Question
I have done every one except for part i and j. Please help me with just those two parts! Thanks! Write a test program that
I have done every one except for part i and j. Please help me with just those two parts! Thanks!
Write a test program that uses BMat objects to do specified Boolean matrix calculations. The starting version of your program should be the sample file P4Test.java. Instance BMat objects for the matrices A, B, C, D, E, F, and G that are defined in P4Test . Using BMat methods, have your program calculate the following (X is an integer variable, and W is a work matrix):
a. W = (C' (A B)) B' (M' = complement of M)
b. W = (BT B) (C CT)
c. W = C18 = C C ... C (18 C's)
d. W = (D E)T (DT ET)
e. W = D1 D2 D3 D4
f. X = maximum out-degree of all nodes in D
g. W = symmetric closure of D. Is D symmetric?
h. W = transitive closure of E. Is E transitive?
i. Show that matrix F represents a tree (has a candidate root node and has no cycles).
j. Show that matrix G does not represent a tree.
*** This is the Bmat.java given***
// Boolean Matrix Class public class BMat { // Instance variables public int M[][]; public int SIZE;
// Boolean matrix constructors
public BMat(int s) { SIZE = s; M = new int[SIZE][SIZE]; // Fill M with zeros for(int r = 0; r < SIZE; r++){ for(int c = 0; c < SIZE; c++){ M[r][c] = 0; } } }
public BMat(int[][] B) { SIZE = B.length; M = new int[SIZE][SIZE]; // Copy matrix B values into M for(int r = 0; r < SIZE; r++){ for(int c = 0; c < SIZE; c++){ if(B[r][c] == 0) M[r][c] = 0; else M[r][c] = 1; } } }
// Boolean matrix methods
public void show() { // Display matrix for(int r = 0; r < SIZE; r++){ for(int c = 0; c < SIZE; c++){ System.out.print(" " + M[r][c]); } System.out.println(); } return; }
public boolean isEqual(BMat M2) { // Check if current matrix equals matrix M2 for(int r = 0; r < SIZE; r++){ for(int c = 0; c < SIZE; c++){ if(this.M[r][c] != M2.M[r][c]) return false; } } return true; }
public int trace() { // Sum of main diagonal values int diag = 0; for(int r = 0; r < SIZE; r++) diag = diag + M[r][r]; return diag; }
public int arrows() { // No. of 1's in matrix int narrows = 0; for(int r = 0; r < SIZE; r++){ for(int c = 0; c < SIZE; c++){ narrows = narrows + M[r][c]; } } return narrows; }
public int indegree(int K) { // Number of arrows INTO node K of digraph // Nodes are numbered 0,1,2,...,SIZE-1 int colsum = 0; for(int r = 0; r < SIZE; r++) colsum = colsum + M[r][K]; return colsum; }
public BMat complement() { // Logical NOT of current matrix BMat W1 = new BMat(SIZE); for(int r = 0; r < SIZE; r++){ for(int c = 0; c < SIZE; c++){ if(this.M[r][c] == 0) W1.M[r][c] = 1; else W1.M[r][c] = 0; } } return W1; }
public BMat join(BMat M2) { // Logical OR of current matrix with matrix M2 BMat W1 = new BMat(SIZE); for(int r = 0; r < SIZE; r++){ for(int c = 0; c < SIZE; c++){ W1.M[r][c] = this.M[r][c] | M2.M[r][c]; } } return W1; }
public BMat power(int N) { // Raise current matrix to Boolean Nth power (N> = 1) BMat W1 = new BMat(this.M); BMat W2 = new BMat(this.M); for(int k = 2; k <= N; k++){ W1 = W1.product(W2); } return W1; }
public BMat rclosure() { // Reflexive closure of current matrix BMat W1 = new BMat(this.M); // Put 1's on main diagonal for(int r = 0; r < SIZE; r++) W1.M[r][r] = 1; return W1; }
public BMat sclosure() { // Symmetric closure of current matrix BMat W1 = new BMat(this.M); BMat W2 = W1.transpose(); W1 = W1.join(W2); return W1; }
// ********************************* // Project #4 functions to add // *********************************
public int outdegree(int K) { int add = 0; for(int i= 0; i < SIZE; i++) { add = add + M[K][i]; } return add; }
public BMat meet(BMat M2) { BMat W1 = new BMat(SIZE); for(int i = 0; i < SIZE; i++) { for(int x = 0; x< SIZE; x++) { W1.M[i][x] = this.M[i][x] & M2.M[i][x]; } } return W1; }
public BMat transpose() { for(int i = 0; i < SIZE; i++) { for(int x = 0; x < SIZE; x++) { if(i == x) continue; this.M[i][x] = this.M[i][x]; } } return this;
}
public BMat product(BMat M2) { BMat W1 = new BMat(SIZE); for(int r = 0; r < SIZE; r++){ for(int c = 0; c < SIZE; c++){ if(this.M[r][c] == 1 || M2.M[r][c] ==1) { W1.M[r][c] = 1; } else { W1.M[r][c] = 0; } } } return W1; }
public BMat tclosure() { for (int i = 0; i < SIZE; i++) { for (int x = 0; x < SIZE; x++) { if (this.M[x][i]==1) for (int k = 0; k < SIZE; k++) if (this.M[x][i]==1 && this.M[i][k]==1) this.M[x][k] = 1; else this.M[x][k]=0; } } return this; }
public int rootnode() { int norn=0; int node=-1; for (int i = 0; i < SIZE; i++) { int count=0; for (int j = 0; j< SIZE; j++) { if (this.M[j][i]==0) count=count+1; } if (count==SIZE) { norn=norn+1; node=i; } } if (norn==1) return node+1; return -1; }
} // end class
***This is the P4Test.java that the code will be written in***
// Project #4 test program
public class P4Test { public static void main(String[] args) { // Boolean matrix definitions int A[][] = new int[][] {{1, 1, 0, 0, 1}, {1, 0, 1, 0, 0}, {0, 0, 0, 0, 0}, {1, 0, 0, 0, 0}, {0, 0, 1, 0, 1}}; int B[][] = new int[][] {{0, 1, 0, 0, 1}, {0, 1, 1, 0, 0}, {1, 0, 1, 0, 0}, {1, 0, 0, 0, 0}, {0, 1, 0, 0, 1}}; int C[][] = new int[][] {{0, 1, 0, 0, 0}, {0, 0, 1, 0, 0}, {0, 0, 0, 1, 0}, {1, 0, 0, 0, 1}, {0, 1, 0, 0, 0}}; int D[][] = new int[][] {{1, 1, 0, 0, 0, 0}, {1, 1, 1, 0, 0, 0}, {0, 1, 1, 1, 0, 0}, {0, 0, 1, 1, 0, 0}, {0, 0, 0, 0, 0, 1}, {0, 0, 0, 0, 1, 1}}; int E[][] = new int[][] {{0, 1, 1, 0, 0, 1}, {0, 1, 1, 0, 0, 1}, {0, 0, 1, 0, 0, 1}, {0, 0, 0, 0, 1, 1}, {0, 0, 0, 1, 1, 1}, {0, 0, 0, 0, 0, 0}}; int F[][] = new int[][] {{0, 0, 0, 0, 1, 0, 1, 0, 0}, {1, 0, 0, 1, 0, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 0, 0, 0, 0}, {0, 0, 1, 0, 0, 0, 0, 1, 1}, {0, 0, 0, 0, 0, 0, 0, 0, 0}, {0, 1, 0, 0, 0, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 0, 0, 0, 0}}; int G[][] = new int[][] {{0, 0, 0, 1, 0, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 0, 0, 0, 0}, {1, 0, 0, 0, 0, 0, 0, 0, 0}, {0, 0, 1, 0, 0, 1, 0, 0, 0}, {0, 1, 0, 0, 0, 0, 1, 0, 1}, {0, 0, 0, 0, 0, 0, 0, 1, 0}, {0, 0, 0, 0, 0, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 0, 0, 0, 0}}; BMat BMA = new BMat(A); BMat BMB = new BMat(B); BMat BMC = new BMat(C); BMat BMD = new BMat(D); BMat BME = new BMat(E); BMat BMF = new BMat(F); //a BMat Wa = new BMat(BMA.SIZE); Wa = BMA.join(BMB); Wa = BMC.complement().meet(Wa); Wa = Wa.meet(BMB.complement()); System.out.println("Part A:"); Wa.show(); //b BMat Wb = new BMat(BMA.SIZE); BMat Wb1 = new BMat(BMA.SIZE); Wb = BMB.transpose(); Wb = Wb.product(BMB); Wb1 = BMC.join(BMC.transpose()); Wb = Wb.meet(Wb1); System.out.println(); System.out.println("Part B:"); Wb.show(); //c BMat Wc = new BMat(BMC.SIZE); Wc = BMC; for (int i = 1; i <= 18; i++) { Wc = Wc.product(BMC); } System.out.println(); System.out.println("Part C:"); Wc.show(); //d BMat Wd = new BMat(BMD.SIZE); BMat Wd1 = new BMat(BMD.SIZE); Wd = BMD.join(BME); Wd = Wd.transpose(); Wd1 = BMD.transpose().join(BME.transpose()); Wd = Wd.meet(Wd1); System.out.println(); System.out.println("Part D:"); Wd.show(); //e BMat We = new BMat(BMD.SIZE); BMat We1 = new BMat(BMD.SIZE); BMat We2 = new BMat(BMD.SIZE); BMat We3 = new BMat(BMD.SIZE); BMat We4 = new BMat(BMD.SIZE); We1 = BMD.product(BMD); We2 = BMD; for (int i = 1; i <= 2; i++) { We2.product(BMD); } We3 = BMD; for (int i = 1; i <= 3; i++) { We3.product(BMD); } We4 = BMD; for (int i = 1; i <= 4; i++) { We4 = We4.product(BMD); } We = We1.join(We2); We = We.join(We3); We = We.join(We4); System.out.println(); System.out.println("Part E:"); We.show(); //f System.out.println(); int outs[] = new int[BMD.SIZE]; for (int i = 0; i < BMD.SIZE; i++) { outs[i] = BMD.outdegree(i); } int max = outs[0]; for (int i = 1; i < (BMD.SIZE); i++) { if (outs[i] > max) { max = outs[i]; continue; } } System.out.println("Part F:"); System.out.println("The maximum out degree of all the nodes is: " + max); //g System.out.println(); BMat Wg = new BMat(BMD.SIZE); Wg = BMD.sclosure(); Wg.show(); if (Wg.isEqual(BMD)) { System.out.println("Yes, the matrix is symmetric."); } else { System.out.println("No. the matrix is not symmetric."); } //h System.out.println(); System.out.println("Part H:"); BMat Wh = new BMat(BME.SIZE); Wh = BMD.tclosure(); Wh.show(); if (Wh.isEqual(BME)) { System.out.println("Yes, the matrix is transitive."); } else { System.out.println("No. the matrix is not transitive."); } //i System.out.println(Wg.arrows());
//THIS IS WHERE THE CODE NEEDS TO BE PUT FOR I AND J } // end main } // end class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
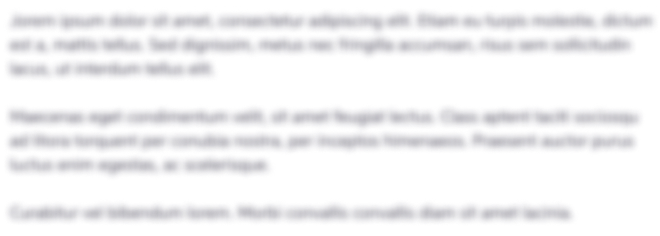
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started