Question
##I NEED HELP CODING THIS JAVA ASSIGNMENT. STUDENT IMPLEMENTATION IS WITHIN THE CODE public DoubleArrayBag clone( ) { // Clone an DoubleArrayBag object. DoubleArrayBag answer;
##I NEED HELP CODING THIS JAVA ASSIGNMENT. STUDENT IMPLEMENTATION IS WITHIN THE CODE
public DoubleArrayBag clone( ) { // Clone an DoubleArrayBag object. DoubleArrayBag answer; try { answer = (DoubleArrayBag) super.clone( ); answer.data = data.clone( ); return answer; } catch (CloneNotSupportedException e) { // This exception should not occur. But if it does, it would probably // indicate a programming error that made super.clone unavailable. // The most common error would be forgetting the "Implements Cloneable" // clause at the start of this class. throw new RuntimeException ("This class does not implement Cloneable"); } } /** * Compare this DoubleArrayBag to another object for equality of value * @param other * reference to another DoubleArrayBag * * @post. * x.equals(x) is true * if x.equals(y) then y.equals(x) * x.equals(null) is false * * @return * true if number of elements in this and other are the same AND if * the values of all the elements in the bag are the same and in the * same position in the bag * * @note. * If the value of other is null, then the return is false **/ @Override public boolean equals(Object other) { if ( other == null ) return false; boolean isEqual = false; if ( other instanceof DoubleArrayBag ) { DoubleArrayBag candidate = (DoubleArrayBag) other; //are we comparing this with this if (this == other) return true; if ( candidate.used != this.used ) return false; if ( candidate.hashCode() != this.hashCode() ) return false; int index = 0; isEqual = true; while ( isEqual && index < this.used ) { if ( this.data[ index ] != candidate.data[ index ] ) isEqual = false; else index++; } } return isEqual; } /** * Potentially increase capacity of this bag * * @pre. * newCapacity must be greater than 0 * * @post. * The bag's capacity is at least newCapacity. If the capacity * was already at or greater than newCapacity, then the capacity * is left unchanged. * * @param * newCapacity an integer greater than 0 * * @exception * OutOfMemoryError if not enough dynamic available to allocate * additional space * @exception * IllegalArgumentException if newCapacity not greater than 0 * **/ public void ensureCapacity( int newCapacity ) { if ( newCapacity < 1) throw new IllegalArgumentException("newCapacity < 1"); if ( this.data.length < newCapacity ) { //STUDENT WORK GOES HERE } } /** * returns the current capacity of the bag * @post. * This method does not alter state of the bag * @return * an int that represents total capacity of this bag **/ public int getCapacity( ) { return this.data.length; } /** * @return the hash code for this object * */ @Override public int hashCode( ) { int usedHashValue = Integer.hashCode( this.used ); int dataHashValue = 0; for ( int i = 0; i < used; ++i ) { dataHashValue += Double.hashCode( this.data[i] ); } return usedHashValue + dataHashValue; } /** * Erases all copies of a specified element from this bag if target exists in bag. * @param target * the element(s) to remove from the bag * @post. * If target was found in the bag, then all copies of * target have been removed and the method returns number of items removed. * @return * int value from 0 to number of items erased from bag **/ public int erase( double target ) { int count = 0; int index = 0; while ( index < used ) { boolean found = false; while ( !found && index < this.used ) { if ( target == this.data[ index ] ) found = true; else ++index; } if (found) { this.data[ index ] = this.data[ --this.used ]; ++count; } } return count; } /** * Remove one copy of a specified element from this bag. * @param target * the element to remove from the bag * @post. * If target was found in the bag, then one copy of * target has been removed and the method returns true. * Otherwise the bag remains unchanged and the method returns false. * @return * true or false depending on whether target exists in the bag **/ public boolean erase_one(double target) { int index = 0; // The location of target in the data array. boolean found = false; while (!found && index < this.used) { if ( target == this.data[index] ) found = true; else ++index; } if (found) this.data[index] = this.data[--this.used]; return found; } /** * Add a new element to this bag doubling capacity if needed * * @param newItem * the new element that is being inserted * @post. * A new copy of the element has been added to this bag. **/ public void insert(double newItem) { if ( used == this.data.length ) { this.ensureCapacity( this.data.length * 2 ); } this.data[ used++ ] = newItem; } /** * Add the contents of another bag to this bag. * @param addend * a bag whose contents will be added to this bag * @pre. * The parameter, addend, is not null. * @post. * The elements from addend have been added to this bag. * @exception NullPointerException * Indicates that addend is null. * @exception OutOfMemoryError * Indicates insufficient memory to add addend to this bag **/ public void plusEquals(DoubleArrayBag addend) { if ( addend == null ) { throw new NullPointerException("Cannot add from null object"); } //STUDENT WORK GOES HERE }
** * Determine the number of elements in this bag. * @return * the number of elements in this bag * @post. * the bag is not altered by this method **/ public int size( ) { return used; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
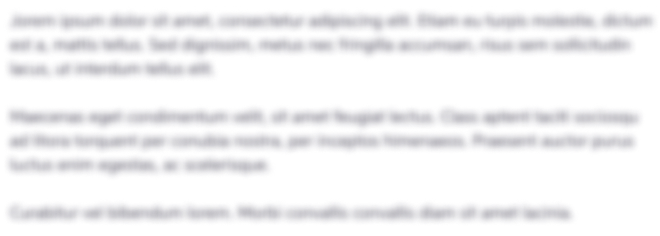
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started