Question
I coded my program all in one file, but I am supposed to do the modular approach to writiting C, so I tried converting the
I coded my program all in one file, but I am supposed to do the modular approach to writiting C, so I tried converting the file to seprate pieces like it wanted, but I am unable to pass my pointer correctly, how would I do so? Each of my functions are in their own .c files shown below:
-------------------------------------------------------------------main.c
#include "my.h" int *pointerNUM; //<--------------------------------------------------here is the pointer // main function int main(int argc, char *argv[]) { // To store the numbers of number in file int numberOfNUM; // Calls the function to read numbers and stores the length numberOfNUM = readFile(argc, argv); // Calls the function to displays the numbers before sorting printf(" Before sort : "); show(numberOfNUM); // Calls the function for sorting insertSORT(numberOfNUM); // Calls the function to displays the numbers after sorting printf(" After sort: "); show(numberOfNUM); }// End of main function
------------------------------------------------------------------------------------insertSORT.c
#include "my.h" // Function for insertion sort void insertSORT(int numberOfNUM) { int x, key, y; // Loops numberOfNUM times for (x = 1; x < numberOfNUM; x++) { // Stores i index position data in key key = pointerNUM[x]; // Stores x minus one as y value y = x - 1;
/* Move elements of pointerNUM[0..x - 1], that are greater than key, to one position ahead of their current position */ while (y >= 0 && pointerNUM[y] > key) { // Stores pointerNUM y index position value at pointerNUM y next index position pointerNUM[y + 1] = pointerNUM[y]; // Decrease the y value by one y = y - 1; }// End of while // Stores the key value at pointerNUM y plus one index position pointerNUM[y + 1] = key; }// End of for loop }// End of function
---------------------------------------------------------------------------readFile.c
#include "my.h" // Read in the parts file and returns the length int readFile(int argc, char *argv[]) { // File pointer FILE *fptr; // numberOfNUM for number of numbers // cntVAR for counter variable int numberOfNUM, cntVAR; // Open the file for reading fptr = fopen(argv[1], "r"); // "r" for read
// Check that it opened properly if (fptr == NULL) { printf("Cannot open file "); exit(0); }// End of if condition // Reads number of numbers in the file fscanf(fptr, "%d", &numberOfNUM);
// Dynamically allocates memory to pointer pointerNUM pointerNUM = (int *) calloc(numberOfNUM, sizeof(int)); // Loops numberOfNUM times for(cntVAR = 0; cntVAR < numberOfNUM; cntVAR++) // Reads each number and stores it in array fscanf(fptr, "%d", &pointerNUM[cntVAR]); // Returns the length of the numbers return numberOfNUM; fclose(fptr); }// End of function
-------------------------------------------------------------------------------------show.c
#include "my.h" // Function to show numbers void show(int numberOfNUM) { int cntVAR; // Loops numberOfNUM times for(cntVAR = 0; cntVAR < numberOfNUM; cntVAR++) // Displays each number printf("%4d, ", pointerNUM[cntVAR]); }// End of function
---------------------------------------------------------------------------------Now my my.h
#include #include
//prototypes void insetSORT(int numberOfNUM); int readFile(int argc, char *argv[]); void show(int numberOfNUM);
--------------------------------------------------------------------------------
When I compile I get issues such as:
insertSORT.c: In function insertSORT: insertSORT.c:10: error: pointerNUM undeclared (first use in this function) insertSORT.c:10: error: (Each undeclared identifier is reported only once insertSORT.c:10: error: for each function it appears in.) readFile.c: In function readFile: readFile.c:23: error: pointerNUM undeclared (first use in this function) readFile.c:23: error: (Each undeclared identifier is reported only once readFile.c:23: error: for each function it appears in.) show.c: In function show: show.c:9: error: pointerNUM undeclared (first use in this function) show.c:9: error: (Each undeclared identifier is reported only once show.c:9: error: for each function it appears in.)
--------------------------------------------------------------------------------------------
This is my file before I tried to convert to the modular approach to writting C:
#include #include // Pointer to store numbers int *pointerNUM; // Read in the parts file and returns the length int readFile(int argc, char *argv[]) { // File pointer FILE *fptr; // numberOfNUM for number of numbers // cntVAR for counter variable int numberOfNUM, cntVAR; // Open the file for reading fptr = fopen(argv[1], "r"); // "r" for read
// Check that it opened properly if (fptr == NULL) { printf("Cannot open file "); exit(0); }// End of if condition // Reads number of numbers in the file fscanf(fptr, "%d", &numberOfNUM);
// Dynamically allocates memory to pointer pointerNUM pointerNUM = (int *) calloc(numberOfNUM, sizeof(int)); // Loops numberOfNUM times for(cntVAR = 0; cntVAR < numberOfNUM; cntVAR++) // Reads each number and stores it in array fscanf(fptr, "%d", &pointerNUM[cntVAR]); // Returns the length of the numbers return numberOfNUM; fclose(fptr); }// End of function
// Function to display numbers void display(int numberOfNUM) { int cntVAR; // Loops numberOfNUM times for(cntVAR = 0; cntVAR < numberOfNUM; cntVAR++) // Displays each number printf("%4d, ", pointerNUM[cntVAR]); }// End of function
// Function for insertion sort void insertionSort(int numberOfNUM) { int x, key, y; // Loops numberOfNUM times for (x = 1; x < numberOfNUM; x++) { // Stores i index position data in key key = pointerNUM[x]; // Stores x minus one as y value y = x - 1;
/* Move elements of pointerNUM[0..x - 1], that are greater than key, to one position ahead of their current position */ while (y >= 0 && pointerNUM[y] > key) { // Stores pointerNUM y index position value at pointerNUM y next index position pointerNUM[y + 1] = pointerNUM[y]; // Decrease the y value by one y = y - 1; }// End of while // Stores the key value at pointerNUM y plus one index position pointerNUM[y + 1] = key; }// End of for loop }// End of function void insertionSort(int numberOfNUM); int readFile(int argc, char *argv[]); void display(int numberOfNUM); // main function int main(int argc, char *argv[]) { // To store the numbers of number in file int numberOfNUM; // Calls the function to read numbers and stores the length numberOfNUM = readFile(argc, argv); // Calls the function to displays the numbers before sorting printf(" Before sort : "); display(numberOfNUM); // Calls the function for sorting insertionSort(numberOfNUM); // Calls the function to displays the numbers after sorting printf(" After sort: "); display(numberOfNUM); }// End of main function
-----------------------------------------------------------------------------------
The modular directions are as follows:
1. For each function, including main(), f() that is written store it as f.c and put #include "my.h" as first line. Put all functions and my.h in a folder by themselves. You should use at least one other function besides main() 2. Compile each function separately without linking , as in $ gcc -c f.c or to do them all at once $ gcc -c *.c-this produces f.o, etc. 3. Compile and link all .o's together , as in $ gcc *.o 4. $./a.out runs the program or $./a.out myfile etc 5. $ shar *.c my.h >bigone 6. $ turnin bigone kmartin.cop3530.a2 for example. 7. When I get the code, I $ unshar bigone 8. $ gcc -c *.c 9. $ gcc *.o etc In my.h put # include # include that you need All function prototypes separated by semicolons All structures separated by semicolons NO variables
Step by Step Solution
There are 3 Steps involved in it
Step: 1
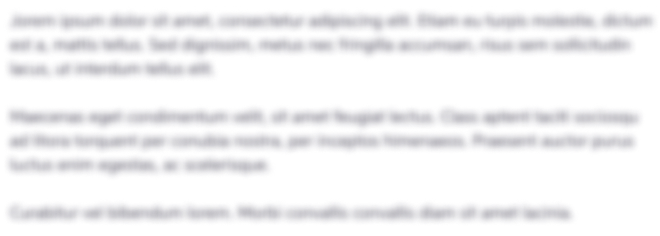
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started