Question
I desperately need to figure out this lab I have been stuck on for dozens of hours and weeks now. I just tried to post
I desperately need to figure out this lab I have been stuck on for dozens of hours and weeks now.
I just tried to post this question but the screen froze 90% of the way through.
Anyways, underneath the assignment I'm struggling to grapple with. It includes three java class files that together compile into a running program. It runs and displays a menu of user command options. The problem is the contains user command, get user command, indexOf command and lastIndexOf command contain no code and thus do not function and just return a basic generic response.
The problem is to code these methods so the commands function properly when any of the three text files are loaded using the "load" command.
The three java files are: Lab24a.java , MyLinkedList.java and MyList.java and I included them in text below the assignment pdf below.
The three test text files are right below them as well, the files being: names.txt, cities.txt and firstTen.txt (referring to the first ten presidents). PleaseHelp, it is so important.
The Following are the three starter java classes, which together run, but the commands do not return the correct results.
1. Lab24a.java
/*
* CIT242 Lab24a: Add enhancements to linked list class.
*/
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.*;
public class Lab24a {
private static boolean verboseMode = false; // Debug aid (== false by default)
static String userInput;
private static MyLinkedList
/**
* Output information regarding user commands.
*/
public static void outputHelpText() {
System.out.println(" COMMAND PARAMETER(S) DESCRIPTION");
System.out.println(" help Output this help text.");
System.out.println(" contains string Test if list contains string (incomplete).");
System.out.println(" first string Get the first index of specified string (incomplete).");
System.out.println(" get index Get the list item at specified index (incomplete).");
System.out.println(" last string Get the last index of specified string (incomplete).");
System.out.println(" load filename Read input file into memory.");
System.out.println(" print Output memory data to console.");
System.out.println(" verbose Turn VERBOSE mode ON or OFF (optional feature).");
System.out.println(" q exit the program.");
System.out.println();
}
//_________________________________________________________________________
// Main method.
public static void main(String[] args)
throws IOException {
Scanner input = new Scanner(System.in);
System.out.println("This program accepts the following inputs and performs the corresponding actions:");
outputHelpText();
do {
System.out.print("Command: ");
userInput = input.nextLine();
if (Lab24a.getVerboseMode()) {
System.out.printf("userInput=%s ", userInput);
}
if (userInput.charAt(0) == 'h') {
outputHelpText();
} else if (userInput.toUpperCase().equals("VERBOSE")) {
Lab24a.setOrClearVerboseMode();
if (Lab24a.getVerboseMode()) {
System.out.println("Verbose mode is now ON.");
} else {
System.out.println("Verbose mode is now OFF.");
}
} else if (userInput.charAt(0) != 'q') {
processCommand(userInput.split("[ ]"));
}
} while (userInput.charAt(0) != 'q');
System.out.println("Exit program.");
} // end main
static void processCommand(String[] commandArgs) {
String command;
if (commandArgs.length
System.out.println("Ignoring empty command.");
} else if (commandArgs[0].equalsIgnoreCase("LOAD")) {
if (commandArgs.length
System.out.println("Usage: LOAD filename");
} else {
ArrayList
list = new MyLinkedList(inputStrings.toArray());
}
} else if (list == null) {
System.out.printf("Please LOAD data file before attempting %s command. ",
commandArgs[0]);
} else if (commandArgs[0].equalsIgnoreCase("PRINT")) {
System.out.println(list);
} else if (commandArgs[0].equalsIgnoreCase("CONTAINS")) {
if (commandArgs.length
System.out.println("Usage: CONTAINS string");
} else {
boolean result = list.contains(commandArgs[1]);
System.out.printf("Result = %b for command: CONTAINS %s ", result, commandArgs[1]);
}
} else if (commandArgs[0].equalsIgnoreCase("GET")) {
if (commandArgs.length
System.out.println("Usage: GET index");
} else {
String result = list.get(Integer.parseInt(commandArgs[1]));
System.out.printf("Result = %s for command: GET %s ", result, commandArgs[1]);
}
} else if (commandArgs[0].equalsIgnoreCase("FIRST")) {
if (commandArgs.length
System.out.println("Usage: FIRST string");
} else {
int result = list.indexOf(commandArgs[1]);
System.out.printf("Result = %d for command: FIRST %s ", result, commandArgs[1]);
}
} else if (commandArgs[0].equalsIgnoreCase("LAST")) {
if (commandArgs.length
System.out.println("Usage: LAST string");
} else {
int result = list.lastIndexOf(commandArgs[1]);
System.out.printf("Result = %d for command: LAST %s ", result, commandArgs[1]);
}
} else {
System.out.printf("Unknown command: %s ", commandArgs[0]);
}
}// end processCommand
/**
* Read the contents of a text file into an ArrayList of String objects.
*
* @param fName = file name
* @return
*/
static ArrayList
File documentFile = new File(fName);
ArrayList
contents = new ArrayList();
Scanner input;
int lineNum = 0;
try {
input = new Scanner(documentFile);
while (input.hasNextLine()) {
String inputText = input.nextLine();
if (Lab24a.getVerboseMode()) {
System.out.println("inputText=" + inputText);
}
lineNum++;
contents.add(inputText.trim());
}
System.out.printf("%d records loaded. ", lineNum);
} catch (FileNotFoundException FNF) {
System.out.printf("file not found: %s ", fName);
}
return contents;
}// end loadFromFile
//________________________________________________________________________________________
/**
*
* @return the current value of the Boolean 'verboseMode' attribute.
*/
static Boolean getVerboseMode() {
return verboseMode;
}
//________________________________________________________________________________________
/**
*
* @param value = new value for the Boolean 'verboseMode' attribute.
*/
static void setVerboseMode(Boolean value) {
verboseMode = value;
}
//________________________________________________________________________________________
/**
* Turn "verbose mode" ON (true) or OFF (false).
*/
static void setOrClearVerboseMode() {
if (verboseMode) {
verboseMode = false;
} else {
verboseMode = true;
}
}
} // end class Lab24a
2. MyLinkedList.java
public class MyLinkedList
private Node
private int size = 0; // Number of elements in the list
/** Create an empty list */
public MyLinkedList() {
}
/** Create a list from an array of objects */
public MyLinkedList(E[] objects) {
for (int i = 0; i
add(objects[i]);
}
/** Return the head element in the list */
public E getFirst() {
if (size == 0) {
return null;
}
else {
return head.element;
}
}
/** Return the last element in the list */
public E getLast() {
if (size == 0) {
return null;
}
else {
return tail.element;
}
}
/** Add an element to the beginning of the list */
public void addFirst(E e) {
Node
newNode.next = head; // link the new node with the head
head = newNode; // head points to the new node
size++; // Increase list size
if (tail == null) // the new node is the only node in list
tail = head;
}
/** Add an element to the end of the list */
public void addLast(E e) {
Node
if (tail == null) {
head = tail = newNode; // The new node is the only node in list
}
else {
tail.next = newNode; // Link the new with the last node
tail = newNode; // tail now points to the last node
}
size++; // Increase size
}
@Override /** Add a new element at the specified index
* in this list. The index of the head element is 0 */
public void add(int index, E e) {
if (index == 0) {
addFirst(e);
}
else if (index >= size) {
addLast(e);
}
else {
Node
for (int i = 1; i
current = current.next;
}
Node
current.next = new Node(e);
(current.next).next = temp;
size++;
}
}
/** Remove the head node and
* return the object that is contained in the removed node. */
public E removeFirst() {
if (size == 0) {
return null;
}
else {
E temp = head.element;
head = head.next;
size--;
if (head == null) {
tail = null;
}
return temp;
}
}
/** Remove the last node and
* return the object that is contained in the removed node. */
public E removeLast() {
if (size == 0) {
return null;
}
else if (size == 1) {
E temp = head.element;
head = tail = null;
size = 0;
return temp;
}
else {
Node
for (int i = 0; i
current = current.next;
}
E temp = tail.element;
tail = current;
tail.next = null;
size--;
return temp;
}
}
@Override /** Remove the element at the specified position in this
* list. Return the element that was removed from the list. */
public E remove(int index) {
if (index = size) {
return null;
}
else if (index == 0) {
return removeFirst();
}
else if (index == size - 1) {
return removeLast();
}
else {
Node
for (int i = 1; i
previous = previous.next;
}
Node
previous.next = current.next;
size--;
return current.element;
}
}
@Override /** Override toString() to return elements in the list */
public String toString() {
StringBuilder result = new StringBuilder("[");
Node
for (int i = 0; i
result.append(current.element);
current = current.next;
if (current != null) {
result.append(", "); // Separate two elements with a comma
}
else {
result.append("]"); // Insert the closing ] in the string
}
}
return result.toString();
}
@Override /** Clear the list */
public void clear() {
size = 0;
head = tail = null;
}
@Override /** Return true if this list contains the element e */
public boolean contains(Object e) {
// Left as an exercise
return true;
}
@Override /** Return the element at the specified index */
public E get(int index) {
// Left as an exercise
return null;
}
@Override /** Return the index of the head matching element in
* this list. Return -1 if no match. */
public int indexOf(Object e) {
// Left as an exercise
return 0;
}
@Override /** Return the index of the last matching element in
* this list. Return -1 if no match. */
public int lastIndexOf(E e) {
// Left as an exercise
return 0;
}
@Override /** Replace the element at the specified position
* in this list with the specified element. */
public E set(int index, E e) {
// Left as an exercise
return null;
}
@Override /** Override iterator() defined in Iterable */
public java.util.Iterator
return new LinkedListIterator();
}
private class LinkedListIterator
implements java.util.Iterator
private Node
@Override
public boolean hasNext() {
return (current != null);
}
@Override
public E next() {
E e = current.element;
current = current.next;
return e;
}
@Override
public void remove() {
// Left as an exercise
}
}
private static class Node
E element;
Node
public Node(E element) {
this.element = element;
}
}
@Override /** Return the number of elements in this list */
public int size() {
return size;
}
}
3. MyList.java
import java.util.Collection;
public interface MyList
/** Add a new element at the specified index in this list */
public void add(int index, E e);
/** Return the element from this list at the specified index */
public E get(int index);
/** Return the index of the first matching element in this list.
* Return -1 if no match. */
public int indexOf(Object e);
/** Return the index of the last matching element in this list
* Return -1 if no match. */
public int lastIndexOf(E e);
/** Remove the element at the specified position in this list
* Shift any subsequent elements to the left.
* Return the element that was removed from the list. */
public E remove(int index);
/** Replace the element at the specified position in this list
* with the specified element and returns the new set. */
public E set(int index, E e);
@Override /** Add a new element at the end of this list */
public default boolean add(E e) {
add(size(), e);
return true;
}
@Override /** Return true if this list contains no elements */
public default boolean isEmpty() {
return size() == 0;
}
@Override /** Remove the first occurrence of the element e
* from this list. Shift any subsequent elements to the left.
* Return true if the element is removed. */
public default boolean remove(Object e) {
if (indexOf(e) >= 0) {
remove(indexOf(e));
return true;
}
else
return false;
}
@Override
public default boolean containsAll(Collection> c) {
// Left as an exercise
return true;
}
@Override
public default boolean addAll(Collection extends E> c) {
// Left as an exercise
return true;
}
@Override
public default boolean removeAll(Collection> c) {
// Left as an exercise
return true;
}
@Override
public default boolean retainAll(Collection> c) {
// Left as an exercise
return true;
}
@Override
public default Object[] toArray() {
// Left as an exercise
return null;
}
@Override
public default
// Left as an exercise
return null;
}
}
And the following are the three test text files to run through the java program :
1. names.txt
Tom
Susan
Kim
George
James
Jean
George
Jane
Denise
Jenny
Kathy
Jane
2. cities.txt
Boston
Paris
Philadelphia
Miami
London
Paris
Chicago
3. firstTen.txt
Washington
Adams
Jefferson
Madison
Monroe
Adams
Jackson
VanBuren
Harrison
Tyler
Step by Step Solution
There are 3 Steps involved in it
Step: 1
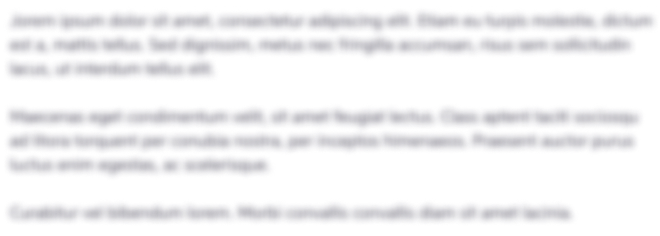
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started