Question
I appreciate any help given. I need help modifying the following code LinkedList to make the following corrections. In the remove method, if the index
I appreciate any help given. I need help modifying the following code LinkedList to make the following corrections.
In the remove method, if the index is exactly the same as the size, it should throw an IndexOutOfBoundsException. This code throws a NullPointerException. On line 47 it should be if(index < 0 || index >= size).
My remove method doesn't return the correct element in the case where index > 0. This code was used to test it: List
The remove method should remove and return the element and index 1, so it should return 5, but my method returns 3.
On line 57, the temp!=null is unnecessary, because that would only happen if the index is out of bounds, but that case was handled earlier.
You are using O(n) time to update last (lines 65-68), but it can be done in O(1) time.
The code doesn't seem to be using a dummy node, so you don't need to create a new node in the constructor.
The following is the code to be corrected:
import java.util.ArrayList;
public class LinkedList
private Node
private int size = 0;
// Construct a new empty list.
public LinkedList() {
first = last = new Node<>(null, null);
}
// Adds element e to the end of the list.
public void add(E e) {
Node
if(size == 0) {
first = temp;
last = first;
last.next = null;
++size;
return;
}
last.next = temp;
last = last.next;
last.next = null;
++size;
}
// Returns the element at the specified index,
// or throws an IndexOutOfBoundsException if the index is out of range.
public E get(int index) {
if (index < 0 || index >= size)
throw new IndexOutOfBoundsException();
Node
for (int i = 0; i < index; ++i)
t = t.next;
return t.data;
}
// Removes and returns the element at the specified index,
// or throws an IndexOutOfBoundsException if the index is out of range.
public E remove(int index) {
if(index < 0 || index > size)
throw new IndexOutOfBoundsException("Index out of bound...");
Node
if(index == 0) {
--size;
first = first.next;
return temp.data;
}
// Find previous node of the node to be deleted
for (int i=0; temp!=null && i temp = temp.next; // Node temp->next is the node to be deleted // Store pointer to the next of node to be deleted Node temp.next = next; // Unlink the deleted node from list --size; Node while(t.next != null) { t = t.next; } last = t; return temp.data; } // Adds element e to the top of the stack. public void push(E e) { Node if(size == 0) { first = last = temp; last.next = null; ++size; return; } temp.next = first; first = temp; ++size; } // Removes and returns the top element of the stack, or returns null if the stack is empty. public E pop() { if(size == 0) { return null; } Node first = first.next; --size; return temp.data; } // Returns but does not remove the top element of the stack, or returns null if the stack is empty. public E top() { return first.data; } // Reverses the order of all the elements of the stack. public void reverse() { ArrayList while(size != 0) { list.add(pop()); } for(E item : list) { push(item); } } // Returns a string representation of the linked list. public String toString() { String str = ""; Node while(temp != null) { str += temp.data.toString() + " "; temp = temp.next; } return str; } // Returns the number of elements. public int size() { return size; } private static class Node E data; Node Node(E data, Node this.data = data; this.next = next; } } } ____________________________________________________________ The following classes are not to be modified in any way but are used to run the LinkedList class: public class HW1 { public static void main(String[] args) { List __________________________________________________ public interface List ____________________________________________________________________________ public interface Stack
Step by Step Solution
There are 3 Steps involved in it
Step: 1
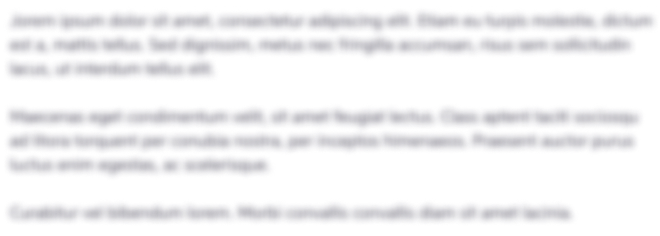
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started