Question
I need a flowchart GeometricObject.java import java.util.Scanner; abstract class GeometricObject { private String color = white; private boolean filled; private java.util.Date dateCreated; /** Construct a
I need a flowchart
GeometricObject.java
import java.util.Scanner;
abstract class GeometricObject { private String color = "white"; private boolean filled; private java.util.Date dateCreated;
/** Construct a default geometric object */ protected GeometricObject() { }
/** Construct a geometric object with color and filled value */ protected GeometricObject(String color, boolean filled) { dateCreated = new java.util.Date(); this.color = color; this.filled = filled; }
/** Return color */ public String getColor() { return color; }
/** Set a new color */ public void setColor(String color) { this.color = color; }
/** Return filled. Since filled is boolean , * the get method is named isFilled */ public boolean isFilled() { return filled; }
/** Set a new filled */ public void setFilled(boolean filled) { this.filled = filled; }
/** Get dateCreated */ public java.util.Date getDateCreated() { return dateCreated; }
@Override public String toString() { return "created on " + dateCreated + " color: " + color + " and filled: " + filled; }
/** Abstract method getArea */ public abstract double getArea();
/** Abstract method getPerimeter */ public abstract double getPerimeter(); }
Triangle.java
import java.util.*;
public class Triangle extends GeometricObject { //variable for sides of triangle private double side1; private double side2; private double side3; //default constructor public Triangle() { side1=1.0; side2=1.0; side3=1.0; } //constructor with parameters public Triangle(double side1,double side2,double side3,String color,boolean filled) { //calling superclass constructor super(color,filled); this.side1=side1; this.side2=side2; this.side3=side3; } //method to set side1 public void setSide1(double side1) { this.side1=side1; } //method to set side2 public void setSide2(double side2) { this.side2=side2; } //method to set side3 public void setSide3(double side3) { this.side3=side3; } //method to get side1 public double getSide1() { return side1; } //method to get side2 public double getSide2() { return side2; } //method to get side3 public double getSide3() { return side3; } //method to get area public double getArea() { double p; double area; p=getPerimeter()/2; //calculating area of the triangle area=Math.sqrt(p*(p-side1)*(p-side2)*(p-side3)); return area; } //method to get perimeter public double getPerimeter() { return (side1+side2+side3); } //method to get string representation of the object public String toString() { return "Area: "+String.format("%.1f", getArea())+" "+ "Perimeter: "+String.format("%.1f", getPerimeter())+" "+ "Color: "+getColor()+" "+ "Filled?: "+isFilled(); } }
TriangleDriver.java
import java.util.*;
//Driver for Triangle class public class TriangleDriver { //main method public static void main(String args[]) { //scanner object Scanner ob=new Scanner(System.in); //variables to store the sides of triangle double side1,side2,side3; //getting the sides of the triangle System.out.print("Enter side1 of triangle: "); side1=ob.nextDouble(); System.out.print("Enter side2 of triangle: "); side2=ob.nextDouble(); System.out.print("Enter side3 of triangle: "); side3=ob.nextDouble(); //getting the color of the triangle System.out.print("Enter color of triangle: "); String color=ob.next(); //getting filled or not System.out.print("Enter whether triangle is filled(yes/no): "); String choice=ob.next(); // converting choice to lowercase choice=choice.toLowerCase(); boolean filled=false; // checking yes or not if (choice.equals("yes")) filled=true; if (choice.equals("no")) filled=false; //creating Triangle object Triangle t1=new Triangle(side1,side2,side3,color,filled); //printing the triangle details System.out.println(t1); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
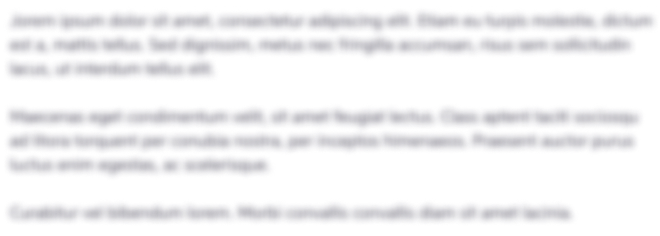
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started