Question
I need help completing this program to not use too much advandce coding, Anyways the program is to make a simple calendar like is shown
I need help completing this program to not use too much advandce coding, Anyways the program is to make a simple calendar like is shown below also are instructions below.
The calendars size should be scalable using class constants. You have some creative freedom with creating the calendar. What characters you use for the outline of the calendar and where you place the date in each calendar cell is up to you. An example of what a calendar might look like with a size of 10 after completing this assignment is below:
Your program should produce output similar to this if the size is 10. , as stated above, you have the freedom to choose what characters you use for the calendar (the | and = in the example above) and the exact location of the number in each cell. Below is an example of a calendar produced with a size of 5:
For now, you may have every month that the calendar displays have 35 days instead of the actual number. We will change that in a later part of the assignment. Upon executing your program, the console should ask what date you would like to look at, as seen in the example above. The use should be able to input a date. The month of that date should be displayed at the top of the calendar. The month and day input by the user should be broken up and displayed at the bottom of the calendar as shown above.
After asking the user for a date and printing the calendar for that date, your program should print a calendar for todays date followed by the month and day broken up as as seen in the image above.
Ultimately, after execution, your calendar should display a prompt to get a date from the user, a calendar for that month from the user input, the month and day input by the user broken up, a second calendar for the current month, and finally the month and day for the current day broken up.
Implementation Details:
You program must include the following methods:
public static void drawMonth(int month)
This method takes in an integer representing the month and displays the month and a graphical representation of the calendar as seen in the examples above.
public static void drawRow(int row)
This method should be called in your drawCalendar method. It should display one week on the calendar (one row). This method is passed an integer representing which row it is displaying.
public static void displayDate(int month, int day)
This method is passed the month and the day as integer values and displays the date information as seen in the above example underneath the graphical representation of the calendar.
public static int monthFromDate(String date)
This method should extract an integer value for the month and return it when passed a given date as a String. Using the split function may be useful for the operation of this function.
public static int dayFromDate(String date)
This method should extract an integer value for the day and return it when passed a given date as a String. Using the split function may be useful for the operation of this function.
Helpful Information:
For this assignment, you may want to use a few tools that we have not explicitly gone over in class.
In order to get todays date, you will likely want to make use of a Calendar object that is already implemented by Java. This object stores basic information that we can access. To use this object, you will want to create a new Calendar object (be careful that your class is also not named Calendar or Java will get confused). The code for that would look like Calendar name = Calendar.getInstance(). This gets the current date and stores it in this Calendar object.
To access the information we want, we need to use the Calendars get method. For instance, if we wanted the month we would use name.get(Calendar.MONTH), or if we wanted the day we would use name.get(Calendar.DATE). Calendar.MONTH and Calendar.DATE are just ways to refer to the locations where those values are stored.
You also may make use of a number of String functions. The indexOf and substring methods could be of particular use. The indexOf method finds the index of the first occurrence of the given character in the String you call the function on. For example, if we had Hello store in a variable x, calling x.indexOf(l) would return a value of 2 since the first l is located at index 2 in the String Hello.
The substring method creates a new string from the starting index given to the ending index given (it does not include the character in the ending index). If not ending index is given, then a substring from the beginning index to the end of the String is created. If we wanted a substring of Hello that just captured ell and if Hello was stored in variable x, we could call x.substring(1, 4). If we wanted just llo, we could call x.substring(2).
Finally, you may find it useful to convert a number as a String into an integer value. In order to do this we can call Integer.parseInt(String), where String is the String value of the integer we want.
I strongly encourage you to tackle this assignments in parts and practice iterative design. Work on a few things and then test them to make sure they work. Then, add some more and test those. Make sure that you come up with a plan before you start coding. Writing pseudocode can also be very helpful. I encourage you to speak with your classmates about your development plan for this assignment. I am also available to discuss how you might approach this assignment. Feel free to reach out to me with any questions or concerns.
Style:
It is important that you get used to writing code in good style. What is demonstrated in examples in class is considered good style. Additionally, you should look at the style guide located on Cavas. Code written not in good style will lose points.
Extra Credit Opportunities (Optional):
You can choose to do as many or as few of these as you would like. Each one completed successfully and in good style will earn some extra credit points towards this assignment.
1. As stated above in the specification, you are allowed to let your calendar run to 35 days. For an added challenge, you can limit the number of days to 31. Completing this task successfully and in good style will result in extra credit.
what date would you like to look at? 9/20 8 10 12 13 14 l 15 16 17 18 19 2e 21 23 24 25 26 | 27 28 29 30 31 32 34 l 35 Month: 9 Day: 2eStep by Step Solution
There are 3 Steps involved in it
Step: 1
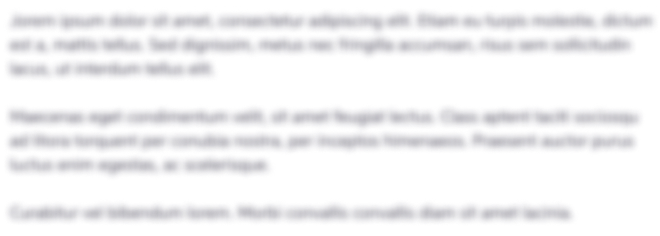
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started