Question
I have the following java class, Date212. Originally it was meant to sort dates from a text file and output the sorted date in the
I have the following java class, Date212. Originally it was meant to sort dates from a text file and output the sorted date in the format October 01, 2014. Which it does, but only for a text file imported to the overall java project. I need this class to be modified to do the same when the text file is selected to be opened from a drop down menu. Thank you for any help.
class Date212
{
/*Establishes the names of the months
*that the number representations of
*the months will be converted to.
*/
private String monthsInYear[]=
{"","January","February",
"March","April","May",
"June","July","August",
"September","October",
"November","December"};
/*All variables set to
* private to prevent
* user tampering.
*/
private int month;
private String monthString; //Needed to convert the number representation of month to the name of the month.
private int day;
private int year;
/*Assigns variables year,
* month, and day.
*/
public Date212 (int y, int m, int d) {
year=y;
month=m;
day=d;
monthString = monthsInYear[m];
}
/*Gets and sets the information
* from the day, month, and year
* sections of the string respectively.
*/
public int getDay() {
return day;
}
public int getMonth() {
return month;
}
public int getYear() {
return year;
}
public void setDay(int d) {
day=d;
}
public void setMonth(int m) {
month=m;
}
public void setYear(int y) {
year=y;
}
public Date212 (String d)
{
/*Calling three argument constructor
* Substring reads the data from the textfile
* and separates them, with years being the first
* 4 numbers, months being the fifth and sixth numbers,
* and days being the seventh and eighth numbers.
*/
this(Integer.parseInt(d.substring(0, 4)), Integer.parseInt(d.substring(4, 6)), Integer.parseInt(d.substring(6, 8)));
}
//Method that returns date in the required format mm dd, yyyy
public String getAsString()
{
return monthString + " " + String.format("%02d", day) + ", " + String.format("%04d", year);
}
/*
* Returns the string representation of the Date212 object
*/
public String toString() {
String result = "";
result += + this.year;
result += this.month < 10 ? "0" + this.month : this.month;
result += this.day < 10 ? "0" + this.day : this.day;
return result;
}
/*
* Override compareTo method
* Comparison of date objects.
* Compares date values to find
* out which is larger for
* sorting
*/
public int compareTo(Date212 other) {
if (this.year < other.year) {
return -1;
} else if (this.year > other.year) {
return 1;
}
if (this.month < other.month) {
return -1;
} else if (this.month > other.month) {
return 1;
}
if (this.day < other.day) {
return -1;
} else if (this.day > other.day) {
return 1;
}
return 0;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
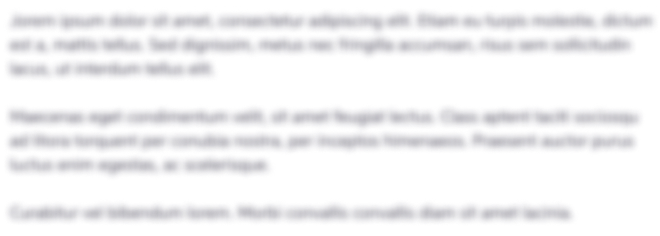
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started