Question
I have a java question. I have included the relevant code but let me know if you need more. I have two classes, a main
I have a java question. I have included the relevant code but let me know if you need more. I have two classes, a main window and a seperate window that pops up when you click edit on the main window. I am trying to make it so that when the user clicks the button on the edit window, it removes everything and then repaints it on the edit window. However, adding a panel after removeAll() is called doesnt seem to work. Here is my code:
editWindow:
import java.awt.BorderLayout; import java.awt.FlowLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener;
import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.JTextField; import javax.swing.SwingConstants;
public class editWindow extends JFrame implements ActionListener, SwingConstants { taskBoardModel editBoard; JPanel mainPanel; JPanel columnPanel; JPanel currentPanel; JButton addButton = new JButton("Add"); JTextField newColumn = new JTextField("",25); JPanel newPanel = new JPanel(); JLabel newLabel = new JLabel("WORK"); public editWindow(taskBoardModel userBoard,JPanel updatePanel) { newPanel.add(newLabel); this.editBoard = userBoard; this.currentPanel = updatePanel; setTitle("Edit Window"); this.setSize(350, 250); this.setLayout(new FlowLayout()); this.add(addButton); this.add(newColumn); addButton.addActionListener(this);
this.removeAll();
this.revalidate(); newPanel.setVisible(true); this.add(newPanel); this.revalidate(); this.repaint(); updateScreen(); setVisible(true); } public void actionPerformed(ActionEvent ae) { mainScreen newScreen = new mainScreen();
/* if(ae.getSource() == addButton) { this.removeAll(); this.add(newPanel); newPanel.setVisible(true); this.revalidate(); this.repaint(); editBoard.columns.get(0).columnName = "CAT POOP"; editBoard.columns.get(1).columnName = "SECOND POOP"; String columnString = newColumn.getText(); if(columnString.equals("")) { //JOptionPane.showMessageDialog(null, "Please enter a column name"); } else { columnModel newColumn = new columnModel(columnString); editBoard.columns.add(newColumn); } //JOptionPane.showMessageDialog(null, editBoard.columns.get(3).columnName); //newScreen.drawMenu(editBoard); updateScreen(); currentPanel.revalidate(); currentPanel.repaint(); } */ }
public void updateScreen() { mainScreen newScreen = new mainScreen(); //columnModel updateColumn; JPanel columnPanel; /* for(int i=0;i { updateColumn = editBoard.columns.get(i); editBoard.columns.get(i).columnLabel.setText(updateColumn.columnName); } */ columnPanel = newScreen.drawColumns(editBoard.columns); currentPanel.removeAll(); currentPanel.add(columnPanel); this.revalidate(); this.repaint(); //columnPanel = newScreen.drawColumns(editBoard.columns); // currentPanel.removeAll(); //currentPanel.add(columnPanel); } }
manScreen:
import java.awt.BorderLayout; import java.awt.Button; import java.awt.Color; import java.awt.FlowLayout; import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.GridBagConstraints; import java.awt.GridBagLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.ArrayList; import java.util.Collections;
import javax.swing.BorderFactory; import javax.swing.JButton; import javax.swing.JComboBox; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JPanel;
public class mainScreen extends JPanel implements ActionListener { JFrame mainFrame = new JFrame("Task Board"); JPanel mainPanel = new JPanel(); editWindow ew; taskBoardModel userBoard;
JButton editButton = new JButton("Edit");
JLabel columnNameLabel; JLabel columnLabel; public void mainScreen(taskBoardModel getBoard) { userBoard = getBoard;
//Collections.swap(userBoard.columns, 0, 1);
this.drawMenu(userBoard); //this.drawColumns(userBoard.columns); } public void updateMenu() { JPanel columnUpdate; columnUpdate = this.drawColumns(userBoard.columns);
/* columnModel updateColumn; for(int i=0;i { updateColumn = userBoard.columns.get(i); userBoard.columns.add(updateColumn); } */ } public void drawMenu(taskBoardModel userBoard) { JPanel menuPanel = new JPanel(); mainFrame.setLayout(new BorderLayout()); mainPanel.setLayout(new FlowLayout(FlowLayout.LEFT)); menuPanel.setLayout(new BorderLayout()); JPanel menuLeft = new JPanel(); JPanel menuRight = new JPanel(); menuLeft.setLayout(new FlowLayout()); menuRight.setLayout(new FlowLayout()); JLabel selectLabel = new JLabel("Select Project: "); JComboBox selectBox = new JComboBox(); selectBox.addItem(""); //JButton editButton = new JButton("Edit"); JButton saveButton = new JButton("Save"); JButton deleteButton = new JButton("Delete"); //JButton editButton = new JButton("Edit"); //editButton = new JButton("Edit"); editButton.addActionListener(this); menuLeft.add(selectLabel); menuLeft.add(selectBox); menuLeft.add(saveButton); menuLeft.add(editButton); menuLeft.add(deleteButton); JButton loadButton = new JButton("Load..."); JButton createButton = new JButton("Create new"); JButton logoutButton = new JButton("Logout"); menuRight.add(loadButton); menuRight.add(createButton); menuRight.add(logoutButton); menuPanel.add(menuLeft,BorderLayout.WEST); menuPanel.add(menuRight,BorderLayout.EAST); menuPanel.setBorder(BorderFactory.createLineBorder(Color.black));
JPanel columnPanel = drawColumns(userBoard.columns); mainPanel.add(columnPanel); mainFrame.add(menuPanel,BorderLayout.NORTH); mainFrame.add(mainPanel,BorderLayout.CENTER); mainFrame.setSize(1000, 1000); mainFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
mainPanel.setVisible(true);
mainFrame.setVisible(true); mainFrame.revalidate(); mainFrame.repaint(); mainPanel.revalidate(); mainPanel.repaint();
} public void actionPerformed(ActionEvent ae) { if(ae.getSource() == editButton) { editWindow ew = new editWindow(userBoard,mainPanel);
ew.setVisible(true); //ew.revalidate(); //ew.repaint(); } } public JPanel drawColumns(ArrayList userColumn) { JPanel columnPanel = new JPanel(); JPanel taskPanel = new JPanel(); columnPanel.setLayout(new FlowLayout(FlowLayout.LEFT)); columnModel currentColumn; for(int i=0;i { currentColumn = userColumn.get(i); JPanel panel = new JPanel(); panel.setLayout(new BorderLayout()); columnLabel = new JLabel(currentColumn.columnName); panel.add(columnLabel,BorderLayout.NORTH); JButton taskButton = new JButton("+"); panel.add(taskButton,BorderLayout.CENTER); taskPanel = drawTasks(userColumn.get(i)); panel.add(taskPanel,BorderLayout.SOUTH); //panel.setVisible(true); columnPanel.add(panel); } return columnPanel; } public JPanel drawTasks(columnModel userColumn) { JPanel taskPanel = new JPanel(); taskPanel.setLayout(new GridBagLayout()); GridBagConstraints gbc = new GridBagConstraints(); int position = 0; for(int i=0;i {
JPanel subTask = new JPanel(); subTask.setLayout(new FlowLayout()); JLabel taskName = new JLabel(userColumn.task.get(i).taskName); JLabel taskDescription = new JLabel(userColumn.task.get(i).taskDescription); subTask.add(taskName); subTask.add(taskDescription); gbc.fill = GridBagConstraints.VERTICAL; gbc.gridx = 0; gbc.gridy = position; taskPanel.add(subTask,gbc); position++; } return taskPanel; } }
Like I said, I have only included the relevant files so let me know if you need more. Thanks.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
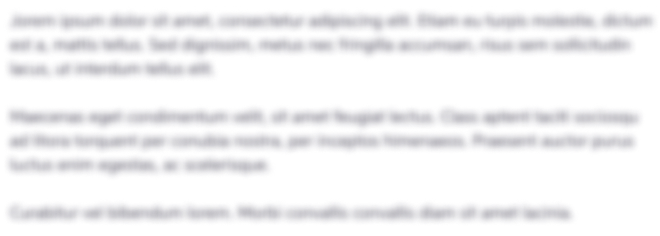
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started