Question
I have tried this code but it just doesn't work, can someone help me find out what is wrong with it. Given the node class
I have tried this code but it just doesn't work, can someone help me find out what is wrong with it.
Given the "node" class below, implement the functions in the linked_list class to provide a singly linked list of nodes. Use the main program included below to test your program. This will allow you/me to run different sets of data against your program without your code needing to be changed/recompiled, etc. Do not modify my code, except perhaps to temporarily comment out parts of it that you have not yet supported. Put your source code in ~/PF3/lab2/lab2.cpp
#include
using namespace std;
class node { public: typedef int data_t;
node* next; data_t data;
node(data_t d) { next = NULL; data = d; } };
class linked_list { private: node* head;
public: linked_list() { head = NULL; }
int size() { node* tptr = head;
int c = 0;
while (tptr) { c++; tptr = tptr->next; }
return c; }
void add(int n, node::data_t d) // code to add element at given index { node *pre=new node; node *cur=new node; node *temp=new node; cur=head; for(int i=1;i
}
void add_last(node::data_t d) { node* tptr = head;
if (!head) { head = new node(d); return; } while (tptr->next) { tptr = tptr->next; }
tptr->next = new node(d); }
void add_first(node::data_t d) { node* newptr = new node(d);
newptr->next = head;
head = newptr; } node::data_t get(int n);
node::data_t get_first();
node::data_t get_last(); { node* tptr = head;
if (head) }
void remove(int n) // delete node at nth index { node *current=new node; node *previous=new node; current=head; for(int i=1;i
void remove_first() // delete from first { node *temp=new node; temp=head; head=head->next; delete temp; }
void remove_last() // delete from last { node *current=new node; node *previous=new node; current=head; while(current->next!=NULL) { previous=current; current=current->next; } tail=previous; previous->next=NULL; delete current; }
int get(int n) // find element at given index { struct Node* current = head; int count = 0; while (current != NULL) { if (count == n) return(current->data); count++; current = current->next; } assert(0); } int get_first(); // find head of list { return head->data; } int get_last(); // find tail of list { struct Node* temp = head; if (temp == NULL) return -1; while (temp != NULL) { if (temp->next==NULL) return temp->data; temp = temp->next; } }
void dump() { node* tptr;
cout << " DUMP: (size = " << size() << ", first = " << get_first() << ", last = " << get_last() << ") ";
if (head == NULL) { cout << " DUMP: head = NULL "; return; }
tptr = head;
while (tptr) { cout << " DUMP: data = : " << tptr->data << endl; tptr = tptr->next; } cout << endl; } };
int main(void) { linked_list ll; string cmd; int i, d;
while (cin >> cmd >> i >> d) { cout << "MAIN: cmd = " << cmd << ", i = " << i << ", d = " << d << endl;
if (cmd == "add") ll.add(i, d); else if (cmd == "addf") ll.add_first(d); else if (cmd == "addl") ll.add_last(d); else if (cmd == "rem") ll.remove(i); else if (cmd == "remf") ll.remove_first(); else if (cmd == "reml") ll.remove_last(); else if (cmd == "get") { d = ll.get(i); cout << "get returns: " << d << endl; } else if (cmd == "getf") { d = ll.get_first(); cout << "getf returns: " << d << endl; } else if (cmd == "getl") { d = ll.get_last(); cout << "getl returns: " << d << endl; } else if (cmd == "dump") ll.dump(); else if (cmd == "quit") exit(0); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
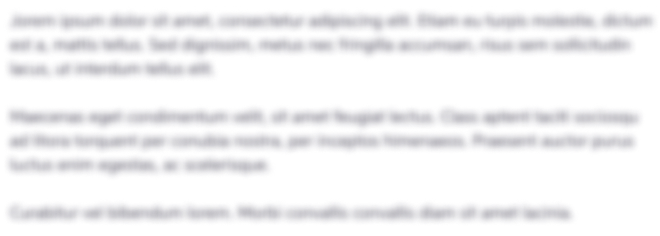
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started