Question
I have to write a class called Set. I started but I need help, any methods that you can add or any suggestion. Below are
I have to write a class called Set. I started but I need help, any methods that you can add or any suggestion.
Below are instructions;
The Set class will contain the following features;
Private Instance Variables
int[] set - stores the elements of the set
int size contains the number of elements in the set.
Note that 0 <= size <= set.length Public Constructor Set(int capacity) allocates memory for the set array. The array length = capacity. Private Method
void increaseCapacity this methods doubles the length of the set array without losing any of the values stored in the array. Example: If set = {8, 2, 1} before calling increaseCapacity then set = {8, 2, 1, 0, 0, 0} after calling increaseCapacity. Public Methods
void add(int value) if value is not contained in the set then perform assign value to set[size] and then increment size by 1. If size = set.length then call the increaseCapacity method to increase the size of the set array before adding any values to the set. boolean contains(int value) returns true if the value is contained in the set; otherwise return false. Use a while loop to determine if a value is in array. Stop iteration if you determine the value is in the array. Do not use a break statement. int size() return the value of size
void clear() allocates a new array of length 10 to set; sets size to 0 boolean remove(int value) if value is not in the set then return false; if value is contained in the set then remove this value from the set with the following algorithm: if the value is stored at index k then shift the values stored in indices k + 1 to size 1 to the left and decrease size by 1. Make sure to use System.arraycopy to shift array elements to the left.
String toString() returns a String representation of the set. Example: suppose the array contains the value 4, 8, and 9. The toString method returns the String {4, 8, 9}. If the set is empty return the String {}.
----------------------------------------------------
This is the code that I started and should be continued;
public class Set {
private int size;
private Set[] set;
public Set(int capacity) {
set = new Set[capacity];
size = capacity;}
private void increaseCapacity() {
for (int i = 0; i < 20; i++);}
public void add(int value) {
set[size] = value;
if (size == set.length)
increaseCapacity();
else
size++;}}
--------------------------------------------------------------
Test code for the class Set;
public class Test { // 2-pts
public static void main(String[] args) {
boolean flag;
Set s = new Set(8);
s.add(4);
System.out.println("Size of the set: " + s.size());
s.add(100);
System.out.println("Size of the set: " + s.size());
s.add(23);
System.out.println("Size of the set: " + s.size());
System.out.println("Contents of the set: " + s);
flag = s.remove(2);
if (flag) {
System.out.println("An element was removed from the set");
} else {
System.out.println("An element was not removed from the set");
}
System.out.println();
s.clear();
System.out.println("Contents of the set: " + s);
System.out.println();
s.add(50);
s.add(24);
s.add(101);
s.add(45);
System.out.println("Size of the set: " + s.size());
System.out.println("Contents of the set: " + s);
System.out.println();
flag = s.remove(50);
if (flag) {
System.out.println("An element was removed from the set");
} else {
System.out.println("An element was not removed from the set");
}
System.out.println("Size of the set: " + s.size());
System.out.println("Contents of the set: " + s);
System.out.println();
s.clear();
for (int i = 0; i < 20; i++) {
s.add(i);
}
System.out.println("Size of the set: " + s.size());
System.out.println("Contents of the set: " + s);
System.out.println();
s.add(10);
System.out.println("Size of the set: " + s.size());
System.out.println("Contents of the set: " + s);}}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
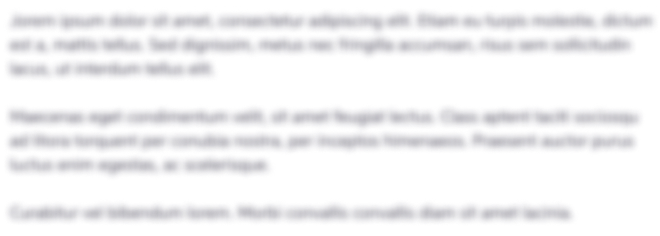
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started