Question
I have the storesim.cpp file and the queuelnk.h file listed below. I need help on this queue c++ check out line simulation program. Help is
I have the storesim.cpp file and the queuelnk.h file listed below. I need help on this queue c++ check out line simulation program. Help is appreciated!
storesim.cpp file
/ Simulates the flow of customers through a line in a store. // Makes use of queues.
#include #include #include #include "queuelnk.h"
using namespace std;
int main () { Queue custQ; // Line (queue) of customers containing the // time that each customer arrived and // joined the line int simLength, // Length of simulation (minutes) minute, // Current minute timeArrived, // Time dequeued customer arrived waitTime, // How long dequeued customer waited totalServed = 0, // Total customers served totalWait = 0, // Total waiting time maxWait = 0, // Longest wait numArrivals = 0;
cout << endl << "Enter the length of time to run the simulator : "; cin >> simLength;
for ( minute = 0 ; minute < simLength ; minute++ ) { if ( !custQ.isEmpty() ) { //* Add code here to do the following: //* 1. dequeue the first customer and capture its return //* in timeArrived //* 2. increment totalServed //* 3. calculate the waitTime of the customer //* 4. add the waitTime of the customer to totalWait //* 5. update maxWait if this customer waited longer than any previous customer. }
switch ( rand() % 4 ) { // The number generated above will be between 0 and 3 // If 0 or 3, then no customers will be added, If 2, then 2 // customers will be added. If 1, then one customer will be added. case 0 : case 3 : numArrivals = 0; break; case 1 : numArrivals = 1; break; case 2 : numArrivals = 2; } for ( int j = 0 ; j < numArrivals ; j++ ) custQ.enqueue(minute); }
cout << endl; cout << "Customers served : " << totalServed << endl; cout << "Average wait : " << setprecision(2) << double(totalWait)/totalServed << endl; cout << "Longest wait : " << maxWait << endl;
return 0; }
queuelnk.h file
#include #include using namespace std;
template < class DT > // Forward declaration of the Stack class class Queue;
template < class DT > class QueueNode // Facilitator class for the Queue class { private: // Constructor QueueNode ( const DT &nodeData, QueueNode *nextPtr );
// Data members DT dataItem; // Queue data item QueueNode *next; // Pointer to the next element
friend class Queue
; };
//--------------------------------------------------------------------
template < class DT > class Queue { public: // Constructor Queue ( int ignored = 0 );
// Destructor ~Queue ();
// Queue manipulation operations void enqueue ( const DT &newData ) ; // Enqueue data element DT dequeue () ; // Dequeue data element void clear (); // Clear queue
// Queue status operations bool isEmpty () const; // Queue is empty bool isFull () const; // Queue is full
// Output the queue structure -- used in testing/debugging void showStructure () const;
private: // Data members QueueNode
*front, // Pointer to the front node *rear; // Pointer to the rear node };
//-------------------------------------------------------------------- // Class implementation of the linked list Queue ADT //--------------------------------------------------------------------
template < class DT > QueueNode
:: QueueNode( const DT &nodeDataItem, QueueNode
*nextPtr )
// Creates a queue node containing data item nodeDataItem and next pointer nextPtr.
: dataItem(nodeDataItem), next(nextPtr) {}
//--------------------------------------------------------------------
template < class DT > Queue
:: Queue ( int ignored )
// Creates an empty queue. Parameter is provided for compatability // with the array implementation and is ignored.
: front(0), rear(0) {}
//--------------------------------------------------------------------
template < class DT > Queue
:: ~Queue ()
// Frees the memory used by a queue.
{ clear(); }
//--------------------------------------------------------------------
template < class DT > void Queue
:: enqueue ( const DT &newDataItem )
// Inserts newElement at the rear of a queue. { QueueNode
*p; // Pointer to enqueued data item
// To be implemented: // 1. make p a new QueueNode that has newDataItem and link of zero // 2. if there are no nodes, set the front to be this new node // 3. otherwise, add the new node to the end // 4. reassign the rear to p }
//--------------------------------------------------------------------
template < class DT > DT Queue
:: dequeue ()
// Removes the least recently (front) element from a queue and returns it. // If the list was empty, throws a logic error.
{ QueueNode
*p; // Pointer to dequeued node DT temp; // Temporarily stores dequeued element
// Requires that the queue is not empty if ( front == 0 ) throw logic_error("Queue
::dequeue : list is empty");
temp = front->dataItem; p = front; front = front->next; if ( front == 0 ) rear = 0; delete p;
return temp; }
//--------------------------------------------------------------------
template < class DT > void Queue
:: clear ()
// Removes all the elements from a queue.
{ QueueNode
*p, // Points to successive nodes *nextP; // Stores pointer to next node p = front; while ( p != 0 ) { nextP = p->next; delete p; p = nextP; }
front = 0; rear = 0; }
//--------------------------------------------------------------------
template < class DT > bool Queue
:: isEmpty () const
// Returns true if a queue is empty. Otherwise, returns false.
{ return ( front == 0 ); }
//--------------------------------------------------------------------
template < class DT > bool Queue
:: isFull () const
// Returns true if a queue is full. Otherwise, returns false.
{ // This is a somewhat hacked way to test if the list is full. // If a node can be successfully allocated than the list is not // full. If the allocation fails it is implied that there is no // more free memory therefore the list is full. QueueNode
*p; DT junk;
try { p = new QueueNode
(junk, 0); } catch ( bad_alloc &e ) { return true; }
delete p; return false; }
//--------------------------------------------------------------------
template < class DT > void Queue
:: showStructure () const
// Linked list implementation. Outputs the elements in a queue. If // the queue is empty, outputs "Empty queue". This operation is // intended for testing and debugging purposes only.
{ QueueNode
*p; // Iterates through the queue
if ( front == 0 ) cout << "Empty queue" << endl; else { cout << "front "; for ( p = front ; p != 0 ; p = p->next ) cout << p->dataItem << " "; cout << "rear" << endl; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
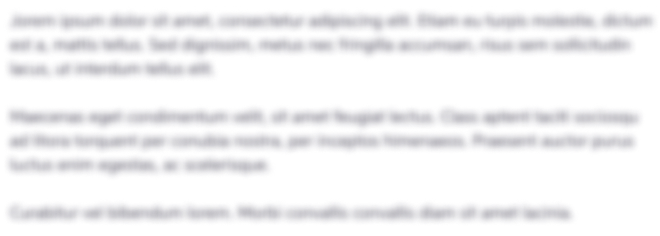
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started