Question
I have a bug in my java code. I've tried everything. I'm still getting duplicates even after shuffling, and the player is picking 6 cards
I have a bug in my java code. I've tried everything. I'm still getting duplicates even after shuffling, and the player is picking 6 cards instead of five. please help me with a solution that works, that is, picking only 5 cards without getting duplicates. Please, run my code on your machine because it feels like I'm the only one whose code isn't working even with the same solution. Please and thank you.
HERE IS MY CODE:
CARD CLASS
public class Card {
public static final int ACE = 1; public static final int TWO = 2; public static final int THREE = 3; public static final int FOUR = 4; public static final int FIVE = 5; public static final int SIX = 6; public static final int SEVEN = 7; public static final int EIGHT = 8; public static final int NINE = 9; public static final int TEN = 10; public static final int JACK= 11; public static final int QUEEN = 12; public static final int KING = 13; public static final int CLUBS = 1; public static final int DIAMONDS = 2; public static final int HEARTS = 3; public static final int SPADES = 4; public int face, suit; public String faceName, suitName; Random rand = new Random(); public Card() { int randomFace = rand.nextInt(13) + 1; int randomSuit = rand.nextInt(4) + 1; face = randomFace; suit= randomSuit; } public Card(int face, int suit) { this.face = face; this.suit = suit; } @Override public String toString() { switch(face) { case ACE: faceName = "Ace"; break; case TWO: faceName = "Two"; break; case THREE: faceName = "Three"; break; case FOUR: faceName = "Four"; break; case FIVE: faceName = "Five"; break; case SIX: faceName = "Six"; break; case SEVEN: faceName = "Seven"; break; case EIGHT: faceName = "Eight"; break; case NINE: faceName = "Nine"; break; case TEN: faceName = "Ten"; break; case JACK: faceName = "Eleven"; break; case QUEEN: faceName = "Twelve"; break; case KING: faceName = "Thirteen"; break; } switch(suit) { case CLUBS: suitName = "Clubs"; break; case DIAMONDS: suitName = "Diamonds"; case HEARTS: suitName = "Hearts"; case SPADES: suitName = "Spades"; } return faceName + " of " + suitName; } }
PLAYER CLASS
public class Player {
private Card[]cards; private int numCards; Random rand = new Random(); public Player() { cards = new Card[5]; deck(); shuffle(); }
public void deck() { Card[] deckOfCards = new Card[52];
int cardIndex = 0;
for (int face = Card.ACE; face
this.numCards = 52; this.cards = deckOfCards; }
public void shuffle() { int N = this.numCards; for (int i = 0; i
public void drawCards() {
for (int i=0; i
@Override public String toString() { String cardsToString = "";
for (int i = 0; i
return cardsToString; } }
DRIVER CLASS
public class Driver {
public static void main(String[]args) { Player b = new Player(); b.drawCards(); System.out.println(b); } }
Q. Problems a Javadoc Declaration E Console Driver (1) [Java Application] C:IProgram Files\Javaljdk-17.0.4\bin\javaw.exe (Feb 20, 2023, 12:13:35 PM - 12:13: Four of Spades Four of Spades Two of Spades Eight of Spades Seven of Spades Eleven of Spades
Step by Step Solution
There are 3 Steps involved in it
Step: 1
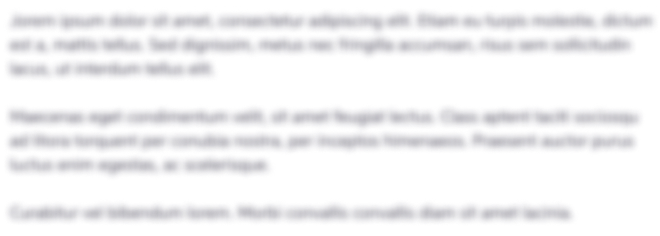
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started