Question
I keep getting an error code in my C++ program. It says [Error] 'strlen' was not declared in this scope in my main.cpp. Here are
I keep getting an error code in my C++ program. It says "[Error] 'strlen' was not declared in this scope" in my main.cpp. Here are my codes.
main.cpp
#include
#include
#include "functions.h"
using namespace std;
//definition of the main function.
//takes arguments from the command-line.
int main(int argc, char *argv[])
{
//Determine if you have enough arguments.
//If not, output a usage message and exit program
if (argc<2 || (argc == 2 && argv[1][0] == '-'))
{
//call the function printUsageInfo()
//with the program name
printUsageInfo("palindrome");
exit(0);
}
//declare the variables.
bool c = true, s = true;
//Loop through remaining arguments which are all input strings :
for (int i = 1; i < argc; i++)
{
//check whether the input has '-','c', 's' ,or both
if (argv[i][0] == '-')
{
if (strlen(argv[i]) == 2)
{
if (argv[i][1] == 's' || argv[i][1] == 'S')
s = false;
else if (argv[i][1] == 'c' || argv[i][1] == 'C')
c = false;
}
else
{
if (argv[i][1] == 's' || argv[i][1] == 'S' || argv[i][2] == 's' || argv[i][2] == 'S')
s = false;
if (argv[i][1] == 'c' || argv[i][1] == 'C' || argv[i][2] == 'c' || argv[i][2] == 'C')
c = false;
}
}
//Process each by calling isPalindrome function with flag values.
else
{
if (isPalindrome(argv[i], c, s))
cout << """ << argv[i] << "" is a palindrome. " << endl;
else
cout << """ << argv[i] << "" is not a palindrome. " << endl;
}
}
return 0;
}
functions.cpp
#include
void BackwardsAlphabet(char currLetter){ if (currLetter == 'a') { cout << currLetter << endl; } else{ cout << currLetter << " "; BackwardsAlphabet(currLetter - 1); } }
int main() { char startingLetter;
startingLetter = 'z'; BackwardsAlphabet(startingLetter);
return 0; }
functions.h
#ifndef FUNCTIONS_H
#define FUNCTIONS_H
#include
#include
using namespace std;
//declare the functions
bool isPalindrome(char str[], bool c, bool s);
bool checkPalandrome(char str[], int start, int end, bool c, bool s);
void printUsageInfo(string name);
#endif
Here are the directions for the assignment:
Background
Palindromes are character sequences that read the same forward or backword (e.g. the strings "mom" or "123 454 321"). Punctuation and spaces are frequently ignored so that phrases like "Dog, as a devil deified, lived as a god." are palindromes. Conversely, even if spaces are not ignored phrases like "Rats live on no evil star" are still palindromes.
Requirements
Command Line Parameters
The program name will be followed by a list of strings. The program will determine whether each string is a palindrome and output the results. Punctuation will always be ignored. An optional flag can preceed a term that modifies how the palindrome is determined.
Strings
Each string will be separated by a space on the command line. If you want to include a string that has spaces in it (e.g. "Rats live on no evil star"), then put quotes around it. The quote characters will not be part of the string that is read in.
Flags
Optional for the user
If present, flags always appear after the program name and before any strings are processed and apply to all subsequent strings processed.
Must start with a minus (-) sign followed by one or two flag values that can be capital or lower case. e.g. -c,-S, -Cs, -Sc, etc.
There are no spaces between starting minus (-) sign and flag(s).
Flags values are case insensitive.
c or C: Indicates that comparisons should be case-sensitive for all input strings. The default condition (i.e. if the flag is NOT included) is to ignore case-sensitivity. So, for example:
palindrome Mom should evalate as being a palindrome.
palindrome -c Mom should not evalate as being a palindrome.
s or S: Indicates that comparisons should not ignore spaces for all input strings. The default condition (i.e. if the flag is NOT included) is to ignore spaces. So, for example:
palindrome "A nut for a jar of tuna" should evalate as being a palindrome.
palindrome -s "A nut for a jar of tuna" shouldnot evalate as being a palindrome.
Options can appear in different flags, e.g. you can use -Sc or-S -c
The same flag cannot appear more than one time prior to a term. If it does, print a usage statement and exit program.
Code Expectations
Your program should only get user input from the command line. (i.e. "cin" should not be anywhere in your code).
Required Functions:Function that prints program usage message in case no input strings were found at command line.
Name: printUsageInfo
Parameter(s): a string representing the name of the executable from the command line.
Return: void.
Recursive function that determines whether a string is a character-unit palindrome.
Name: isPalindrome
Parameter(s): an input string, a boolean flag that considers case-sensitivity when true, and a boolean flag that does not ignore spaces when true.
Return: bool.
All functions should be placed in a separate file following the code organization conventions we covered.
Program Flow
Your program will take arguments from the command-line.
Determine if you have enough arguments. If not, output a usage message and exit program.
If flags are present.
Process and set values to use when processing a palindrome.
Loop through remaining arguments which are all input strings:
Process each by calling isPalindrome function with flag values.
Output results
Program Flow Notes:
Any time you encounter a syntax error, you should print a usage message and exit the program immediately.
Hints
Remember rules for a good recursive function.
Recommended Functions to write: Note: You could combine these into a single function. e.g. "preprocessString"tolower - convert each letter to lowercase version for case insensitive comparisons.
Parameter(s): a string to be converted to lower case
Return: a string with all lower case characters
removePunctuation - Remove all punctuation marks possibly including spaces.
Parameter(s): a string and a boolean flag indicating whether to also remove spaces
Return: a string with punctuation/spaces removed
Existing functions that might help:
tolower
erase
substr
isalnum
Example Output
Note: you will probably only see the ./ if running on build.tamu.edu.
./palindrome Usage: ./palindrome [-c] [-s] string ... -c: case sensitivity turned on -s: ignoring spaces turned off ./palindrome -c Usage: ./palindrome [-c] [-s] string ... -c: case sensitivity turned on -s: ignoring spaces turned off ./palindrome Kayak "Kayak" is a palindrome ./palindrome -c Kayak "Kayak" is not a palindrome. ./palindrome -C Kayak "Kayak" is not a palindrome. ./palindrome -c kayak "kayak" is a palindrome. ./palindrome "Test Set" "Test Set" is a palindrome. ./palindrome -sc "Test Set" "Test Set" is not a palindrome. ./palindrome -s -c "Test Set" "Test Set" is not a palindrome. ./palindrome -s -s "Test Set" "Test Set" is not a palindrome. ./palindrome -scs "Test Set" "Test Set" is not a palindrome. ./palindrome Kayak madam "Test Set" "Evil Olive" "test set" "loop pool" "Kayak" is a palindrome. "madam" is a palindrome. "Test Set" is a palindrome. "Evil Olive" is a palindrome. "test set" is a palindrome. "loop pool" is a palindrome. ./palindrome -s Kayak madam "Test Set" "Evil Olive" "test set" "loop pool" "Kayak" is a palindrome. "madam" is a palindrome. "Test Set" is not a palindrome. "Evil Olive" is not a palindrome. "test set" is not a palindrome. "loop pool" is a palindrome. ./palindrome -c Kayak madam "Test Set" "Evil Olive" "test set" "loop pool" "Kayak" is not a palindrome. "madam" is a palindrome. "Test Set" is not a palindrome. "Evil Olive" is not a palindrome. "test set" is a palindrome. "loop pool" is a palindrome. ./palindrome -c -s Kayak madam "Test Set" "Evil Olive" "test set" "loop pool" "Kayak" is not a palindrome. "madam" is a palindrome. "Test Set" is not a palindrome. "Evil Olive" is not a palindrome. "test set" is not a palindrome. "loop pool" is a palindrome.
Sorry for the extra info, thank you in advance!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
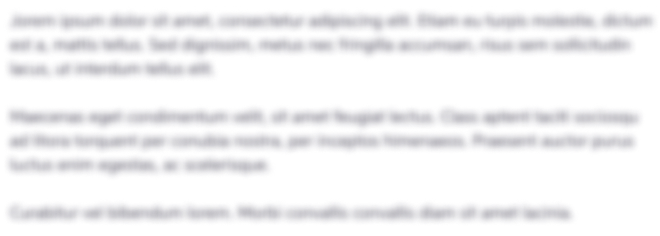
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started