Question
I have this problem that needs to be added to my work, and i have this so far of my problem. I need the function
I have this problem that needs to be added to my work, and i have this so far of my problem. I need the function added and explained how you got to it. Here is the question then the program i have written so far.
Add a function to the Programming Challenge that allows the user to search the structure array for a particular customers account. It should accept part of the customers name as an argument then search for an account with a name that matches it. All accounts that match should be displayed. If no account matches, a message saying so should be displayed.
#include
#include
using namespace std;
struct account{
string userName;
string address;
string city;
string state;
string zip;
string telephone;
string date;
double balance;
string dateOfLastPay;
};
void showMainMenu(const unsigned);
void showModifyMenu();
void addAccount(account[], const unsigned, unsigned&);
void modifyAccount(account[], const unsigned);
void displayAccounts(account[], const unsigned);
void setName(account&);
void setAddress(account&);
void setCity(account&);
void setState(account&);
void setZIP(account&);
void setTelephone(account&);
void setDate(account&);
void setDateOfLastPay(account&);
void setBalance(account&);
string getOrdinal(const unsigned);
bool isEmpty(const string);
int main(){
const unsigned MAX = 10;
account customers[MAX];
unsigned firstAvailableIndex = 0;
int choice = 0;
do{
showMainMenu(firstAvailableIndex);
cout << "You choose: ";
cin >> choice;
cin.ignore();
if(choice == 1)
addAccount(customers, MAX, firstAvailableIndex);
else if(choice == 2)
modifyAccount(customers, firstAvailableIndex);
else if (choice == 3)
displayAccounts(customers, firstAvailableIndex);
else if(choice != 0){
cout << "No choice value, Enter a valid choice! ";
}
}
while(choice != 0);
cout << "Thank you for exploring my program.";
return 0;
}
void showMainMenu(const unsigned numCustomers){
cout << "1.) Add a new Account. ";
cout << "2.) Modify existing account. ";
cout << "3.) Display all the data stored. ";
cout << "0.) Exit. ";
cout << "(Current number of accounts: " << numCustomers << " )";
cout << endl;
}
void showModifyMenu(){
cout << "1.) Modify account user name. ";
cout << "2.) Modify account address. ";
cout << "3.) Modify account city location ";
cout << "4.) Modify account state location. ";
cout << "5.) Modify account ZIP code location. ";
cout << "6.) Modify account phone number. ";
cout << "7.) Modify account balance. ";
cout << "8.) Modify date of the last payment. ";
cout << "9.) Exit. ";
}
void addAccount(account customers[], const unsigned SIZE, unsigned& index){
if( index >= SIZE){
cout << "Array is full. ";
cout << "(Current number of account: " << index << " ) ";
return;
}
cout << "The info about " << (index + 1) << getOrdinal(index + 1) << "account, ";
setName (customers[index]);
setAddress (customers[index]);
setCity (customers[index]);
setState (customers[index]);
setZIP (customers[index]);
setTelephone (customers[index]);
setBalance (customers[index]);
setDateOfLastPay (customers[index]);
index ++;
cout << "Account number (" << index << ") was stored ";
}
void modifyAccount(account customers[],
const unsigned NUM_ACCOUNTS){
if(NUM_ACCOUNTS == 0){
cout << "Cannot modify any account. ";
cout << "Array is empty. ";
cout << "(Current number of accounts: " << NUM_ACCOUNTS << " ) ";
return;
}
int account = -1;
int choice = 0;
cout << "Enter the account number, you want to modify. ";
cout << " No 0 or negative numbers are allowed. ";
cout << "(Current number of accounts: " << NUM_ACCOUNTS << " ) ";
cout << endl;
cout << "Your account: ";
cin >> account;
while (account < 1 || account > NUM_ACCOUNTS){
cout << "NO 0 or negative numbers! ";
cout << "(Current number of accounts: " << NUM_ACCOUNTS << " ) ";
cout << "You chose: ";
cin >> account;
}
cout << "Choose your option, ";
account --;
do{
showModifyMenu();
cout << "Your choice: ";
cin >> choice;
cin.ignore();
if (choice == 1){
cout << "You chose the user name of ";
cout << (account + 1) << getOrdinal(account + 1) << " account, ";
cout << "Old value: " << customers[account].userName
<< endl;
cout << "Enter the new value, "; setName(customers[account]);
}
else if(choice == 2){
cout << "You chose the address of ";
cout << (account + 1) << getOrdinal (account + 1) << "account, ";
cout << "Old value: " << customers[account].address
<< endl;
cout << "Enter the new value, "; setAddress(customers[account]);
}
else if(choice == 3){
cout << "You chose the city of ";
cout << (account + 1) << getOrdinal (account + 1) << "account, ";
cout << "Old value: " << customers[account].city
<< endl;
cout << "Enter the new value, "; setCity(customers[account]);
}
else if(choice == 4){
cout << "You chose the state of ";
cout << (account + 1) << getOrdinal (account + 1) << "account, ";
cout << "Old value: " << customers[account].address
<< endl;
cout << "Enter the new value, "; setState(customers[account]);
}
else if(choice == 5){
cout << "You chose the ZIP code of ";
cout << (account + 1) << getOrdinal (account + 1) << "account, ";
cout << "Old value: " << customers[account].zip
<< endl;
cout << "Enter the new value, "; setZIP(customers[account]);
}
else if(choice == 6){
cout << "You chose the phone number of ";
cout << (account + 1) << getOrdinal (account + 1) << "account, ";
cout << "Old value: " << customers[account].telephone
<< endl;
cout << "Enter the new value, "; setTelephone(customers[account]);
}
else if(choice == 7){
cout << "You chose the balance of ";
cout << (account + 1) << getOrdinal (account + 1) << "account, ";
cout << "Old value: " << customers[account].balance
<< endl;
cout << "Enter the new value, "; setBalance(customers[account]);
}
else if(choice == 8){
cout << "You chose the last payment of ";
cout << (account + 1) << getOrdinal (account + 1) << "account, ";
cout << "Old value: " << customers[account].dateOfLastPay
<< endl;
cout << "Enter the new value, "; setDateOfLastPay(customers[account]);
}
else if(choice != 0){
cout << "Wrong value, enter a valid value! ";
}
}
while(choice != 0);
cout << "You will exit the modifcation mode. ";
}
void displayAccounts(account customers[], const unsigned NUM_ACCOUNTS){
if(NUM_ACCOUNTS == 0){
cout << "Array is empty. ";
cout << "(Current number of accounts: " << NUM_ACCOUNTS << " ) ";
return;
}
for(unsigned i = 0; i < NUM_ACCOUNTS; i++){
cout << "The info about " << (i + 1) << getOrdinal (i + 1) << " account, ";
cout << "User Name: " << customers[i].userName << endl;
cout << "Address: " << customers[i].address << endl;
cout << "City: " << customers[i].city << endl;
cout << "State: " << customers[i].state << endl;
cout << "ZIP code: " << customers[i].zip << endl;
cout << "Telephone: " << customers[i].telephone << endl;
cout << "Balance: " << customers[i].balance << endl;
cout << "Date of last Pay: " << customers[i].dateOfLastPay << endl;
if(i < (NUM_ACCOUNTS - 1))
cout << "-------------------- ";
}
cout << "================= ";
}
void setName(account& account){
cout << "User name: ";
getline(cin, account.userName);
while (isEmpty(account.userName)){
cout << "Name field cannot be empty! ";
cout << "User name: ";
getline(cin, account.userName);
}
}
void setAddress(account& account){
cout << "Address: ";
getline(cin, account.address);
while (isEmpty(account.address)){
cout << "Address field cannot be empty! ";
cout << "Address: ";
getline(cin, account.address);
}
}
void setCity(account& account){
cout << "City: ";
getline(cin, account.city);
while (isEmpty(account.city)){
cout << "City field cannot be empty! ";
cout << "City: ";
getline(cin, account.city);
}
}
void setState(account& account){
cout << "State: ";
getline(cin, account.state);
while (isEmpty(account.state)){
cout << "State field cannot be empty! ";
cout << "State: ";
getline(cin, account.state);
}
}
void setZIP(account& account){
cout << "ZIP code: ";
getline(cin, account.zip);
while (isEmpty(account.zip)){
cout << "ZIP code field cannot be empty! ";
cout << "ZIP code: ";
getline(cin, account.zip);
}
}
void setTelephone(account& account){
cout << "Phone Number: ";
getline(cin, account.telephone);
while (isEmpty(account.telephone)){
cout << "Phone number field cannot be empty! ";
cout << "Phone number: ";
getline(cin, account.telephone);
}
}
void setBalance(account& account){
cout << "Balance: ";
cin >> account.balance;
while (account.balance < 0){
cout << "Balance field cannot be negative! ";
cout << "Balance: ";
cin >> account.balance;
}
cin.ignore();
}
void setDateOfLastPay(account& account){
cout << "Date of last payment: ";
getline(cin, account.city);
while (isEmpty(account.dateOfLastPay)){
cout << "Date of last payment field cannot be empty! ";
cout << "Date of last payment: ";
getline(cin, account.dateOfLastPay);
}
}
bool isEmpty(const string str){
if (str.empty())
return true;
unsigned len = str.length();
for(unsigned i = 0; i < len; i++){
if(!isspace(str[i]))
return false;
}
return true;
}
string getOrdinal(const unsigned num){
unsigned parser = num; 100;
if (parser < 20 && parser > 10){
return "th";
}
parser = num; 10;
if(parser == 1){
return "st";
}
else if(parser == 2){
return "nd";
}
else if(parser == 3){
return "rd";
}
else {
return "th";
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
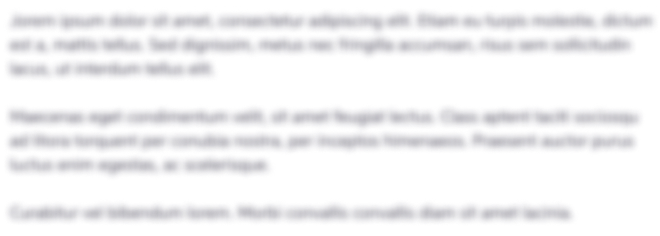
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started