiencePoints. The monsters hitPoints is a random value between 10 and 30, inclusive. The total number of hitPoints lost is equal to the monsters hitPoints minus the players experiencePoints. Should the players experiencePoints be greater than the monsters hitPoints, the player does not lose any hitPoints. Each time a player battles a monster (win or lose), the player will get an increase to their experiencePoints. This increase is a random value of 0 or 1.
- If a players hitPoints goes to zero or below at any point, they automatically lose.
- If a player completes all the rooms without their hitPoints going to zero at any time, they win.
create and write monsterbattle.c and monsterbattle.h
I will need to write source (.c) and header (.h) files for the game.
The header file will have the definitions for all the structs you need, an enum, and the prototypes for the functions below.
enum
Include this enum in your header file so you can use as values for determining what the room has.
typedef enum TYPE { EMPTY, PRIZE, MONSTER } TYPE;
structs
- Character this struct has two data members hitPoints and experiencePoints. This struct will represent a monster in the game. In this game, a monsters hitPoints represents the amount of damage it can inflict on the player and the experiencePoints is the number of experience points the player gains from battling this monster. Note that this struct will also be used to represent the player in which case the hitPoints represents their life points. Should this value go to zero or below, they have died. The experiencePoints for a player are used to deflect a monsters attack.
- Prize this struct has one data members points. The points is what is awarded to a players hitPoints should they enter a room with a prize.
- Room this struct represents a room. The game will create an array of these structs. The struct has three data members: type (see the enum above) to determine if this room is empty, has a monster, or a prize. If the room has a prize, then the prize data member will be initialized (see fillRooms()). If the room has a monster, then the monster data member will be initialized (see fillRooms()). If the room is empty, nothing happens with these data members. This struct is provided to you here:
typedef struct Room
{
TYPE type;
Character monster;
Prize prize;
} Room;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
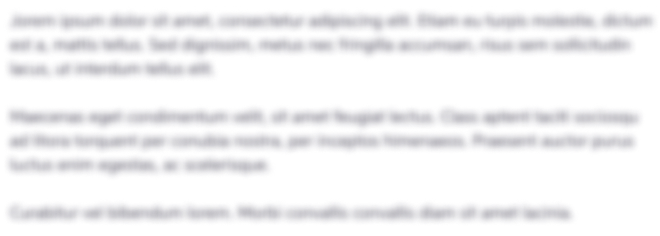
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started