Question
I have this problem in my class: Create a simple GUI application that will obtain from a user their birth date. The program will then
I have this problem in my class:
Create a simple GUI application that will obtain from a user their birth date. The program will then calculate and display the age of the user within a JPanel when the user clicks on a button. The GUI should display the value presented. Ensure that your application includes the following components:
JPanel
JButton
ActionListener
Submit screenshots of your programs execution and output. Include all appropriate source code in a zip file.
This is the code I came up with. I'm wondering if this is correct/what I need to fix with it and why:
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextField;
public class BirthdayFrame extends JFrame {
private JButton button;
private JLabel ageLabel;
private JLabel monthLabel;
private JLabel dayLabel;
private JLabel yearLabel;
private JTextField monthField;
private JTextField dayField;
private JTextField yearField;
private double age;
private static final int FRAME_WIDTH = 500;
private static final int FRAME_HEIGHT = 500;
private static final double TODAYS_MONTH = 7;
private static final double TODAYS_DAY = 11;
private static final double TODAYS_YEAR = 2018;
}
public BirthdayFrame() {
double userAge = age;
createTextFields();
createButton();
createPanel();
setSize(FRAME_WIDTH, FRAME_HEIGHT);
}
Private void createTextFields() {
monthLabel = new JLabel(Birth Month (Number): );
final int FIELD_WIDTH = 2;
monthField = new JTextField(FIELD_WIDTH);
monthField.setEditable(true);
monthField.setText(userMonth);
dayLabel = new JLabel(Birth Day: );
dayField = new JTextField(FIELD_WIDTH);
dayField.setEditable(true);
dayField.setText(userDay);
yearLabel = new JLabel(Birth Year: );
final int YEAR_WIDTH = 4;
yearField = new JTextField(YEAR_WIDTH);
yearField.setEditable(true);
yearField.setText(userYear);
class CalculateBirthdayListener implements ActionListener {
public void actionPerformed(ActionEvent event) {
int userMonth = 0;
int userDay = 0;
int userYear = 0;
userMonth = yearField.getValue();
userDay = dayField.getValue();
userYear = yearField.getValue();
if (userMonth < todaysMonth) {
userAge = todaysYear userYear;
}
else if ((userMonth = todaysMonth) && (userDay < todaysDay)) {
userAge = todaysYear userYear;
}
else {
userAge = (todaysYear userYear) 1;
}
ageLabel.setText(Age: + userAge);
}
}
private void createButton() {
button = new JButton(Calculate Birthday);
ActionListener listener = new CalculateBirthdayListener();
button.addActionListener(listener);
private void createPanel() {
JPanel panel == new JPanel();
panel.add(monthLabel);
panel.add(monthField);
panel.add(dayLabel);
panel.add(dayField);
panel.add(yearLabel);
panel.add(yearField);
panel.add(button);
panel.add(ageLabel);
add(panel);
}
}
import javax.swing.JFrame;
public class BirthdayViewer {
public static void main(String[] args) {
JFrame frame = new BirthdayFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
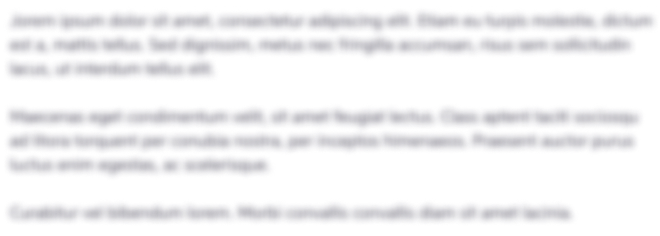
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started