Answered step by step
Verified Expert Solution
Question
1 Approved Answer
How's it going? So I need to have this code read from 2 files instead of asking the user to enter their information. I have
How's it going?
So I need to have this code read from 2 files instead of asking the user to enter their information. I have been trying to store the address of the file into a string variable, and the key as well. But, no luck so far. Would it be possible to do so? The code is a Vigenere Cipher by the way. Thank you!
import java.io.File; import java.io.IOException; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Scanner; import java.util.Random; import java.io.FileWriter; public class vignereCipher { public static void main(String arg[]){ Scanner sc = new Scanner(System.in); System.out.print("Enter the Encryption key : "); String key = sc.nextLine(); encrypt_file(key); //System.out.print("Enter the String to be encrypted : "); //String inString = sc.nextLine(); //String finalString = encrypt(inString, key); //System.out.print(" The encrypted String is : " + finalString); } // method that removes all the numbers, punctuations static String remove_ichars(String original_msg){ original_msg = original_msg.replaceAll("[^A-Z ]", ""); return original_msg; } static String encrypt(String original_msg, String key){ // allchars is our keyspace String allChars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; int all_chars_length = allChars.length(); String encrypted_msg = ""; // converting our string to uppercase original_msg = original_msg.toUpperCase(); // removing all numbers, punctuations original_msg = remove_ichars(original_msg); // converting key to uppercase key = key.toUpperCase(); int index = 0; // iterating over the original message character by chracter and decrypting it and appending it to the new string for(int i=0; i< original_msg.length(); i++){ Character letter = original_msg.charAt(i); if(allChars.indexOf(letter) >= 0) { int position = (allChars.indexOf(letter) + allChars.indexOf(key.charAt(index))) % all_chars_length; Character e_letter = allChars.charAt(position); encrypted_msg += e_letter; index++; if(index >= key.length()){ index %= key.length(); } }else{ encrypted_msg += letter; index = 0; } } return encrypted_msg; } static void encrypt_file(String key){ FileInputStream fin; Scanner sc = new Scanner(System.in); System.out.print("Enter the path of the file : "); String file_name = sc.nextLine(); String fullFile = ""; try { fin = new FileInputStream(file_name); Scanner fp = new Scanner(fin); int size = 0; while (fp.hasNextLine()) { String line = fp.nextLine(); fullFile += line + " "; size += line.length(); } System.out.println("File read successfully!!"); fullFile = encrypt(fullFile, key); System.out.println("Data encrypted successfully!!"); System.out.println(fullFile); }catch (Exception e){ e.printStackTrace(); } try { File newTextFile = new File("C:\\Users\\MOHIT\\Desktop\\encrypted_file.txt"); FileWriter f1 = new FileWriter(newTextFile); f1.write(fullFile); f1.close(); System.out.println("Encrypted file stored on Desktop"); } catch (IOException ex) { ex.printStackTrace(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
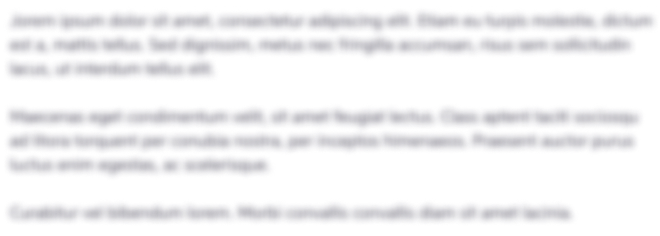
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started